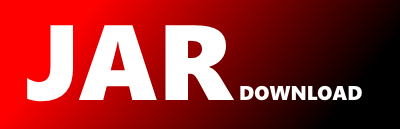
com.pulumi.azurenative.eventhub.inputs.CaptureDescriptionArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.eventhub.inputs;
import com.pulumi.azurenative.eventhub.enums.EncodingCaptureDescription;
import com.pulumi.azurenative.eventhub.inputs.DestinationArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.Boolean;
import java.lang.Integer;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Properties to configure capture description for eventhub
*
*/
public final class CaptureDescriptionArgs extends com.pulumi.resources.ResourceArgs {
public static final CaptureDescriptionArgs Empty = new CaptureDescriptionArgs();
/**
* Properties of Destination where capture will be stored. (Storage Account, Blob Names)
*
*/
@Import(name="destination")
private @Nullable Output destination;
/**
* @return Properties of Destination where capture will be stored. (Storage Account, Blob Names)
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy