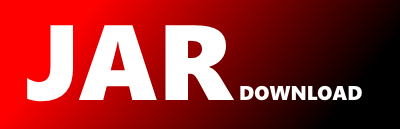
com.pulumi.azurenative.datamigration.outputs.MigrateSqlServerSqlDbTaskOutputTableLevelResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.datamigration.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Double;
import java.lang.String;
import java.util.Objects;
@CustomType
public final class MigrateSqlServerSqlDbTaskOutputTableLevelResponse {
/**
* @return Migration end time
*
*/
private String endedOn;
/**
* @return Wildcard string prefix to use for querying all errors of the item
*
*/
private String errorPrefix;
/**
* @return Result identifier
*
*/
private String id;
/**
* @return Number of successfully completed items
*
*/
private Double itemsCompletedCount;
/**
* @return Number of items
*
*/
private Double itemsCount;
/**
* @return Name of the item
*
*/
private String objectName;
/**
* @return Wildcard string prefix to use for querying all sub-tem results of the item
*
*/
private String resultPrefix;
/**
* @return Result type
* Expected value is 'TableLevelOutput'.
*
*/
private String resultType;
/**
* @return Migration start time
*
*/
private String startedOn;
/**
* @return Current state of migration
*
*/
private String state;
/**
* @return Status message
*
*/
private String statusMessage;
private MigrateSqlServerSqlDbTaskOutputTableLevelResponse() {}
/**
* @return Migration end time
*
*/
public String endedOn() {
return this.endedOn;
}
/**
* @return Wildcard string prefix to use for querying all errors of the item
*
*/
public String errorPrefix() {
return this.errorPrefix;
}
/**
* @return Result identifier
*
*/
public String id() {
return this.id;
}
/**
* @return Number of successfully completed items
*
*/
public Double itemsCompletedCount() {
return this.itemsCompletedCount;
}
/**
* @return Number of items
*
*/
public Double itemsCount() {
return this.itemsCount;
}
/**
* @return Name of the item
*
*/
public String objectName() {
return this.objectName;
}
/**
* @return Wildcard string prefix to use for querying all sub-tem results of the item
*
*/
public String resultPrefix() {
return this.resultPrefix;
}
/**
* @return Result type
* Expected value is 'TableLevelOutput'.
*
*/
public String resultType() {
return this.resultType;
}
/**
* @return Migration start time
*
*/
public String startedOn() {
return this.startedOn;
}
/**
* @return Current state of migration
*
*/
public String state() {
return this.state;
}
/**
* @return Status message
*
*/
public String statusMessage() {
return this.statusMessage;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(MigrateSqlServerSqlDbTaskOutputTableLevelResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String endedOn;
private String errorPrefix;
private String id;
private Double itemsCompletedCount;
private Double itemsCount;
private String objectName;
private String resultPrefix;
private String resultType;
private String startedOn;
private String state;
private String statusMessage;
public Builder() {}
public Builder(MigrateSqlServerSqlDbTaskOutputTableLevelResponse defaults) {
Objects.requireNonNull(defaults);
this.endedOn = defaults.endedOn;
this.errorPrefix = defaults.errorPrefix;
this.id = defaults.id;
this.itemsCompletedCount = defaults.itemsCompletedCount;
this.itemsCount = defaults.itemsCount;
this.objectName = defaults.objectName;
this.resultPrefix = defaults.resultPrefix;
this.resultType = defaults.resultType;
this.startedOn = defaults.startedOn;
this.state = defaults.state;
this.statusMessage = defaults.statusMessage;
}
@CustomType.Setter
public Builder endedOn(String endedOn) {
if (endedOn == null) {
throw new MissingRequiredPropertyException("MigrateSqlServerSqlDbTaskOutputTableLevelResponse", "endedOn");
}
this.endedOn = endedOn;
return this;
}
@CustomType.Setter
public Builder errorPrefix(String errorPrefix) {
if (errorPrefix == null) {
throw new MissingRequiredPropertyException("MigrateSqlServerSqlDbTaskOutputTableLevelResponse", "errorPrefix");
}
this.errorPrefix = errorPrefix;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("MigrateSqlServerSqlDbTaskOutputTableLevelResponse", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder itemsCompletedCount(Double itemsCompletedCount) {
if (itemsCompletedCount == null) {
throw new MissingRequiredPropertyException("MigrateSqlServerSqlDbTaskOutputTableLevelResponse", "itemsCompletedCount");
}
this.itemsCompletedCount = itemsCompletedCount;
return this;
}
@CustomType.Setter
public Builder itemsCount(Double itemsCount) {
if (itemsCount == null) {
throw new MissingRequiredPropertyException("MigrateSqlServerSqlDbTaskOutputTableLevelResponse", "itemsCount");
}
this.itemsCount = itemsCount;
return this;
}
@CustomType.Setter
public Builder objectName(String objectName) {
if (objectName == null) {
throw new MissingRequiredPropertyException("MigrateSqlServerSqlDbTaskOutputTableLevelResponse", "objectName");
}
this.objectName = objectName;
return this;
}
@CustomType.Setter
public Builder resultPrefix(String resultPrefix) {
if (resultPrefix == null) {
throw new MissingRequiredPropertyException("MigrateSqlServerSqlDbTaskOutputTableLevelResponse", "resultPrefix");
}
this.resultPrefix = resultPrefix;
return this;
}
@CustomType.Setter
public Builder resultType(String resultType) {
if (resultType == null) {
throw new MissingRequiredPropertyException("MigrateSqlServerSqlDbTaskOutputTableLevelResponse", "resultType");
}
this.resultType = resultType;
return this;
}
@CustomType.Setter
public Builder startedOn(String startedOn) {
if (startedOn == null) {
throw new MissingRequiredPropertyException("MigrateSqlServerSqlDbTaskOutputTableLevelResponse", "startedOn");
}
this.startedOn = startedOn;
return this;
}
@CustomType.Setter
public Builder state(String state) {
if (state == null) {
throw new MissingRequiredPropertyException("MigrateSqlServerSqlDbTaskOutputTableLevelResponse", "state");
}
this.state = state;
return this;
}
@CustomType.Setter
public Builder statusMessage(String statusMessage) {
if (statusMessage == null) {
throw new MissingRequiredPropertyException("MigrateSqlServerSqlDbTaskOutputTableLevelResponse", "statusMessage");
}
this.statusMessage = statusMessage;
return this;
}
public MigrateSqlServerSqlDbTaskOutputTableLevelResponse build() {
final var _resultValue = new MigrateSqlServerSqlDbTaskOutputTableLevelResponse();
_resultValue.endedOn = endedOn;
_resultValue.errorPrefix = errorPrefix;
_resultValue.id = id;
_resultValue.itemsCompletedCount = itemsCompletedCount;
_resultValue.itemsCount = itemsCount;
_resultValue.objectName = objectName;
_resultValue.resultPrefix = resultPrefix;
_resultValue.resultType = resultType;
_resultValue.startedOn = startedOn;
_resultValue.state = state;
_resultValue.statusMessage = statusMessage;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy