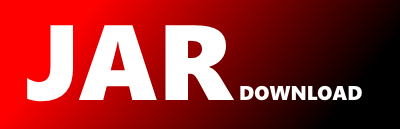
com.pulumi.azurenative.datamigration.outputs.GetDatabaseMigrationsMongoToCosmosDbRUMongoResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.datamigration.outputs;
import com.pulumi.azurenative.datamigration.outputs.ErrorInfoResponse;
import com.pulumi.azurenative.datamigration.outputs.MongoConnectionInformationResponse;
import com.pulumi.azurenative.datamigration.outputs.MongoMigrationCollectionResponse;
import com.pulumi.azurenative.datamigration.outputs.SystemDataResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetDatabaseMigrationsMongoToCosmosDbRUMongoResult {
/**
* @return List of Mongo Collections to be migrated.
*
*/
private @Nullable List collectionList;
/**
* @return Database migration end time.
*
*/
private String endedOn;
/**
* @return Fully qualified resource ID for the resource. E.g. "/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}"
*
*/
private String id;
/**
* @return
* Expected value is 'MongoToCosmosDbMongo'.
*
*/
private String kind;
/**
* @return Error details in case of migration failure.
*
*/
private ErrorInfoResponse migrationFailureError;
/**
* @return ID for current migration operation.
*
*/
private @Nullable String migrationOperationId;
/**
* @return Resource Id of the Migration Service.
*
*/
private @Nullable String migrationService;
/**
* @return Migration status.
*
*/
private String migrationStatus;
/**
* @return The name of the resource
*
*/
private String name;
/**
* @return Error message for migration provisioning failure, if any.
*
*/
private @Nullable String provisioningError;
/**
* @return Provisioning State of migration. ProvisioningState as Succeeded implies that validations have been performed and migration has started.
*
*/
private String provisioningState;
/**
* @return Resource Id of the target resource.
*
*/
private @Nullable String scope;
/**
* @return Source Mongo connection details.
*
*/
private @Nullable MongoConnectionInformationResponse sourceMongoConnection;
/**
* @return Database migration start time.
*
*/
private String startedOn;
/**
* @return Azure Resource Manager metadata containing createdBy and modifiedBy information.
*
*/
private SystemDataResponse systemData;
/**
* @return Target Cosmos DB Mongo connection details.
*
*/
private @Nullable MongoConnectionInformationResponse targetMongoConnection;
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
private String type;
private GetDatabaseMigrationsMongoToCosmosDbRUMongoResult() {}
/**
* @return List of Mongo Collections to be migrated.
*
*/
public List collectionList() {
return this.collectionList == null ? List.of() : this.collectionList;
}
/**
* @return Database migration end time.
*
*/
public String endedOn() {
return this.endedOn;
}
/**
* @return Fully qualified resource ID for the resource. E.g. "/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}"
*
*/
public String id() {
return this.id;
}
/**
* @return
* Expected value is 'MongoToCosmosDbMongo'.
*
*/
public String kind() {
return this.kind;
}
/**
* @return Error details in case of migration failure.
*
*/
public ErrorInfoResponse migrationFailureError() {
return this.migrationFailureError;
}
/**
* @return ID for current migration operation.
*
*/
public Optional migrationOperationId() {
return Optional.ofNullable(this.migrationOperationId);
}
/**
* @return Resource Id of the Migration Service.
*
*/
public Optional migrationService() {
return Optional.ofNullable(this.migrationService);
}
/**
* @return Migration status.
*
*/
public String migrationStatus() {
return this.migrationStatus;
}
/**
* @return The name of the resource
*
*/
public String name() {
return this.name;
}
/**
* @return Error message for migration provisioning failure, if any.
*
*/
public Optional provisioningError() {
return Optional.ofNullable(this.provisioningError);
}
/**
* @return Provisioning State of migration. ProvisioningState as Succeeded implies that validations have been performed and migration has started.
*
*/
public String provisioningState() {
return this.provisioningState;
}
/**
* @return Resource Id of the target resource.
*
*/
public Optional scope() {
return Optional.ofNullable(this.scope);
}
/**
* @return Source Mongo connection details.
*
*/
public Optional sourceMongoConnection() {
return Optional.ofNullable(this.sourceMongoConnection);
}
/**
* @return Database migration start time.
*
*/
public String startedOn() {
return this.startedOn;
}
/**
* @return Azure Resource Manager metadata containing createdBy and modifiedBy information.
*
*/
public SystemDataResponse systemData() {
return this.systemData;
}
/**
* @return Target Cosmos DB Mongo connection details.
*
*/
public Optional targetMongoConnection() {
return Optional.ofNullable(this.targetMongoConnection);
}
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
public String type() {
return this.type;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetDatabaseMigrationsMongoToCosmosDbRUMongoResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable List collectionList;
private String endedOn;
private String id;
private String kind;
private ErrorInfoResponse migrationFailureError;
private @Nullable String migrationOperationId;
private @Nullable String migrationService;
private String migrationStatus;
private String name;
private @Nullable String provisioningError;
private String provisioningState;
private @Nullable String scope;
private @Nullable MongoConnectionInformationResponse sourceMongoConnection;
private String startedOn;
private SystemDataResponse systemData;
private @Nullable MongoConnectionInformationResponse targetMongoConnection;
private String type;
public Builder() {}
public Builder(GetDatabaseMigrationsMongoToCosmosDbRUMongoResult defaults) {
Objects.requireNonNull(defaults);
this.collectionList = defaults.collectionList;
this.endedOn = defaults.endedOn;
this.id = defaults.id;
this.kind = defaults.kind;
this.migrationFailureError = defaults.migrationFailureError;
this.migrationOperationId = defaults.migrationOperationId;
this.migrationService = defaults.migrationService;
this.migrationStatus = defaults.migrationStatus;
this.name = defaults.name;
this.provisioningError = defaults.provisioningError;
this.provisioningState = defaults.provisioningState;
this.scope = defaults.scope;
this.sourceMongoConnection = defaults.sourceMongoConnection;
this.startedOn = defaults.startedOn;
this.systemData = defaults.systemData;
this.targetMongoConnection = defaults.targetMongoConnection;
this.type = defaults.type;
}
@CustomType.Setter
public Builder collectionList(@Nullable List collectionList) {
this.collectionList = collectionList;
return this;
}
public Builder collectionList(MongoMigrationCollectionResponse... collectionList) {
return collectionList(List.of(collectionList));
}
@CustomType.Setter
public Builder endedOn(String endedOn) {
if (endedOn == null) {
throw new MissingRequiredPropertyException("GetDatabaseMigrationsMongoToCosmosDbRUMongoResult", "endedOn");
}
this.endedOn = endedOn;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetDatabaseMigrationsMongoToCosmosDbRUMongoResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder kind(String kind) {
if (kind == null) {
throw new MissingRequiredPropertyException("GetDatabaseMigrationsMongoToCosmosDbRUMongoResult", "kind");
}
this.kind = kind;
return this;
}
@CustomType.Setter
public Builder migrationFailureError(ErrorInfoResponse migrationFailureError) {
if (migrationFailureError == null) {
throw new MissingRequiredPropertyException("GetDatabaseMigrationsMongoToCosmosDbRUMongoResult", "migrationFailureError");
}
this.migrationFailureError = migrationFailureError;
return this;
}
@CustomType.Setter
public Builder migrationOperationId(@Nullable String migrationOperationId) {
this.migrationOperationId = migrationOperationId;
return this;
}
@CustomType.Setter
public Builder migrationService(@Nullable String migrationService) {
this.migrationService = migrationService;
return this;
}
@CustomType.Setter
public Builder migrationStatus(String migrationStatus) {
if (migrationStatus == null) {
throw new MissingRequiredPropertyException("GetDatabaseMigrationsMongoToCosmosDbRUMongoResult", "migrationStatus");
}
this.migrationStatus = migrationStatus;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetDatabaseMigrationsMongoToCosmosDbRUMongoResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder provisioningError(@Nullable String provisioningError) {
this.provisioningError = provisioningError;
return this;
}
@CustomType.Setter
public Builder provisioningState(String provisioningState) {
if (provisioningState == null) {
throw new MissingRequiredPropertyException("GetDatabaseMigrationsMongoToCosmosDbRUMongoResult", "provisioningState");
}
this.provisioningState = provisioningState;
return this;
}
@CustomType.Setter
public Builder scope(@Nullable String scope) {
this.scope = scope;
return this;
}
@CustomType.Setter
public Builder sourceMongoConnection(@Nullable MongoConnectionInformationResponse sourceMongoConnection) {
this.sourceMongoConnection = sourceMongoConnection;
return this;
}
@CustomType.Setter
public Builder startedOn(String startedOn) {
if (startedOn == null) {
throw new MissingRequiredPropertyException("GetDatabaseMigrationsMongoToCosmosDbRUMongoResult", "startedOn");
}
this.startedOn = startedOn;
return this;
}
@CustomType.Setter
public Builder systemData(SystemDataResponse systemData) {
if (systemData == null) {
throw new MissingRequiredPropertyException("GetDatabaseMigrationsMongoToCosmosDbRUMongoResult", "systemData");
}
this.systemData = systemData;
return this;
}
@CustomType.Setter
public Builder targetMongoConnection(@Nullable MongoConnectionInformationResponse targetMongoConnection) {
this.targetMongoConnection = targetMongoConnection;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetDatabaseMigrationsMongoToCosmosDbRUMongoResult", "type");
}
this.type = type;
return this;
}
public GetDatabaseMigrationsMongoToCosmosDbRUMongoResult build() {
final var _resultValue = new GetDatabaseMigrationsMongoToCosmosDbRUMongoResult();
_resultValue.collectionList = collectionList;
_resultValue.endedOn = endedOn;
_resultValue.id = id;
_resultValue.kind = kind;
_resultValue.migrationFailureError = migrationFailureError;
_resultValue.migrationOperationId = migrationOperationId;
_resultValue.migrationService = migrationService;
_resultValue.migrationStatus = migrationStatus;
_resultValue.name = name;
_resultValue.provisioningError = provisioningError;
_resultValue.provisioningState = provisioningState;
_resultValue.scope = scope;
_resultValue.sourceMongoConnection = sourceMongoConnection;
_resultValue.startedOn = startedOn;
_resultValue.systemData = systemData;
_resultValue.targetMongoConnection = targetMongoConnection;
_resultValue.type = type;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy