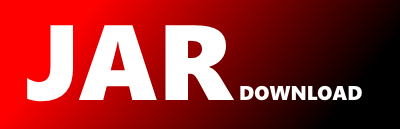
com.pulumi.azurenative.databoxedge.outputs.AddressResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.databoxedge.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class AddressResponse {
/**
* @return The address line1.
*
*/
private @Nullable String addressLine1;
/**
* @return The address line2.
*
*/
private @Nullable String addressLine2;
/**
* @return The address line3.
*
*/
private @Nullable String addressLine3;
/**
* @return The city name.
*
*/
private @Nullable String city;
/**
* @return The country name.
*
*/
private String country;
/**
* @return The postal code.
*
*/
private @Nullable String postalCode;
/**
* @return The state name.
*
*/
private @Nullable String state;
private AddressResponse() {}
/**
* @return The address line1.
*
*/
public Optional addressLine1() {
return Optional.ofNullable(this.addressLine1);
}
/**
* @return The address line2.
*
*/
public Optional addressLine2() {
return Optional.ofNullable(this.addressLine2);
}
/**
* @return The address line3.
*
*/
public Optional addressLine3() {
return Optional.ofNullable(this.addressLine3);
}
/**
* @return The city name.
*
*/
public Optional city() {
return Optional.ofNullable(this.city);
}
/**
* @return The country name.
*
*/
public String country() {
return this.country;
}
/**
* @return The postal code.
*
*/
public Optional postalCode() {
return Optional.ofNullable(this.postalCode);
}
/**
* @return The state name.
*
*/
public Optional state() {
return Optional.ofNullable(this.state);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(AddressResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String addressLine1;
private @Nullable String addressLine2;
private @Nullable String addressLine3;
private @Nullable String city;
private String country;
private @Nullable String postalCode;
private @Nullable String state;
public Builder() {}
public Builder(AddressResponse defaults) {
Objects.requireNonNull(defaults);
this.addressLine1 = defaults.addressLine1;
this.addressLine2 = defaults.addressLine2;
this.addressLine3 = defaults.addressLine3;
this.city = defaults.city;
this.country = defaults.country;
this.postalCode = defaults.postalCode;
this.state = defaults.state;
}
@CustomType.Setter
public Builder addressLine1(@Nullable String addressLine1) {
this.addressLine1 = addressLine1;
return this;
}
@CustomType.Setter
public Builder addressLine2(@Nullable String addressLine2) {
this.addressLine2 = addressLine2;
return this;
}
@CustomType.Setter
public Builder addressLine3(@Nullable String addressLine3) {
this.addressLine3 = addressLine3;
return this;
}
@CustomType.Setter
public Builder city(@Nullable String city) {
this.city = city;
return this;
}
@CustomType.Setter
public Builder country(String country) {
if (country == null) {
throw new MissingRequiredPropertyException("AddressResponse", "country");
}
this.country = country;
return this;
}
@CustomType.Setter
public Builder postalCode(@Nullable String postalCode) {
this.postalCode = postalCode;
return this;
}
@CustomType.Setter
public Builder state(@Nullable String state) {
this.state = state;
return this;
}
public AddressResponse build() {
final var _resultValue = new AddressResponse();
_resultValue.addressLine1 = addressLine1;
_resultValue.addressLine2 = addressLine2;
_resultValue.addressLine3 = addressLine3;
_resultValue.city = city;
_resultValue.country = country;
_resultValue.postalCode = postalCode;
_resultValue.state = state;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy