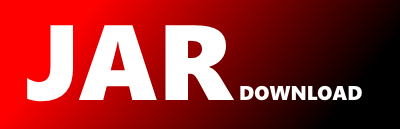
com.pulumi.azurenative.deviceupdate.DeviceupdateFunctions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.deviceupdate;
import com.pulumi.azurenative.Utilities;
import com.pulumi.azurenative.deviceupdate.inputs.GetAccountArgs;
import com.pulumi.azurenative.deviceupdate.inputs.GetAccountPlainArgs;
import com.pulumi.azurenative.deviceupdate.inputs.GetInstanceArgs;
import com.pulumi.azurenative.deviceupdate.inputs.GetInstancePlainArgs;
import com.pulumi.azurenative.deviceupdate.inputs.GetPrivateEndpointConnectionArgs;
import com.pulumi.azurenative.deviceupdate.inputs.GetPrivateEndpointConnectionPlainArgs;
import com.pulumi.azurenative.deviceupdate.inputs.GetPrivateEndpointConnectionProxyArgs;
import com.pulumi.azurenative.deviceupdate.inputs.GetPrivateEndpointConnectionProxyPlainArgs;
import com.pulumi.azurenative.deviceupdate.outputs.GetAccountResult;
import com.pulumi.azurenative.deviceupdate.outputs.GetInstanceResult;
import com.pulumi.azurenative.deviceupdate.outputs.GetPrivateEndpointConnectionProxyResult;
import com.pulumi.azurenative.deviceupdate.outputs.GetPrivateEndpointConnectionResult;
import com.pulumi.core.Output;
import com.pulumi.core.TypeShape;
import com.pulumi.deployment.Deployment;
import com.pulumi.deployment.InvokeOptions;
import java.util.concurrent.CompletableFuture;
public final class DeviceupdateFunctions {
/**
* Returns account details for the given account name.
* Azure REST API version: 2023-07-01.
*
*/
public static Output getAccount(GetAccountArgs args) {
return getAccount(args, InvokeOptions.Empty);
}
/**
* Returns account details for the given account name.
* Azure REST API version: 2023-07-01.
*
*/
public static CompletableFuture getAccountPlain(GetAccountPlainArgs args) {
return getAccountPlain(args, InvokeOptions.Empty);
}
/**
* Returns account details for the given account name.
* Azure REST API version: 2023-07-01.
*
*/
public static Output getAccount(GetAccountArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:deviceupdate:getAccount", TypeShape.of(GetAccountResult.class), args, Utilities.withVersion(options));
}
/**
* Returns account details for the given account name.
* Azure REST API version: 2023-07-01.
*
*/
public static CompletableFuture getAccountPlain(GetAccountPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:deviceupdate:getAccount", TypeShape.of(GetAccountResult.class), args, Utilities.withVersion(options));
}
/**
* Returns instance details for the given instance and account name.
* Azure REST API version: 2023-07-01.
*
*/
public static Output getInstance(GetInstanceArgs args) {
return getInstance(args, InvokeOptions.Empty);
}
/**
* Returns instance details for the given instance and account name.
* Azure REST API version: 2023-07-01.
*
*/
public static CompletableFuture getInstancePlain(GetInstancePlainArgs args) {
return getInstancePlain(args, InvokeOptions.Empty);
}
/**
* Returns instance details for the given instance and account name.
* Azure REST API version: 2023-07-01.
*
*/
public static Output getInstance(GetInstanceArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:deviceupdate:getInstance", TypeShape.of(GetInstanceResult.class), args, Utilities.withVersion(options));
}
/**
* Returns instance details for the given instance and account name.
* Azure REST API version: 2023-07-01.
*
*/
public static CompletableFuture getInstancePlain(GetInstancePlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:deviceupdate:getInstance", TypeShape.of(GetInstanceResult.class), args, Utilities.withVersion(options));
}
/**
* Get the specified private endpoint connection associated with the device update account.
* Azure REST API version: 2023-07-01.
*
*/
public static Output getPrivateEndpointConnection(GetPrivateEndpointConnectionArgs args) {
return getPrivateEndpointConnection(args, InvokeOptions.Empty);
}
/**
* Get the specified private endpoint connection associated with the device update account.
* Azure REST API version: 2023-07-01.
*
*/
public static CompletableFuture getPrivateEndpointConnectionPlain(GetPrivateEndpointConnectionPlainArgs args) {
return getPrivateEndpointConnectionPlain(args, InvokeOptions.Empty);
}
/**
* Get the specified private endpoint connection associated with the device update account.
* Azure REST API version: 2023-07-01.
*
*/
public static Output getPrivateEndpointConnection(GetPrivateEndpointConnectionArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:deviceupdate:getPrivateEndpointConnection", TypeShape.of(GetPrivateEndpointConnectionResult.class), args, Utilities.withVersion(options));
}
/**
* Get the specified private endpoint connection associated with the device update account.
* Azure REST API version: 2023-07-01.
*
*/
public static CompletableFuture getPrivateEndpointConnectionPlain(GetPrivateEndpointConnectionPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:deviceupdate:getPrivateEndpointConnection", TypeShape.of(GetPrivateEndpointConnectionResult.class), args, Utilities.withVersion(options));
}
/**
* (INTERNAL - DO NOT USE) Get the specified private endpoint connection proxy associated with the device update account.
* Azure REST API version: 2023-07-01.
*
*/
public static Output getPrivateEndpointConnectionProxy(GetPrivateEndpointConnectionProxyArgs args) {
return getPrivateEndpointConnectionProxy(args, InvokeOptions.Empty);
}
/**
* (INTERNAL - DO NOT USE) Get the specified private endpoint connection proxy associated with the device update account.
* Azure REST API version: 2023-07-01.
*
*/
public static CompletableFuture getPrivateEndpointConnectionProxyPlain(GetPrivateEndpointConnectionProxyPlainArgs args) {
return getPrivateEndpointConnectionProxyPlain(args, InvokeOptions.Empty);
}
/**
* (INTERNAL - DO NOT USE) Get the specified private endpoint connection proxy associated with the device update account.
* Azure REST API version: 2023-07-01.
*
*/
public static Output getPrivateEndpointConnectionProxy(GetPrivateEndpointConnectionProxyArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:deviceupdate:getPrivateEndpointConnectionProxy", TypeShape.of(GetPrivateEndpointConnectionProxyResult.class), args, Utilities.withVersion(options));
}
/**
* (INTERNAL - DO NOT USE) Get the specified private endpoint connection proxy associated with the device update account.
* Azure REST API version: 2023-07-01.
*
*/
public static CompletableFuture getPrivateEndpointConnectionProxyPlain(GetPrivateEndpointConnectionProxyPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:deviceupdate:getPrivateEndpointConnectionProxy", TypeShape.of(GetPrivateEndpointConnectionProxyResult.class), args, Utilities.withVersion(options));
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy