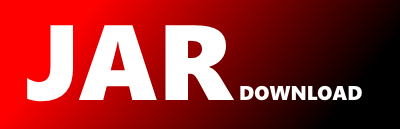
com.pulumi.azurenative.eventhub.inputs.ThrottlingPolicyArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.eventhub.inputs;
import com.pulumi.azurenative.eventhub.enums.MetricId;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Double;
import java.lang.String;
import java.util.Objects;
/**
* Properties of the throttling policy
*
*/
public final class ThrottlingPolicyArgs extends com.pulumi.resources.ResourceArgs {
public static final ThrottlingPolicyArgs Empty = new ThrottlingPolicyArgs();
/**
* Metric Id on which the throttle limit should be set, MetricId can be discovered by hovering over Metric in the Metrics section of Event Hub Namespace inside Azure Portal
*
*/
@Import(name="metricId", required=true)
private Output> metricId;
/**
* @return Metric Id on which the throttle limit should be set, MetricId can be discovered by hovering over Metric in the Metrics section of Event Hub Namespace inside Azure Portal
*
*/
public Output> metricId() {
return this.metricId;
}
/**
* The Name of this policy
*
*/
@Import(name="name", required=true)
private Output name;
/**
* @return The Name of this policy
*
*/
public Output name() {
return this.name;
}
/**
* The Threshold limit above which the application group will be throttled.Rate limit is always per second.
*
*/
@Import(name="rateLimitThreshold", required=true)
private Output rateLimitThreshold;
/**
* @return The Threshold limit above which the application group will be throttled.Rate limit is always per second.
*
*/
public Output rateLimitThreshold() {
return this.rateLimitThreshold;
}
/**
* Application Group Policy types
* Expected value is 'ThrottlingPolicy'.
*
*/
@Import(name="type", required=true)
private Output type;
/**
* @return Application Group Policy types
* Expected value is 'ThrottlingPolicy'.
*
*/
public Output type() {
return this.type;
}
private ThrottlingPolicyArgs() {}
private ThrottlingPolicyArgs(ThrottlingPolicyArgs $) {
this.metricId = $.metricId;
this.name = $.name;
this.rateLimitThreshold = $.rateLimitThreshold;
this.type = $.type;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(ThrottlingPolicyArgs defaults) {
return new Builder(defaults);
}
public static final class Builder {
private ThrottlingPolicyArgs $;
public Builder() {
$ = new ThrottlingPolicyArgs();
}
public Builder(ThrottlingPolicyArgs defaults) {
$ = new ThrottlingPolicyArgs(Objects.requireNonNull(defaults));
}
/**
* @param metricId Metric Id on which the throttle limit should be set, MetricId can be discovered by hovering over Metric in the Metrics section of Event Hub Namespace inside Azure Portal
*
* @return builder
*
*/
public Builder metricId(Output> metricId) {
$.metricId = metricId;
return this;
}
/**
* @param metricId Metric Id on which the throttle limit should be set, MetricId can be discovered by hovering over Metric in the Metrics section of Event Hub Namespace inside Azure Portal
*
* @return builder
*
*/
public Builder metricId(Either metricId) {
return metricId(Output.of(metricId));
}
/**
* @param metricId Metric Id on which the throttle limit should be set, MetricId can be discovered by hovering over Metric in the Metrics section of Event Hub Namespace inside Azure Portal
*
* @return builder
*
*/
public Builder metricId(String metricId) {
return metricId(Either.ofLeft(metricId));
}
/**
* @param metricId Metric Id on which the throttle limit should be set, MetricId can be discovered by hovering over Metric in the Metrics section of Event Hub Namespace inside Azure Portal
*
* @return builder
*
*/
public Builder metricId(MetricId metricId) {
return metricId(Either.ofRight(metricId));
}
/**
* @param name The Name of this policy
*
* @return builder
*
*/
public Builder name(Output name) {
$.name = name;
return this;
}
/**
* @param name The Name of this policy
*
* @return builder
*
*/
public Builder name(String name) {
return name(Output.of(name));
}
/**
* @param rateLimitThreshold The Threshold limit above which the application group will be throttled.Rate limit is always per second.
*
* @return builder
*
*/
public Builder rateLimitThreshold(Output rateLimitThreshold) {
$.rateLimitThreshold = rateLimitThreshold;
return this;
}
/**
* @param rateLimitThreshold The Threshold limit above which the application group will be throttled.Rate limit is always per second.
*
* @return builder
*
*/
public Builder rateLimitThreshold(Double rateLimitThreshold) {
return rateLimitThreshold(Output.of(rateLimitThreshold));
}
/**
* @param type Application Group Policy types
* Expected value is 'ThrottlingPolicy'.
*
* @return builder
*
*/
public Builder type(Output type) {
$.type = type;
return this;
}
/**
* @param type Application Group Policy types
* Expected value is 'ThrottlingPolicy'.
*
* @return builder
*
*/
public Builder type(String type) {
return type(Output.of(type));
}
public ThrottlingPolicyArgs build() {
if ($.metricId == null) {
throw new MissingRequiredPropertyException("ThrottlingPolicyArgs", "metricId");
}
if ($.name == null) {
throw new MissingRequiredPropertyException("ThrottlingPolicyArgs", "name");
}
if ($.rateLimitThreshold == null) {
throw new MissingRequiredPropertyException("ThrottlingPolicyArgs", "rateLimitThreshold");
}
$.type = Codegen.stringProp("type").output().arg($.type).require();
return $;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy