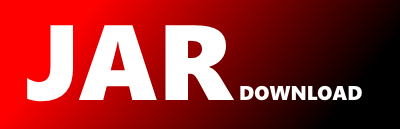
com.pulumi.azurenative.datareplication.outputs.AzStackHCIFabricModelCustomPropertiesResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.datareplication.outputs;
import com.pulumi.azurenative.datareplication.outputs.AzStackHCIClusterPropertiesResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Objects;
@CustomType
public final class AzStackHCIFabricModelCustomPropertiesResponse {
/**
* @return Gets or sets the Appliance name.
*
*/
private List applianceName;
/**
* @return Gets or sets the ARM Id of the AzStackHCI site.
*
*/
private String azStackHciSiteId;
/**
* @return AzStackHCI cluster properties.
*
*/
private AzStackHCIClusterPropertiesResponse cluster;
/**
* @return Gets or sets the fabric container Id.
*
*/
private String fabricContainerId;
/**
* @return Gets or sets the fabric resource Id.
*
*/
private String fabricResourceId;
/**
* @return Gets or sets the instance type.
* Expected value is 'AzStackHCI'.
*
*/
private String instanceType;
/**
* @return Gets or sets the migration hub Uri.
*
*/
private String migrationHubUri;
/**
* @return Gets or sets the Migration solution ARM Id.
*
*/
private String migrationSolutionId;
private AzStackHCIFabricModelCustomPropertiesResponse() {}
/**
* @return Gets or sets the Appliance name.
*
*/
public List applianceName() {
return this.applianceName;
}
/**
* @return Gets or sets the ARM Id of the AzStackHCI site.
*
*/
public String azStackHciSiteId() {
return this.azStackHciSiteId;
}
/**
* @return AzStackHCI cluster properties.
*
*/
public AzStackHCIClusterPropertiesResponse cluster() {
return this.cluster;
}
/**
* @return Gets or sets the fabric container Id.
*
*/
public String fabricContainerId() {
return this.fabricContainerId;
}
/**
* @return Gets or sets the fabric resource Id.
*
*/
public String fabricResourceId() {
return this.fabricResourceId;
}
/**
* @return Gets or sets the instance type.
* Expected value is 'AzStackHCI'.
*
*/
public String instanceType() {
return this.instanceType;
}
/**
* @return Gets or sets the migration hub Uri.
*
*/
public String migrationHubUri() {
return this.migrationHubUri;
}
/**
* @return Gets or sets the Migration solution ARM Id.
*
*/
public String migrationSolutionId() {
return this.migrationSolutionId;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(AzStackHCIFabricModelCustomPropertiesResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private List applianceName;
private String azStackHciSiteId;
private AzStackHCIClusterPropertiesResponse cluster;
private String fabricContainerId;
private String fabricResourceId;
private String instanceType;
private String migrationHubUri;
private String migrationSolutionId;
public Builder() {}
public Builder(AzStackHCIFabricModelCustomPropertiesResponse defaults) {
Objects.requireNonNull(defaults);
this.applianceName = defaults.applianceName;
this.azStackHciSiteId = defaults.azStackHciSiteId;
this.cluster = defaults.cluster;
this.fabricContainerId = defaults.fabricContainerId;
this.fabricResourceId = defaults.fabricResourceId;
this.instanceType = defaults.instanceType;
this.migrationHubUri = defaults.migrationHubUri;
this.migrationSolutionId = defaults.migrationSolutionId;
}
@CustomType.Setter
public Builder applianceName(List applianceName) {
if (applianceName == null) {
throw new MissingRequiredPropertyException("AzStackHCIFabricModelCustomPropertiesResponse", "applianceName");
}
this.applianceName = applianceName;
return this;
}
public Builder applianceName(String... applianceName) {
return applianceName(List.of(applianceName));
}
@CustomType.Setter
public Builder azStackHciSiteId(String azStackHciSiteId) {
if (azStackHciSiteId == null) {
throw new MissingRequiredPropertyException("AzStackHCIFabricModelCustomPropertiesResponse", "azStackHciSiteId");
}
this.azStackHciSiteId = azStackHciSiteId;
return this;
}
@CustomType.Setter
public Builder cluster(AzStackHCIClusterPropertiesResponse cluster) {
if (cluster == null) {
throw new MissingRequiredPropertyException("AzStackHCIFabricModelCustomPropertiesResponse", "cluster");
}
this.cluster = cluster;
return this;
}
@CustomType.Setter
public Builder fabricContainerId(String fabricContainerId) {
if (fabricContainerId == null) {
throw new MissingRequiredPropertyException("AzStackHCIFabricModelCustomPropertiesResponse", "fabricContainerId");
}
this.fabricContainerId = fabricContainerId;
return this;
}
@CustomType.Setter
public Builder fabricResourceId(String fabricResourceId) {
if (fabricResourceId == null) {
throw new MissingRequiredPropertyException("AzStackHCIFabricModelCustomPropertiesResponse", "fabricResourceId");
}
this.fabricResourceId = fabricResourceId;
return this;
}
@CustomType.Setter
public Builder instanceType(String instanceType) {
if (instanceType == null) {
throw new MissingRequiredPropertyException("AzStackHCIFabricModelCustomPropertiesResponse", "instanceType");
}
this.instanceType = instanceType;
return this;
}
@CustomType.Setter
public Builder migrationHubUri(String migrationHubUri) {
if (migrationHubUri == null) {
throw new MissingRequiredPropertyException("AzStackHCIFabricModelCustomPropertiesResponse", "migrationHubUri");
}
this.migrationHubUri = migrationHubUri;
return this;
}
@CustomType.Setter
public Builder migrationSolutionId(String migrationSolutionId) {
if (migrationSolutionId == null) {
throw new MissingRequiredPropertyException("AzStackHCIFabricModelCustomPropertiesResponse", "migrationSolutionId");
}
this.migrationSolutionId = migrationSolutionId;
return this;
}
public AzStackHCIFabricModelCustomPropertiesResponse build() {
final var _resultValue = new AzStackHCIFabricModelCustomPropertiesResponse();
_resultValue.applianceName = applianceName;
_resultValue.azStackHciSiteId = azStackHciSiteId;
_resultValue.cluster = cluster;
_resultValue.fabricContainerId = fabricContainerId;
_resultValue.fabricResourceId = fabricResourceId;
_resultValue.instanceType = instanceType;
_resultValue.migrationHubUri = migrationHubUri;
_resultValue.migrationSolutionId = migrationSolutionId;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy