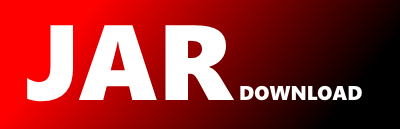
com.pulumi.azurenative.dbformariadb.inputs.ServerPropertiesForRestoreArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.dbformariadb.inputs;
import com.pulumi.azurenative.dbformariadb.enums.MinimalTlsVersionEnum;
import com.pulumi.azurenative.dbformariadb.enums.PublicNetworkAccessEnum;
import com.pulumi.azurenative.dbformariadb.enums.ServerVersion;
import com.pulumi.azurenative.dbformariadb.enums.SslEnforcementEnum;
import com.pulumi.azurenative.dbformariadb.inputs.StorageProfileArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* The properties used to create a new server by restoring from a backup.
*
*/
public final class ServerPropertiesForRestoreArgs extends com.pulumi.resources.ResourceArgs {
public static final ServerPropertiesForRestoreArgs Empty = new ServerPropertiesForRestoreArgs();
/**
* The mode to create a new server.
* Expected value is 'PointInTimeRestore'.
*
*/
@Import(name="createMode", required=true)
private Output createMode;
/**
* @return The mode to create a new server.
* Expected value is 'PointInTimeRestore'.
*
*/
public Output createMode() {
return this.createMode;
}
/**
* Enforce a minimal Tls version for the server.
*
*/
@Import(name="minimalTlsVersion")
private @Nullable Output> minimalTlsVersion;
/**
* @return Enforce a minimal Tls version for the server.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy