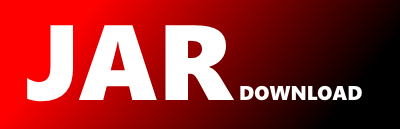
com.pulumi.azurenative.databasewatcher.SharedPrivateLinkResourceArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.databasewatcher;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class SharedPrivateLinkResourceArgs extends com.pulumi.resources.ResourceArgs {
public static final SharedPrivateLinkResourceArgs Empty = new SharedPrivateLinkResourceArgs();
/**
* The DNS zone to be included in the DNS name of the shared private link. Value is required for Azure Data Explorer clusters and SQL managed instances. The value to use is the second segment of the host FQDN name of the resource that the shared private link resource is for.
*
*/
@Import(name="dnsZone")
private @Nullable Output dnsZone;
/**
* @return The DNS zone to be included in the DNS name of the shared private link. Value is required for Azure Data Explorer clusters and SQL managed instances. The value to use is the second segment of the host FQDN name of the resource that the shared private link resource is for.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy