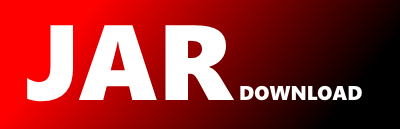
com.pulumi.azurenative.edgeorder.outputs.OrderItemDetailsResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.edgeorder.outputs;
import com.pulumi.azurenative.edgeorder.outputs.ErrorDetailResponse;
import com.pulumi.azurenative.edgeorder.outputs.ForwardShippingDetailsResponse;
import com.pulumi.azurenative.edgeorder.outputs.PreferencesResponse;
import com.pulumi.azurenative.edgeorder.outputs.ProductDetailsResponse;
import com.pulumi.azurenative.edgeorder.outputs.ResourceProviderDetailsResponse;
import com.pulumi.azurenative.edgeorder.outputs.ReverseShippingDetailsResponse;
import com.pulumi.azurenative.edgeorder.outputs.StageDetailsResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class OrderItemDetailsResponse {
/**
* @return Cancellation reason.
*
*/
private String cancellationReason;
/**
* @return Describes whether the order item is cancellable or not.
*
*/
private String cancellationStatus;
/**
* @return Current Order item Status
*
*/
private StageDetailsResponse currentStage;
/**
* @return Describes whether the order item is deletable or not.
*
*/
private String deletionStatus;
/**
* @return Top level error for the job.
*
*/
private ErrorDetailResponse error;
/**
* @return Forward Package Shipping details
*
*/
private ForwardShippingDetailsResponse forwardShippingDetails;
/**
* @return Parent RP details - this returns only the first or default parent RP from the entire list
*
*/
private ResourceProviderDetailsResponse managementRpDetails;
/**
* @return List of parent RP details supported for configuration.
*
*/
private List managementRpDetailsList;
/**
* @return Additional notification email list
*
*/
private @Nullable List notificationEmailList;
/**
* @return Defines the mode of the Order item.
*
*/
private @Nullable String orderItemMode;
/**
* @return Order item status history
*
*/
private List orderItemStageHistory;
/**
* @return Order item type.
*
*/
private String orderItemType;
/**
* @return Customer notification Preferences
*
*/
private @Nullable PreferencesResponse preferences;
/**
* @return Unique identifier for configuration.
*
*/
private ProductDetailsResponse productDetails;
/**
* @return Return reason.
*
*/
private String returnReason;
/**
* @return Describes whether the order item is returnable or not.
*
*/
private String returnStatus;
/**
* @return Reverse Package Shipping details
*
*/
private ReverseShippingDetailsResponse reverseShippingDetails;
private OrderItemDetailsResponse() {}
/**
* @return Cancellation reason.
*
*/
public String cancellationReason() {
return this.cancellationReason;
}
/**
* @return Describes whether the order item is cancellable or not.
*
*/
public String cancellationStatus() {
return this.cancellationStatus;
}
/**
* @return Current Order item Status
*
*/
public StageDetailsResponse currentStage() {
return this.currentStage;
}
/**
* @return Describes whether the order item is deletable or not.
*
*/
public String deletionStatus() {
return this.deletionStatus;
}
/**
* @return Top level error for the job.
*
*/
public ErrorDetailResponse error() {
return this.error;
}
/**
* @return Forward Package Shipping details
*
*/
public ForwardShippingDetailsResponse forwardShippingDetails() {
return this.forwardShippingDetails;
}
/**
* @return Parent RP details - this returns only the first or default parent RP from the entire list
*
*/
public ResourceProviderDetailsResponse managementRpDetails() {
return this.managementRpDetails;
}
/**
* @return List of parent RP details supported for configuration.
*
*/
public List managementRpDetailsList() {
return this.managementRpDetailsList;
}
/**
* @return Additional notification email list
*
*/
public List notificationEmailList() {
return this.notificationEmailList == null ? List.of() : this.notificationEmailList;
}
/**
* @return Defines the mode of the Order item.
*
*/
public Optional orderItemMode() {
return Optional.ofNullable(this.orderItemMode);
}
/**
* @return Order item status history
*
*/
public List orderItemStageHistory() {
return this.orderItemStageHistory;
}
/**
* @return Order item type.
*
*/
public String orderItemType() {
return this.orderItemType;
}
/**
* @return Customer notification Preferences
*
*/
public Optional preferences() {
return Optional.ofNullable(this.preferences);
}
/**
* @return Unique identifier for configuration.
*
*/
public ProductDetailsResponse productDetails() {
return this.productDetails;
}
/**
* @return Return reason.
*
*/
public String returnReason() {
return this.returnReason;
}
/**
* @return Describes whether the order item is returnable or not.
*
*/
public String returnStatus() {
return this.returnStatus;
}
/**
* @return Reverse Package Shipping details
*
*/
public ReverseShippingDetailsResponse reverseShippingDetails() {
return this.reverseShippingDetails;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(OrderItemDetailsResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String cancellationReason;
private String cancellationStatus;
private StageDetailsResponse currentStage;
private String deletionStatus;
private ErrorDetailResponse error;
private ForwardShippingDetailsResponse forwardShippingDetails;
private ResourceProviderDetailsResponse managementRpDetails;
private List managementRpDetailsList;
private @Nullable List notificationEmailList;
private @Nullable String orderItemMode;
private List orderItemStageHistory;
private String orderItemType;
private @Nullable PreferencesResponse preferences;
private ProductDetailsResponse productDetails;
private String returnReason;
private String returnStatus;
private ReverseShippingDetailsResponse reverseShippingDetails;
public Builder() {}
public Builder(OrderItemDetailsResponse defaults) {
Objects.requireNonNull(defaults);
this.cancellationReason = defaults.cancellationReason;
this.cancellationStatus = defaults.cancellationStatus;
this.currentStage = defaults.currentStage;
this.deletionStatus = defaults.deletionStatus;
this.error = defaults.error;
this.forwardShippingDetails = defaults.forwardShippingDetails;
this.managementRpDetails = defaults.managementRpDetails;
this.managementRpDetailsList = defaults.managementRpDetailsList;
this.notificationEmailList = defaults.notificationEmailList;
this.orderItemMode = defaults.orderItemMode;
this.orderItemStageHistory = defaults.orderItemStageHistory;
this.orderItemType = defaults.orderItemType;
this.preferences = defaults.preferences;
this.productDetails = defaults.productDetails;
this.returnReason = defaults.returnReason;
this.returnStatus = defaults.returnStatus;
this.reverseShippingDetails = defaults.reverseShippingDetails;
}
@CustomType.Setter
public Builder cancellationReason(String cancellationReason) {
if (cancellationReason == null) {
throw new MissingRequiredPropertyException("OrderItemDetailsResponse", "cancellationReason");
}
this.cancellationReason = cancellationReason;
return this;
}
@CustomType.Setter
public Builder cancellationStatus(String cancellationStatus) {
if (cancellationStatus == null) {
throw new MissingRequiredPropertyException("OrderItemDetailsResponse", "cancellationStatus");
}
this.cancellationStatus = cancellationStatus;
return this;
}
@CustomType.Setter
public Builder currentStage(StageDetailsResponse currentStage) {
if (currentStage == null) {
throw new MissingRequiredPropertyException("OrderItemDetailsResponse", "currentStage");
}
this.currentStage = currentStage;
return this;
}
@CustomType.Setter
public Builder deletionStatus(String deletionStatus) {
if (deletionStatus == null) {
throw new MissingRequiredPropertyException("OrderItemDetailsResponse", "deletionStatus");
}
this.deletionStatus = deletionStatus;
return this;
}
@CustomType.Setter
public Builder error(ErrorDetailResponse error) {
if (error == null) {
throw new MissingRequiredPropertyException("OrderItemDetailsResponse", "error");
}
this.error = error;
return this;
}
@CustomType.Setter
public Builder forwardShippingDetails(ForwardShippingDetailsResponse forwardShippingDetails) {
if (forwardShippingDetails == null) {
throw new MissingRequiredPropertyException("OrderItemDetailsResponse", "forwardShippingDetails");
}
this.forwardShippingDetails = forwardShippingDetails;
return this;
}
@CustomType.Setter
public Builder managementRpDetails(ResourceProviderDetailsResponse managementRpDetails) {
if (managementRpDetails == null) {
throw new MissingRequiredPropertyException("OrderItemDetailsResponse", "managementRpDetails");
}
this.managementRpDetails = managementRpDetails;
return this;
}
@CustomType.Setter
public Builder managementRpDetailsList(List managementRpDetailsList) {
if (managementRpDetailsList == null) {
throw new MissingRequiredPropertyException("OrderItemDetailsResponse", "managementRpDetailsList");
}
this.managementRpDetailsList = managementRpDetailsList;
return this;
}
public Builder managementRpDetailsList(ResourceProviderDetailsResponse... managementRpDetailsList) {
return managementRpDetailsList(List.of(managementRpDetailsList));
}
@CustomType.Setter
public Builder notificationEmailList(@Nullable List notificationEmailList) {
this.notificationEmailList = notificationEmailList;
return this;
}
public Builder notificationEmailList(String... notificationEmailList) {
return notificationEmailList(List.of(notificationEmailList));
}
@CustomType.Setter
public Builder orderItemMode(@Nullable String orderItemMode) {
this.orderItemMode = orderItemMode;
return this;
}
@CustomType.Setter
public Builder orderItemStageHistory(List orderItemStageHistory) {
if (orderItemStageHistory == null) {
throw new MissingRequiredPropertyException("OrderItemDetailsResponse", "orderItemStageHistory");
}
this.orderItemStageHistory = orderItemStageHistory;
return this;
}
public Builder orderItemStageHistory(StageDetailsResponse... orderItemStageHistory) {
return orderItemStageHistory(List.of(orderItemStageHistory));
}
@CustomType.Setter
public Builder orderItemType(String orderItemType) {
if (orderItemType == null) {
throw new MissingRequiredPropertyException("OrderItemDetailsResponse", "orderItemType");
}
this.orderItemType = orderItemType;
return this;
}
@CustomType.Setter
public Builder preferences(@Nullable PreferencesResponse preferences) {
this.preferences = preferences;
return this;
}
@CustomType.Setter
public Builder productDetails(ProductDetailsResponse productDetails) {
if (productDetails == null) {
throw new MissingRequiredPropertyException("OrderItemDetailsResponse", "productDetails");
}
this.productDetails = productDetails;
return this;
}
@CustomType.Setter
public Builder returnReason(String returnReason) {
if (returnReason == null) {
throw new MissingRequiredPropertyException("OrderItemDetailsResponse", "returnReason");
}
this.returnReason = returnReason;
return this;
}
@CustomType.Setter
public Builder returnStatus(String returnStatus) {
if (returnStatus == null) {
throw new MissingRequiredPropertyException("OrderItemDetailsResponse", "returnStatus");
}
this.returnStatus = returnStatus;
return this;
}
@CustomType.Setter
public Builder reverseShippingDetails(ReverseShippingDetailsResponse reverseShippingDetails) {
if (reverseShippingDetails == null) {
throw new MissingRequiredPropertyException("OrderItemDetailsResponse", "reverseShippingDetails");
}
this.reverseShippingDetails = reverseShippingDetails;
return this;
}
public OrderItemDetailsResponse build() {
final var _resultValue = new OrderItemDetailsResponse();
_resultValue.cancellationReason = cancellationReason;
_resultValue.cancellationStatus = cancellationStatus;
_resultValue.currentStage = currentStage;
_resultValue.deletionStatus = deletionStatus;
_resultValue.error = error;
_resultValue.forwardShippingDetails = forwardShippingDetails;
_resultValue.managementRpDetails = managementRpDetails;
_resultValue.managementRpDetailsList = managementRpDetailsList;
_resultValue.notificationEmailList = notificationEmailList;
_resultValue.orderItemMode = orderItemMode;
_resultValue.orderItemStageHistory = orderItemStageHistory;
_resultValue.orderItemType = orderItemType;
_resultValue.preferences = preferences;
_resultValue.productDetails = productDetails;
_resultValue.returnReason = returnReason;
_resultValue.returnStatus = returnStatus;
_resultValue.reverseShippingDetails = reverseShippingDetails;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy