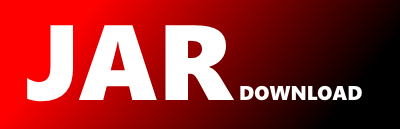
com.pulumi.azurenative.dataprotection.outputs.BackupInstanceResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.dataprotection.outputs;
import com.pulumi.azurenative.dataprotection.outputs.DatasourceResponse;
import com.pulumi.azurenative.dataprotection.outputs.DatasourceSetResponse;
import com.pulumi.azurenative.dataprotection.outputs.PolicyInfoResponse;
import com.pulumi.azurenative.dataprotection.outputs.ProtectionStatusDetailsResponse;
import com.pulumi.azurenative.dataprotection.outputs.SecretStoreBasedAuthCredentialsResponse;
import com.pulumi.azurenative.dataprotection.outputs.UserFacingErrorResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class BackupInstanceResponse {
/**
* @return Specifies the current protection state of the resource
*
*/
private String currentProtectionState;
/**
* @return Gets or sets the data source information.
*
*/
private DatasourceResponse dataSourceInfo;
/**
* @return Gets or sets the data source set information.
*
*/
private @Nullable DatasourceSetResponse dataSourceSetInfo;
/**
* @return Credentials to use to authenticate with data source provider.
*
*/
private @Nullable SecretStoreBasedAuthCredentialsResponse datasourceAuthCredentials;
/**
* @return Gets or sets the Backup Instance friendly name.
*
*/
private @Nullable String friendlyName;
private String objectType;
/**
* @return Gets or sets the policy information.
*
*/
private PolicyInfoResponse policyInfo;
/**
* @return Specifies the protection error of the resource
*
*/
private UserFacingErrorResponse protectionErrorDetails;
/**
* @return Specifies the protection status of the resource
*
*/
private ProtectionStatusDetailsResponse protectionStatus;
/**
* @return Specifies the provisioning state of the resource i.e. provisioning/updating/Succeeded/Failed
*
*/
private String provisioningState;
/**
* @return Specifies the type of validation. In case of DeepValidation, all validations from /validateForBackup API will run again.
*
*/
private @Nullable String validationType;
private BackupInstanceResponse() {}
/**
* @return Specifies the current protection state of the resource
*
*/
public String currentProtectionState() {
return this.currentProtectionState;
}
/**
* @return Gets or sets the data source information.
*
*/
public DatasourceResponse dataSourceInfo() {
return this.dataSourceInfo;
}
/**
* @return Gets or sets the data source set information.
*
*/
public Optional dataSourceSetInfo() {
return Optional.ofNullable(this.dataSourceSetInfo);
}
/**
* @return Credentials to use to authenticate with data source provider.
*
*/
public Optional datasourceAuthCredentials() {
return Optional.ofNullable(this.datasourceAuthCredentials);
}
/**
* @return Gets or sets the Backup Instance friendly name.
*
*/
public Optional friendlyName() {
return Optional.ofNullable(this.friendlyName);
}
public String objectType() {
return this.objectType;
}
/**
* @return Gets or sets the policy information.
*
*/
public PolicyInfoResponse policyInfo() {
return this.policyInfo;
}
/**
* @return Specifies the protection error of the resource
*
*/
public UserFacingErrorResponse protectionErrorDetails() {
return this.protectionErrorDetails;
}
/**
* @return Specifies the protection status of the resource
*
*/
public ProtectionStatusDetailsResponse protectionStatus() {
return this.protectionStatus;
}
/**
* @return Specifies the provisioning state of the resource i.e. provisioning/updating/Succeeded/Failed
*
*/
public String provisioningState() {
return this.provisioningState;
}
/**
* @return Specifies the type of validation. In case of DeepValidation, all validations from /validateForBackup API will run again.
*
*/
public Optional validationType() {
return Optional.ofNullable(this.validationType);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(BackupInstanceResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String currentProtectionState;
private DatasourceResponse dataSourceInfo;
private @Nullable DatasourceSetResponse dataSourceSetInfo;
private @Nullable SecretStoreBasedAuthCredentialsResponse datasourceAuthCredentials;
private @Nullable String friendlyName;
private String objectType;
private PolicyInfoResponse policyInfo;
private UserFacingErrorResponse protectionErrorDetails;
private ProtectionStatusDetailsResponse protectionStatus;
private String provisioningState;
private @Nullable String validationType;
public Builder() {}
public Builder(BackupInstanceResponse defaults) {
Objects.requireNonNull(defaults);
this.currentProtectionState = defaults.currentProtectionState;
this.dataSourceInfo = defaults.dataSourceInfo;
this.dataSourceSetInfo = defaults.dataSourceSetInfo;
this.datasourceAuthCredentials = defaults.datasourceAuthCredentials;
this.friendlyName = defaults.friendlyName;
this.objectType = defaults.objectType;
this.policyInfo = defaults.policyInfo;
this.protectionErrorDetails = defaults.protectionErrorDetails;
this.protectionStatus = defaults.protectionStatus;
this.provisioningState = defaults.provisioningState;
this.validationType = defaults.validationType;
}
@CustomType.Setter
public Builder currentProtectionState(String currentProtectionState) {
if (currentProtectionState == null) {
throw new MissingRequiredPropertyException("BackupInstanceResponse", "currentProtectionState");
}
this.currentProtectionState = currentProtectionState;
return this;
}
@CustomType.Setter
public Builder dataSourceInfo(DatasourceResponse dataSourceInfo) {
if (dataSourceInfo == null) {
throw new MissingRequiredPropertyException("BackupInstanceResponse", "dataSourceInfo");
}
this.dataSourceInfo = dataSourceInfo;
return this;
}
@CustomType.Setter
public Builder dataSourceSetInfo(@Nullable DatasourceSetResponse dataSourceSetInfo) {
this.dataSourceSetInfo = dataSourceSetInfo;
return this;
}
@CustomType.Setter
public Builder datasourceAuthCredentials(@Nullable SecretStoreBasedAuthCredentialsResponse datasourceAuthCredentials) {
this.datasourceAuthCredentials = datasourceAuthCredentials;
return this;
}
@CustomType.Setter
public Builder friendlyName(@Nullable String friendlyName) {
this.friendlyName = friendlyName;
return this;
}
@CustomType.Setter
public Builder objectType(String objectType) {
if (objectType == null) {
throw new MissingRequiredPropertyException("BackupInstanceResponse", "objectType");
}
this.objectType = objectType;
return this;
}
@CustomType.Setter
public Builder policyInfo(PolicyInfoResponse policyInfo) {
if (policyInfo == null) {
throw new MissingRequiredPropertyException("BackupInstanceResponse", "policyInfo");
}
this.policyInfo = policyInfo;
return this;
}
@CustomType.Setter
public Builder protectionErrorDetails(UserFacingErrorResponse protectionErrorDetails) {
if (protectionErrorDetails == null) {
throw new MissingRequiredPropertyException("BackupInstanceResponse", "protectionErrorDetails");
}
this.protectionErrorDetails = protectionErrorDetails;
return this;
}
@CustomType.Setter
public Builder protectionStatus(ProtectionStatusDetailsResponse protectionStatus) {
if (protectionStatus == null) {
throw new MissingRequiredPropertyException("BackupInstanceResponse", "protectionStatus");
}
this.protectionStatus = protectionStatus;
return this;
}
@CustomType.Setter
public Builder provisioningState(String provisioningState) {
if (provisioningState == null) {
throw new MissingRequiredPropertyException("BackupInstanceResponse", "provisioningState");
}
this.provisioningState = provisioningState;
return this;
}
@CustomType.Setter
public Builder validationType(@Nullable String validationType) {
this.validationType = validationType;
return this;
}
public BackupInstanceResponse build() {
final var _resultValue = new BackupInstanceResponse();
_resultValue.currentProtectionState = currentProtectionState;
_resultValue.dataSourceInfo = dataSourceInfo;
_resultValue.dataSourceSetInfo = dataSourceSetInfo;
_resultValue.datasourceAuthCredentials = datasourceAuthCredentials;
_resultValue.friendlyName = friendlyName;
_resultValue.objectType = objectType;
_resultValue.policyInfo = policyInfo;
_resultValue.protectionErrorDetails = protectionErrorDetails;
_resultValue.protectionStatus = protectionStatus;
_resultValue.provisioningState = provisioningState;
_resultValue.validationType = validationType;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy