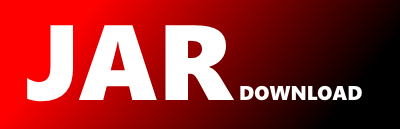
com.pulumi.azurenative.documentdb.SqlResourceSqlRoleAssignmentArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.documentdb;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class SqlResourceSqlRoleAssignmentArgs extends com.pulumi.resources.ResourceArgs {
public static final SqlResourceSqlRoleAssignmentArgs Empty = new SqlResourceSqlRoleAssignmentArgs();
/**
* Cosmos DB database account name.
*
*/
@Import(name="accountName", required=true)
private Output accountName;
/**
* @return Cosmos DB database account name.
*
*/
public Output accountName() {
return this.accountName;
}
/**
* The unique identifier for the associated AAD principal in the AAD graph to which access is being granted through this Role Assignment. Tenant ID for the principal is inferred using the tenant associated with the subscription.
*
*/
@Import(name="principalId")
private @Nullable Output principalId;
/**
* @return The unique identifier for the associated AAD principal in the AAD graph to which access is being granted through this Role Assignment. Tenant ID for the principal is inferred using the tenant associated with the subscription.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy