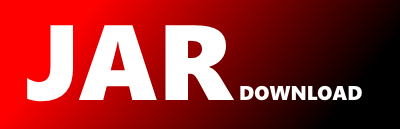
com.pulumi.azurenative.elasticsan.outputs.GetPrivateEndpointConnectionResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.elasticsan.outputs;
import com.pulumi.azurenative.elasticsan.outputs.PrivateEndpointResponse;
import com.pulumi.azurenative.elasticsan.outputs.PrivateLinkServiceConnectionStateResponse;
import com.pulumi.azurenative.elasticsan.outputs.SystemDataResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetPrivateEndpointConnectionResult {
/**
* @return List of resources private endpoint is mapped
*
*/
private @Nullable List groupIds;
/**
* @return Fully qualified resource ID for the resource. Ex - /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}
*
*/
private String id;
/**
* @return The name of the resource
*
*/
private String name;
/**
* @return Private Endpoint resource
*
*/
private @Nullable PrivateEndpointResponse privateEndpoint;
/**
* @return Private Link Service Connection State.
*
*/
private PrivateLinkServiceConnectionStateResponse privateLinkServiceConnectionState;
/**
* @return Provisioning State of Private Endpoint connection resource
*
*/
private String provisioningState;
/**
* @return Azure Resource Manager metadata containing createdBy and modifiedBy information.
*
*/
private SystemDataResponse systemData;
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
private String type;
private GetPrivateEndpointConnectionResult() {}
/**
* @return List of resources private endpoint is mapped
*
*/
public List groupIds() {
return this.groupIds == null ? List.of() : this.groupIds;
}
/**
* @return Fully qualified resource ID for the resource. Ex - /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}
*
*/
public String id() {
return this.id;
}
/**
* @return The name of the resource
*
*/
public String name() {
return this.name;
}
/**
* @return Private Endpoint resource
*
*/
public Optional privateEndpoint() {
return Optional.ofNullable(this.privateEndpoint);
}
/**
* @return Private Link Service Connection State.
*
*/
public PrivateLinkServiceConnectionStateResponse privateLinkServiceConnectionState() {
return this.privateLinkServiceConnectionState;
}
/**
* @return Provisioning State of Private Endpoint connection resource
*
*/
public String provisioningState() {
return this.provisioningState;
}
/**
* @return Azure Resource Manager metadata containing createdBy and modifiedBy information.
*
*/
public SystemDataResponse systemData() {
return this.systemData;
}
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
public String type() {
return this.type;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetPrivateEndpointConnectionResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable List groupIds;
private String id;
private String name;
private @Nullable PrivateEndpointResponse privateEndpoint;
private PrivateLinkServiceConnectionStateResponse privateLinkServiceConnectionState;
private String provisioningState;
private SystemDataResponse systemData;
private String type;
public Builder() {}
public Builder(GetPrivateEndpointConnectionResult defaults) {
Objects.requireNonNull(defaults);
this.groupIds = defaults.groupIds;
this.id = defaults.id;
this.name = defaults.name;
this.privateEndpoint = defaults.privateEndpoint;
this.privateLinkServiceConnectionState = defaults.privateLinkServiceConnectionState;
this.provisioningState = defaults.provisioningState;
this.systemData = defaults.systemData;
this.type = defaults.type;
}
@CustomType.Setter
public Builder groupIds(@Nullable List groupIds) {
this.groupIds = groupIds;
return this;
}
public Builder groupIds(String... groupIds) {
return groupIds(List.of(groupIds));
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetPrivateEndpointConnectionResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetPrivateEndpointConnectionResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder privateEndpoint(@Nullable PrivateEndpointResponse privateEndpoint) {
this.privateEndpoint = privateEndpoint;
return this;
}
@CustomType.Setter
public Builder privateLinkServiceConnectionState(PrivateLinkServiceConnectionStateResponse privateLinkServiceConnectionState) {
if (privateLinkServiceConnectionState == null) {
throw new MissingRequiredPropertyException("GetPrivateEndpointConnectionResult", "privateLinkServiceConnectionState");
}
this.privateLinkServiceConnectionState = privateLinkServiceConnectionState;
return this;
}
@CustomType.Setter
public Builder provisioningState(String provisioningState) {
if (provisioningState == null) {
throw new MissingRequiredPropertyException("GetPrivateEndpointConnectionResult", "provisioningState");
}
this.provisioningState = provisioningState;
return this;
}
@CustomType.Setter
public Builder systemData(SystemDataResponse systemData) {
if (systemData == null) {
throw new MissingRequiredPropertyException("GetPrivateEndpointConnectionResult", "systemData");
}
this.systemData = systemData;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetPrivateEndpointConnectionResult", "type");
}
this.type = type;
return this;
}
public GetPrivateEndpointConnectionResult build() {
final var _resultValue = new GetPrivateEndpointConnectionResult();
_resultValue.groupIds = groupIds;
_resultValue.id = id;
_resultValue.name = name;
_resultValue.privateEndpoint = privateEndpoint;
_resultValue.privateLinkServiceConnectionState = privateLinkServiceConnectionState;
_resultValue.provisioningState = provisioningState;
_resultValue.systemData = systemData;
_resultValue.type = type;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy