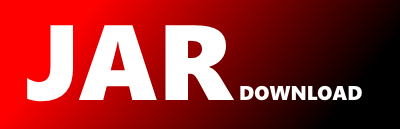
com.pulumi.azurenative.datamigration.inputs.MigrateSqlServerSqlMITaskInputArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.datamigration.inputs;
import com.pulumi.azurenative.datamigration.enums.BackupMode;
import com.pulumi.azurenative.datamigration.inputs.BlobShareArgs;
import com.pulumi.azurenative.datamigration.inputs.FileShareArgs;
import com.pulumi.azurenative.datamigration.inputs.MigrateSqlServerSqlMIDatabaseInputArgs;
import com.pulumi.azurenative.datamigration.inputs.SqlConnectionInfoArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Input for task that migrates SQL Server databases to Azure SQL Database Managed Instance.
*
*/
public final class MigrateSqlServerSqlMITaskInputArgs extends com.pulumi.resources.ResourceArgs {
public static final MigrateSqlServerSqlMITaskInputArgs Empty = new MigrateSqlServerSqlMITaskInputArgs();
/**
* Azure Active Directory domain name in the format of 'contoso.com' for federated Azure AD or 'contoso.onmicrosoft.com' for managed domain, required if and only if Windows logins are selected
*
*/
@Import(name="aadDomainName")
private @Nullable Output aadDomainName;
/**
* @return Azure Active Directory domain name in the format of 'contoso.com' for federated Azure AD or 'contoso.onmicrosoft.com' for managed domain, required if and only if Windows logins are selected
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy