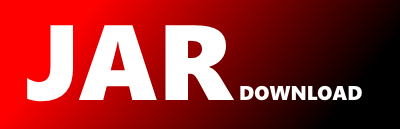
com.pulumi.azurenative.containerregistry.Task Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.containerregistry;
import com.pulumi.azurenative.Utilities;
import com.pulumi.azurenative.containerregistry.TaskArgs;
import com.pulumi.azurenative.containerregistry.outputs.AgentPropertiesResponse;
import com.pulumi.azurenative.containerregistry.outputs.CredentialsResponse;
import com.pulumi.azurenative.containerregistry.outputs.DockerBuildStepResponse;
import com.pulumi.azurenative.containerregistry.outputs.EncodedTaskStepResponse;
import com.pulumi.azurenative.containerregistry.outputs.FileTaskStepResponse;
import com.pulumi.azurenative.containerregistry.outputs.IdentityPropertiesResponse;
import com.pulumi.azurenative.containerregistry.outputs.PlatformPropertiesResponse;
import com.pulumi.azurenative.containerregistry.outputs.SystemDataResponse;
import com.pulumi.azurenative.containerregistry.outputs.TriggerPropertiesResponse;
import com.pulumi.core.Alias;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.Object;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* The task that has the ARM resource and task properties.
* The task will have all information to schedule a run against it.
* Azure REST API version: 2019-06-01-preview. Prior API version in Azure Native 1.x: 2019-06-01-preview.
*
* Other available API versions: 2018-09-01, 2019-04-01.
*
* ## Example Usage
* ### Tasks_Create
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.containerregistry.Task;
* import com.pulumi.azurenative.containerregistry.TaskArgs;
* import com.pulumi.azurenative.containerregistry.inputs.AgentPropertiesArgs;
* import com.pulumi.azurenative.containerregistry.inputs.IdentityPropertiesArgs;
* import com.pulumi.azurenative.containerregistry.inputs.PlatformPropertiesArgs;
* import com.pulumi.azurenative.containerregistry.inputs.TriggerPropertiesArgs;
* import com.pulumi.azurenative.containerregistry.inputs.BaseImageTriggerArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App }{{@code
* public static void main(String[] args) }{{@code
* Pulumi.run(App::stack);
* }}{@code
*
* public static void stack(Context ctx) }{{@code
* var task = new Task("task", TaskArgs.builder()
* .agentConfiguration(AgentPropertiesArgs.builder()
* .cpu(2)
* .build())
* .identity(IdentityPropertiesArgs.builder()
* .type("SystemAssigned")
* .build())
* .isSystemTask(false)
* .location("eastus")
* .logTemplate("acr/tasks:}{{{@code .Run.OS}}}{@code ")
* .platform(PlatformPropertiesArgs.builder()
* .architecture("amd64")
* .os("Linux")
* .build())
* .registryName("myRegistry")
* .resourceGroupName("myResourceGroup")
* .status("Enabled")
* .step(DockerBuildStepArgs.builder()
* .arguments(
* ArgumentArgs.builder()
* .isSecret(false)
* .name("mytestargument")
* .value("mytestvalue")
* .build(),
* ArgumentArgs.builder()
* .isSecret(true)
* .name("mysecrettestargument")
* .value("mysecrettestvalue")
* .build())
* .contextPath("src")
* .dockerFilePath("src/DockerFile")
* .imageNames("azurerest:testtag")
* .isPushEnabled(true)
* .noCache(false)
* .type("Docker")
* .build())
* .tags(Map.of("testkey", "value"))
* .taskName("mytTask")
* .trigger(TriggerPropertiesArgs.builder()
* .baseImageTrigger(BaseImageTriggerArgs.builder()
* .baseImageTriggerType("Runtime")
* .name("myBaseImageTrigger")
* .updateTriggerEndpoint("https://user:pass}{@literal @}{@code mycicd.webhook.com?token=foo")
* .updateTriggerPayloadType("Token")
* .build())
* .sourceTriggers(SourceTriggerArgs.builder()
* .name("mySourceTrigger")
* .sourceRepository(SourcePropertiesArgs.builder()
* .branch("master")
* .repositoryUrl("https://github.com/Azure/azure-rest-api-specs")
* .sourceControlAuthProperties(AuthInfoArgs.builder()
* .token("xxxxx")
* .tokenType("PAT")
* .build())
* .sourceControlType("Github")
* .build())
* .sourceTriggerEvents("commit")
* .build())
* .timerTriggers(TimerTriggerArgs.builder()
* .name("myTimerTrigger")
* .schedule("30 9 * * 1-5")
* .build())
* .build())
* .build());
*
* }}{@code
* }}{@code
*
* }
*
* ### Tasks_Create_QuickTask
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.containerregistry.Task;
* import com.pulumi.azurenative.containerregistry.TaskArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var task = new Task("task", TaskArgs.builder()
* .isSystemTask(true)
* .location("eastus")
* .logTemplate("acr/tasks:{{.Run.OS}}")
* .registryName("myRegistry")
* .resourceGroupName("myResourceGroup")
* .status("Enabled")
* .tags(Map.of("testkey", "value"))
* .taskName("quicktask")
* .build());
*
* }
* }
*
* }
*
* ### Tasks_Create_WithSystemAndUserIdentities
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.containerregistry.Task;
* import com.pulumi.azurenative.containerregistry.TaskArgs;
* import com.pulumi.azurenative.containerregistry.inputs.AgentPropertiesArgs;
* import com.pulumi.azurenative.containerregistry.inputs.IdentityPropertiesArgs;
* import com.pulumi.azurenative.containerregistry.inputs.PlatformPropertiesArgs;
* import com.pulumi.azurenative.containerregistry.inputs.TriggerPropertiesArgs;
* import com.pulumi.azurenative.containerregistry.inputs.BaseImageTriggerArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App }{{@code
* public static void main(String[] args) }{{@code
* Pulumi.run(App::stack);
* }}{@code
*
* public static void stack(Context ctx) }{{@code
* var task = new Task("task", TaskArgs.builder()
* .agentConfiguration(AgentPropertiesArgs.builder()
* .cpu(2)
* .build())
* .identity(IdentityPropertiesArgs.builder()
* .type("SystemAssigned, UserAssigned")
* .userAssignedIdentities(Map.of("/subscriptions/f9d7ebed-adbd-4cb4-b973-aaf82c136138/resourcegroups/myResourceGroup1/providers/Microsoft.ManagedIdentity/userAssignedIdentities/identity2", ))
* .build())
* .isSystemTask(false)
* .location("eastus")
* .platform(PlatformPropertiesArgs.builder()
* .architecture("amd64")
* .os("Linux")
* .build())
* .registryName("myRegistry")
* .resourceGroupName("myResourceGroup")
* .status("Enabled")
* .step(DockerBuildStepArgs.builder()
* .arguments(
* ArgumentArgs.builder()
* .isSecret(false)
* .name("mytestargument")
* .value("mytestvalue")
* .build(),
* ArgumentArgs.builder()
* .isSecret(true)
* .name("mysecrettestargument")
* .value("mysecrettestvalue")
* .build())
* .contextPath("src")
* .dockerFilePath("src/DockerFile")
* .imageNames("azurerest:testtag")
* .isPushEnabled(true)
* .noCache(false)
* .type("Docker")
* .build())
* .tags(Map.of("testkey", "value"))
* .taskName("mytTask")
* .trigger(TriggerPropertiesArgs.builder()
* .baseImageTrigger(BaseImageTriggerArgs.builder()
* .baseImageTriggerType("Runtime")
* .name("myBaseImageTrigger")
* .updateTriggerEndpoint("https://user:pass}{@literal @}{@code mycicd.webhook.com?token=foo")
* .updateTriggerPayloadType("Default")
* .build())
* .sourceTriggers(SourceTriggerArgs.builder()
* .name("mySourceTrigger")
* .sourceRepository(SourcePropertiesArgs.builder()
* .branch("master")
* .repositoryUrl("https://github.com/Azure/azure-rest-api-specs")
* .sourceControlAuthProperties(AuthInfoArgs.builder()
* .token("xxxxx")
* .tokenType("PAT")
* .build())
* .sourceControlType("Github")
* .build())
* .sourceTriggerEvents("commit")
* .build())
* .timerTriggers(TimerTriggerArgs.builder()
* .name("myTimerTrigger")
* .schedule("30 9 * * 1-5")
* .build())
* .build())
* .build());
*
* }}{@code
* }}{@code
*
* }
*
* ### Tasks_Create_WithUserIdentities
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.containerregistry.Task;
* import com.pulumi.azurenative.containerregistry.TaskArgs;
* import com.pulumi.azurenative.containerregistry.inputs.AgentPropertiesArgs;
* import com.pulumi.azurenative.containerregistry.inputs.IdentityPropertiesArgs;
* import com.pulumi.azurenative.containerregistry.inputs.PlatformPropertiesArgs;
* import com.pulumi.azurenative.containerregistry.inputs.TriggerPropertiesArgs;
* import com.pulumi.azurenative.containerregistry.inputs.BaseImageTriggerArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App }{{@code
* public static void main(String[] args) }{{@code
* Pulumi.run(App::stack);
* }}{@code
*
* public static void stack(Context ctx) }{{@code
* var task = new Task("task", TaskArgs.builder()
* .agentConfiguration(AgentPropertiesArgs.builder()
* .cpu(2)
* .build())
* .identity(IdentityPropertiesArgs.builder()
* .type("UserAssigned")
* .userAssignedIdentities(Map.ofEntries(
* Map.entry("/subscriptions/f9d7ebed-adbd-4cb4-b973-aaf82c136138/resourcegroups/myResourceGroup/providers/Microsoft.ManagedIdentity/userAssignedIdentities/identity1", ),
* Map.entry("/subscriptions/f9d7ebed-adbd-4cb4-b973-aaf82c136138/resourcegroups/myResourceGroup1/providers/Microsoft.ManagedIdentity/userAssignedIdentities/identity2", )
* ))
* .build())
* .isSystemTask(false)
* .location("eastus")
* .platform(PlatformPropertiesArgs.builder()
* .architecture("amd64")
* .os("Linux")
* .build())
* .registryName("myRegistry")
* .resourceGroupName("myResourceGroup")
* .status("Enabled")
* .step(DockerBuildStepArgs.builder()
* .arguments(
* ArgumentArgs.builder()
* .isSecret(false)
* .name("mytestargument")
* .value("mytestvalue")
* .build(),
* ArgumentArgs.builder()
* .isSecret(true)
* .name("mysecrettestargument")
* .value("mysecrettestvalue")
* .build())
* .contextPath("src")
* .dockerFilePath("src/DockerFile")
* .imageNames("azurerest:testtag")
* .isPushEnabled(true)
* .noCache(false)
* .type("Docker")
* .build())
* .tags(Map.of("testkey", "value"))
* .taskName("mytTask")
* .trigger(TriggerPropertiesArgs.builder()
* .baseImageTrigger(BaseImageTriggerArgs.builder()
* .baseImageTriggerType("Runtime")
* .name("myBaseImageTrigger")
* .updateTriggerEndpoint("https://user:pass}{@literal @}{@code mycicd.webhook.com?token=foo")
* .updateTriggerPayloadType("Default")
* .build())
* .sourceTriggers(SourceTriggerArgs.builder()
* .name("mySourceTrigger")
* .sourceRepository(SourcePropertiesArgs.builder()
* .branch("master")
* .repositoryUrl("https://github.com/Azure/azure-rest-api-specs")
* .sourceControlAuthProperties(AuthInfoArgs.builder()
* .token("xxxxx")
* .tokenType("PAT")
* .build())
* .sourceControlType("Github")
* .build())
* .sourceTriggerEvents("commit")
* .build())
* .timerTriggers(TimerTriggerArgs.builder()
* .name("myTimerTrigger")
* .schedule("30 9 * * 1-5")
* .build())
* .build())
* .build());
*
* }}{@code
* }}{@code
*
* }
*
* ### Tasks_Create_WithUserIdentities_WithSystemIdentity
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.containerregistry.Task;
* import com.pulumi.azurenative.containerregistry.TaskArgs;
* import com.pulumi.azurenative.containerregistry.inputs.AgentPropertiesArgs;
* import com.pulumi.azurenative.containerregistry.inputs.IdentityPropertiesArgs;
* import com.pulumi.azurenative.containerregistry.inputs.PlatformPropertiesArgs;
* import com.pulumi.azurenative.containerregistry.inputs.TriggerPropertiesArgs;
* import com.pulumi.azurenative.containerregistry.inputs.BaseImageTriggerArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var task = new Task("task", TaskArgs.builder()
* .agentConfiguration(AgentPropertiesArgs.builder()
* .cpu(2)
* .build())
* .identity(IdentityPropertiesArgs.builder()
* .type("SystemAssigned")
* .build())
* .isSystemTask(false)
* .location("eastus")
* .platform(PlatformPropertiesArgs.builder()
* .architecture("amd64")
* .os("Linux")
* .build())
* .registryName("myRegistry")
* .resourceGroupName("myResourceGroup")
* .status("Enabled")
* .step(DockerBuildStepArgs.builder()
* .arguments(
* ArgumentArgs.builder()
* .isSecret(false)
* .name("mytestargument")
* .value("mytestvalue")
* .build(),
* ArgumentArgs.builder()
* .isSecret(true)
* .name("mysecrettestargument")
* .value("mysecrettestvalue")
* .build())
* .contextPath("src")
* .dockerFilePath("src/DockerFile")
* .imageNames("azurerest:testtag")
* .isPushEnabled(true)
* .noCache(false)
* .type("Docker")
* .build())
* .tags(Map.of("testkey", "value"))
* .taskName("mytTask")
* .trigger(TriggerPropertiesArgs.builder()
* .baseImageTrigger(BaseImageTriggerArgs.builder()
* .baseImageTriggerType("Runtime")
* .name("myBaseImageTrigger")
* .build())
* .sourceTriggers(SourceTriggerArgs.builder()
* .name("mySourceTrigger")
* .sourceRepository(SourcePropertiesArgs.builder()
* .branch("master")
* .repositoryUrl("https://github.com/Azure/azure-rest-api-specs")
* .sourceControlAuthProperties(AuthInfoArgs.builder()
* .token("xxxxx")
* .tokenType("PAT")
* .build())
* .sourceControlType("Github")
* .build())
* .sourceTriggerEvents("commit")
* .build())
* .timerTriggers(TimerTriggerArgs.builder()
* .name("myTimerTrigger")
* .schedule("30 9 * * 1-5")
* .build())
* .build())
* .build());
*
* }
* }
*
* }
*
*
* ## Import
*
* An existing resource can be imported using its type token, name, and identifier, e.g.
*
* ```sh
* $ pulumi import azure-native:containerregistry:Task myTask /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.ContainerRegistry/registries/{registryName}/tasks/{taskName}
* ```
*
*/
@ResourceType(type="azure-native:containerregistry:Task")
public class Task extends com.pulumi.resources.CustomResource {
/**
* The machine configuration of the run agent.
*
*/
@Export(name="agentConfiguration", refs={AgentPropertiesResponse.class}, tree="[0]")
private Output* @Nullable */ AgentPropertiesResponse> agentConfiguration;
/**
* @return The machine configuration of the run agent.
*
*/
public Output> agentConfiguration() {
return Codegen.optional(this.agentConfiguration);
}
/**
* The dedicated agent pool for the task.
*
*/
@Export(name="agentPoolName", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> agentPoolName;
/**
* @return The dedicated agent pool for the task.
*
*/
public Output> agentPoolName() {
return Codegen.optional(this.agentPoolName);
}
/**
* The creation date of task.
*
*/
@Export(name="creationDate", refs={String.class}, tree="[0]")
private Output creationDate;
/**
* @return The creation date of task.
*
*/
public Output creationDate() {
return this.creationDate;
}
/**
* The properties that describes a set of credentials that will be used when this run is invoked.
*
*/
@Export(name="credentials", refs={CredentialsResponse.class}, tree="[0]")
private Output* @Nullable */ CredentialsResponse> credentials;
/**
* @return The properties that describes a set of credentials that will be used when this run is invoked.
*
*/
public Output> credentials() {
return Codegen.optional(this.credentials);
}
/**
* Identity for the resource.
*
*/
@Export(name="identity", refs={IdentityPropertiesResponse.class}, tree="[0]")
private Output* @Nullable */ IdentityPropertiesResponse> identity;
/**
* @return Identity for the resource.
*
*/
public Output> identity() {
return Codegen.optional(this.identity);
}
/**
* The value of this property indicates whether the task resource is system task or not.
*
*/
@Export(name="isSystemTask", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> isSystemTask;
/**
* @return The value of this property indicates whether the task resource is system task or not.
*
*/
public Output> isSystemTask() {
return Codegen.optional(this.isSystemTask);
}
/**
* The location of the resource. This cannot be changed after the resource is created.
*
*/
@Export(name="location", refs={String.class}, tree="[0]")
private Output location;
/**
* @return The location of the resource. This cannot be changed after the resource is created.
*
*/
public Output location() {
return this.location;
}
/**
* The template that describes the repository and tag information for run log artifact.
*
*/
@Export(name="logTemplate", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> logTemplate;
/**
* @return The template that describes the repository and tag information for run log artifact.
*
*/
public Output> logTemplate() {
return Codegen.optional(this.logTemplate);
}
/**
* The name of the resource.
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return The name of the resource.
*
*/
public Output name() {
return this.name;
}
/**
* The platform properties against which the run has to happen.
*
*/
@Export(name="platform", refs={PlatformPropertiesResponse.class}, tree="[0]")
private Output* @Nullable */ PlatformPropertiesResponse> platform;
/**
* @return The platform properties against which the run has to happen.
*
*/
public Output> platform() {
return Codegen.optional(this.platform);
}
/**
* The provisioning state of the task.
*
*/
@Export(name="provisioningState", refs={String.class}, tree="[0]")
private Output provisioningState;
/**
* @return The provisioning state of the task.
*
*/
public Output provisioningState() {
return this.provisioningState;
}
/**
* The current status of task.
*
*/
@Export(name="status", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> status;
/**
* @return The current status of task.
*
*/
public Output> status() {
return Codegen.optional(this.status);
}
/**
* The properties of a task step.
*
*/
@Export(name="step", refs={Object.class}, tree="[0]")
private Output* @Nullable */ Object> step;
/**
* @return The properties of a task step.
*
*/
public Output> step() {
return Codegen.optional(this.step);
}
/**
* Metadata pertaining to creation and last modification of the resource.
*
*/
@Export(name="systemData", refs={SystemDataResponse.class}, tree="[0]")
private Output systemData;
/**
* @return Metadata pertaining to creation and last modification of the resource.
*
*/
public Output systemData() {
return this.systemData;
}
/**
* The tags of the resource.
*
*/
@Export(name="tags", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> tags;
/**
* @return The tags of the resource.
*
*/
public Output>> tags() {
return Codegen.optional(this.tags);
}
/**
* Run timeout in seconds.
*
*/
@Export(name="timeout", refs={Integer.class}, tree="[0]")
private Output* @Nullable */ Integer> timeout;
/**
* @return Run timeout in seconds.
*
*/
public Output> timeout() {
return Codegen.optional(this.timeout);
}
/**
* The properties that describe all triggers for the task.
*
*/
@Export(name="trigger", refs={TriggerPropertiesResponse.class}, tree="[0]")
private Output* @Nullable */ TriggerPropertiesResponse> trigger;
/**
* @return The properties that describe all triggers for the task.
*
*/
public Output> trigger() {
return Codegen.optional(this.trigger);
}
/**
* The type of the resource.
*
*/
@Export(name="type", refs={String.class}, tree="[0]")
private Output type;
/**
* @return The type of the resource.
*
*/
public Output type() {
return this.type;
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public Task(java.lang.String name) {
this(name, TaskArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public Task(java.lang.String name, TaskArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public Task(java.lang.String name, TaskArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:containerregistry:Task", name, makeArgs(args, options), makeResourceOptions(options, Codegen.empty()), false);
}
private Task(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:containerregistry:Task", name, null, makeResourceOptions(options, id), false);
}
private static TaskArgs makeArgs(TaskArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
if (options != null && options.getUrn().isPresent()) {
return null;
}
return args == null ? TaskArgs.Empty : args;
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.aliases(List.of(
Output.of(Alias.builder().type("azure-native:containerregistry/v20180901:Task").build()),
Output.of(Alias.builder().type("azure-native:containerregistry/v20190401:Task").build()),
Output.of(Alias.builder().type("azure-native:containerregistry/v20190601preview:Task").build())
))
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static Task get(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new Task(name, id, options);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy