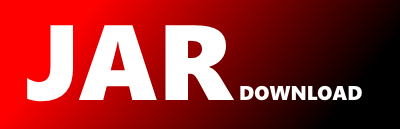
com.pulumi.azurenative.customerinsights.PredictionArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.customerinsights;
import com.pulumi.azurenative.customerinsights.inputs.PredictionGradesArgs;
import com.pulumi.azurenative.customerinsights.inputs.PredictionMappingsArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class PredictionArgs extends com.pulumi.resources.ResourceArgs {
public static final PredictionArgs Empty = new PredictionArgs();
/**
* Whether do auto analyze.
*
*/
@Import(name="autoAnalyze", required=true)
private Output autoAnalyze;
/**
* @return Whether do auto analyze.
*
*/
public Output autoAnalyze() {
return this.autoAnalyze;
}
/**
* Description of the prediction.
*
*/
@Import(name="description")
private @Nullable Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy