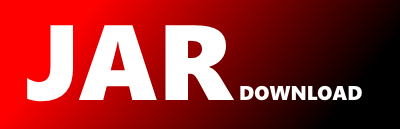
com.pulumi.azurenative.desktopvirtualization.WorkspaceArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.desktopvirtualization;
import com.pulumi.azurenative.desktopvirtualization.inputs.ResourceModelWithAllowedPropertySetIdentityArgs;
import com.pulumi.azurenative.desktopvirtualization.inputs.ResourceModelWithAllowedPropertySetPlanArgs;
import com.pulumi.azurenative.desktopvirtualization.inputs.ResourceModelWithAllowedPropertySetSkuArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class WorkspaceArgs extends com.pulumi.resources.ResourceArgs {
public static final WorkspaceArgs Empty = new WorkspaceArgs();
/**
* List of applicationGroup resource Ids.
*
*/
@Import(name="applicationGroupReferences")
private @Nullable Output> applicationGroupReferences;
/**
* @return List of applicationGroup resource Ids.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy