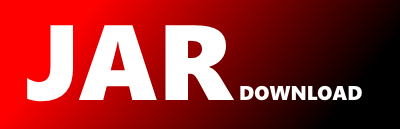
com.pulumi.azurenative.hdinsight.outputs.ClusterGetPropertiesResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.hdinsight.outputs;
import com.pulumi.azurenative.hdinsight.outputs.ClusterDefinitionResponse;
import com.pulumi.azurenative.hdinsight.outputs.ComputeIsolationPropertiesResponse;
import com.pulumi.azurenative.hdinsight.outputs.ComputeProfileResponse;
import com.pulumi.azurenative.hdinsight.outputs.ConnectivityEndpointResponse;
import com.pulumi.azurenative.hdinsight.outputs.DiskEncryptionPropertiesResponse;
import com.pulumi.azurenative.hdinsight.outputs.EncryptionInTransitPropertiesResponse;
import com.pulumi.azurenative.hdinsight.outputs.ErrorsResponse;
import com.pulumi.azurenative.hdinsight.outputs.ExcludedServicesConfigResponse;
import com.pulumi.azurenative.hdinsight.outputs.KafkaRestPropertiesResponse;
import com.pulumi.azurenative.hdinsight.outputs.NetworkPropertiesResponse;
import com.pulumi.azurenative.hdinsight.outputs.PrivateEndpointConnectionResponse;
import com.pulumi.azurenative.hdinsight.outputs.PrivateLinkConfigurationResponse;
import com.pulumi.azurenative.hdinsight.outputs.QuotaInfoResponse;
import com.pulumi.azurenative.hdinsight.outputs.SecurityProfileResponse;
import com.pulumi.azurenative.hdinsight.outputs.StorageProfileResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class ClusterGetPropertiesResponse {
/**
* @return The cluster definition.
*
*/
private ClusterDefinitionResponse clusterDefinition;
/**
* @return The hdp version of the cluster.
*
*/
private @Nullable String clusterHdpVersion;
/**
* @return The cluster id.
*
*/
private @Nullable String clusterId;
/**
* @return The state of the cluster.
*
*/
private @Nullable String clusterState;
/**
* @return The version of the cluster.
*
*/
private @Nullable String clusterVersion;
/**
* @return The compute isolation properties.
*
*/
private @Nullable ComputeIsolationPropertiesResponse computeIsolationProperties;
/**
* @return The compute profile.
*
*/
private @Nullable ComputeProfileResponse computeProfile;
/**
* @return The list of connectivity endpoints.
*
*/
private @Nullable List connectivityEndpoints;
/**
* @return The date on which the cluster was created.
*
*/
private @Nullable String createdDate;
/**
* @return The disk encryption properties.
*
*/
private @Nullable DiskEncryptionPropertiesResponse diskEncryptionProperties;
/**
* @return The encryption-in-transit properties.
*
*/
private @Nullable EncryptionInTransitPropertiesResponse encryptionInTransitProperties;
/**
* @return The list of errors.
*
*/
private @Nullable List errors;
/**
* @return The excluded services config.
*
*/
private @Nullable ExcludedServicesConfigResponse excludedServicesConfig;
/**
* @return The cluster kafka rest proxy configuration.
*
*/
private @Nullable KafkaRestPropertiesResponse kafkaRestProperties;
/**
* @return The minimal supported tls version.
*
*/
private @Nullable String minSupportedTlsVersion;
/**
* @return The network properties.
*
*/
private @Nullable NetworkPropertiesResponse networkProperties;
/**
* @return The type of operating system.
*
*/
private @Nullable String osType;
/**
* @return The list of private endpoint connections.
*
*/
private List privateEndpointConnections;
/**
* @return The private link configurations.
*
*/
private @Nullable List privateLinkConfigurations;
/**
* @return The provisioning state, which only appears in the response.
*
*/
private @Nullable String provisioningState;
/**
* @return The quota information.
*
*/
private @Nullable QuotaInfoResponse quotaInfo;
/**
* @return The security profile.
*
*/
private @Nullable SecurityProfileResponse securityProfile;
/**
* @return The storage profile.
*
*/
private @Nullable StorageProfileResponse storageProfile;
/**
* @return The cluster tier.
*
*/
private @Nullable String tier;
private ClusterGetPropertiesResponse() {}
/**
* @return The cluster definition.
*
*/
public ClusterDefinitionResponse clusterDefinition() {
return this.clusterDefinition;
}
/**
* @return The hdp version of the cluster.
*
*/
public Optional clusterHdpVersion() {
return Optional.ofNullable(this.clusterHdpVersion);
}
/**
* @return The cluster id.
*
*/
public Optional clusterId() {
return Optional.ofNullable(this.clusterId);
}
/**
* @return The state of the cluster.
*
*/
public Optional clusterState() {
return Optional.ofNullable(this.clusterState);
}
/**
* @return The version of the cluster.
*
*/
public Optional clusterVersion() {
return Optional.ofNullable(this.clusterVersion);
}
/**
* @return The compute isolation properties.
*
*/
public Optional computeIsolationProperties() {
return Optional.ofNullable(this.computeIsolationProperties);
}
/**
* @return The compute profile.
*
*/
public Optional computeProfile() {
return Optional.ofNullable(this.computeProfile);
}
/**
* @return The list of connectivity endpoints.
*
*/
public List connectivityEndpoints() {
return this.connectivityEndpoints == null ? List.of() : this.connectivityEndpoints;
}
/**
* @return The date on which the cluster was created.
*
*/
public Optional createdDate() {
return Optional.ofNullable(this.createdDate);
}
/**
* @return The disk encryption properties.
*
*/
public Optional diskEncryptionProperties() {
return Optional.ofNullable(this.diskEncryptionProperties);
}
/**
* @return The encryption-in-transit properties.
*
*/
public Optional encryptionInTransitProperties() {
return Optional.ofNullable(this.encryptionInTransitProperties);
}
/**
* @return The list of errors.
*
*/
public List errors() {
return this.errors == null ? List.of() : this.errors;
}
/**
* @return The excluded services config.
*
*/
public Optional excludedServicesConfig() {
return Optional.ofNullable(this.excludedServicesConfig);
}
/**
* @return The cluster kafka rest proxy configuration.
*
*/
public Optional kafkaRestProperties() {
return Optional.ofNullable(this.kafkaRestProperties);
}
/**
* @return The minimal supported tls version.
*
*/
public Optional minSupportedTlsVersion() {
return Optional.ofNullable(this.minSupportedTlsVersion);
}
/**
* @return The network properties.
*
*/
public Optional networkProperties() {
return Optional.ofNullable(this.networkProperties);
}
/**
* @return The type of operating system.
*
*/
public Optional osType() {
return Optional.ofNullable(this.osType);
}
/**
* @return The list of private endpoint connections.
*
*/
public List privateEndpointConnections() {
return this.privateEndpointConnections;
}
/**
* @return The private link configurations.
*
*/
public List privateLinkConfigurations() {
return this.privateLinkConfigurations == null ? List.of() : this.privateLinkConfigurations;
}
/**
* @return The provisioning state, which only appears in the response.
*
*/
public Optional provisioningState() {
return Optional.ofNullable(this.provisioningState);
}
/**
* @return The quota information.
*
*/
public Optional quotaInfo() {
return Optional.ofNullable(this.quotaInfo);
}
/**
* @return The security profile.
*
*/
public Optional securityProfile() {
return Optional.ofNullable(this.securityProfile);
}
/**
* @return The storage profile.
*
*/
public Optional storageProfile() {
return Optional.ofNullable(this.storageProfile);
}
/**
* @return The cluster tier.
*
*/
public Optional tier() {
return Optional.ofNullable(this.tier);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(ClusterGetPropertiesResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private ClusterDefinitionResponse clusterDefinition;
private @Nullable String clusterHdpVersion;
private @Nullable String clusterId;
private @Nullable String clusterState;
private @Nullable String clusterVersion;
private @Nullable ComputeIsolationPropertiesResponse computeIsolationProperties;
private @Nullable ComputeProfileResponse computeProfile;
private @Nullable List connectivityEndpoints;
private @Nullable String createdDate;
private @Nullable DiskEncryptionPropertiesResponse diskEncryptionProperties;
private @Nullable EncryptionInTransitPropertiesResponse encryptionInTransitProperties;
private @Nullable List errors;
private @Nullable ExcludedServicesConfigResponse excludedServicesConfig;
private @Nullable KafkaRestPropertiesResponse kafkaRestProperties;
private @Nullable String minSupportedTlsVersion;
private @Nullable NetworkPropertiesResponse networkProperties;
private @Nullable String osType;
private List privateEndpointConnections;
private @Nullable List privateLinkConfigurations;
private @Nullable String provisioningState;
private @Nullable QuotaInfoResponse quotaInfo;
private @Nullable SecurityProfileResponse securityProfile;
private @Nullable StorageProfileResponse storageProfile;
private @Nullable String tier;
public Builder() {}
public Builder(ClusterGetPropertiesResponse defaults) {
Objects.requireNonNull(defaults);
this.clusterDefinition = defaults.clusterDefinition;
this.clusterHdpVersion = defaults.clusterHdpVersion;
this.clusterId = defaults.clusterId;
this.clusterState = defaults.clusterState;
this.clusterVersion = defaults.clusterVersion;
this.computeIsolationProperties = defaults.computeIsolationProperties;
this.computeProfile = defaults.computeProfile;
this.connectivityEndpoints = defaults.connectivityEndpoints;
this.createdDate = defaults.createdDate;
this.diskEncryptionProperties = defaults.diskEncryptionProperties;
this.encryptionInTransitProperties = defaults.encryptionInTransitProperties;
this.errors = defaults.errors;
this.excludedServicesConfig = defaults.excludedServicesConfig;
this.kafkaRestProperties = defaults.kafkaRestProperties;
this.minSupportedTlsVersion = defaults.minSupportedTlsVersion;
this.networkProperties = defaults.networkProperties;
this.osType = defaults.osType;
this.privateEndpointConnections = defaults.privateEndpointConnections;
this.privateLinkConfigurations = defaults.privateLinkConfigurations;
this.provisioningState = defaults.provisioningState;
this.quotaInfo = defaults.quotaInfo;
this.securityProfile = defaults.securityProfile;
this.storageProfile = defaults.storageProfile;
this.tier = defaults.tier;
}
@CustomType.Setter
public Builder clusterDefinition(ClusterDefinitionResponse clusterDefinition) {
if (clusterDefinition == null) {
throw new MissingRequiredPropertyException("ClusterGetPropertiesResponse", "clusterDefinition");
}
this.clusterDefinition = clusterDefinition;
return this;
}
@CustomType.Setter
public Builder clusterHdpVersion(@Nullable String clusterHdpVersion) {
this.clusterHdpVersion = clusterHdpVersion;
return this;
}
@CustomType.Setter
public Builder clusterId(@Nullable String clusterId) {
this.clusterId = clusterId;
return this;
}
@CustomType.Setter
public Builder clusterState(@Nullable String clusterState) {
this.clusterState = clusterState;
return this;
}
@CustomType.Setter
public Builder clusterVersion(@Nullable String clusterVersion) {
this.clusterVersion = clusterVersion;
return this;
}
@CustomType.Setter
public Builder computeIsolationProperties(@Nullable ComputeIsolationPropertiesResponse computeIsolationProperties) {
this.computeIsolationProperties = computeIsolationProperties;
return this;
}
@CustomType.Setter
public Builder computeProfile(@Nullable ComputeProfileResponse computeProfile) {
this.computeProfile = computeProfile;
return this;
}
@CustomType.Setter
public Builder connectivityEndpoints(@Nullable List connectivityEndpoints) {
this.connectivityEndpoints = connectivityEndpoints;
return this;
}
public Builder connectivityEndpoints(ConnectivityEndpointResponse... connectivityEndpoints) {
return connectivityEndpoints(List.of(connectivityEndpoints));
}
@CustomType.Setter
public Builder createdDate(@Nullable String createdDate) {
this.createdDate = createdDate;
return this;
}
@CustomType.Setter
public Builder diskEncryptionProperties(@Nullable DiskEncryptionPropertiesResponse diskEncryptionProperties) {
this.diskEncryptionProperties = diskEncryptionProperties;
return this;
}
@CustomType.Setter
public Builder encryptionInTransitProperties(@Nullable EncryptionInTransitPropertiesResponse encryptionInTransitProperties) {
this.encryptionInTransitProperties = encryptionInTransitProperties;
return this;
}
@CustomType.Setter
public Builder errors(@Nullable List errors) {
this.errors = errors;
return this;
}
public Builder errors(ErrorsResponse... errors) {
return errors(List.of(errors));
}
@CustomType.Setter
public Builder excludedServicesConfig(@Nullable ExcludedServicesConfigResponse excludedServicesConfig) {
this.excludedServicesConfig = excludedServicesConfig;
return this;
}
@CustomType.Setter
public Builder kafkaRestProperties(@Nullable KafkaRestPropertiesResponse kafkaRestProperties) {
this.kafkaRestProperties = kafkaRestProperties;
return this;
}
@CustomType.Setter
public Builder minSupportedTlsVersion(@Nullable String minSupportedTlsVersion) {
this.minSupportedTlsVersion = minSupportedTlsVersion;
return this;
}
@CustomType.Setter
public Builder networkProperties(@Nullable NetworkPropertiesResponse networkProperties) {
this.networkProperties = networkProperties;
return this;
}
@CustomType.Setter
public Builder osType(@Nullable String osType) {
this.osType = osType;
return this;
}
@CustomType.Setter
public Builder privateEndpointConnections(List privateEndpointConnections) {
if (privateEndpointConnections == null) {
throw new MissingRequiredPropertyException("ClusterGetPropertiesResponse", "privateEndpointConnections");
}
this.privateEndpointConnections = privateEndpointConnections;
return this;
}
public Builder privateEndpointConnections(PrivateEndpointConnectionResponse... privateEndpointConnections) {
return privateEndpointConnections(List.of(privateEndpointConnections));
}
@CustomType.Setter
public Builder privateLinkConfigurations(@Nullable List privateLinkConfigurations) {
this.privateLinkConfigurations = privateLinkConfigurations;
return this;
}
public Builder privateLinkConfigurations(PrivateLinkConfigurationResponse... privateLinkConfigurations) {
return privateLinkConfigurations(List.of(privateLinkConfigurations));
}
@CustomType.Setter
public Builder provisioningState(@Nullable String provisioningState) {
this.provisioningState = provisioningState;
return this;
}
@CustomType.Setter
public Builder quotaInfo(@Nullable QuotaInfoResponse quotaInfo) {
this.quotaInfo = quotaInfo;
return this;
}
@CustomType.Setter
public Builder securityProfile(@Nullable SecurityProfileResponse securityProfile) {
this.securityProfile = securityProfile;
return this;
}
@CustomType.Setter
public Builder storageProfile(@Nullable StorageProfileResponse storageProfile) {
this.storageProfile = storageProfile;
return this;
}
@CustomType.Setter
public Builder tier(@Nullable String tier) {
this.tier = tier;
return this;
}
public ClusterGetPropertiesResponse build() {
final var _resultValue = new ClusterGetPropertiesResponse();
_resultValue.clusterDefinition = clusterDefinition;
_resultValue.clusterHdpVersion = clusterHdpVersion;
_resultValue.clusterId = clusterId;
_resultValue.clusterState = clusterState;
_resultValue.clusterVersion = clusterVersion;
_resultValue.computeIsolationProperties = computeIsolationProperties;
_resultValue.computeProfile = computeProfile;
_resultValue.connectivityEndpoints = connectivityEndpoints;
_resultValue.createdDate = createdDate;
_resultValue.diskEncryptionProperties = diskEncryptionProperties;
_resultValue.encryptionInTransitProperties = encryptionInTransitProperties;
_resultValue.errors = errors;
_resultValue.excludedServicesConfig = excludedServicesConfig;
_resultValue.kafkaRestProperties = kafkaRestProperties;
_resultValue.minSupportedTlsVersion = minSupportedTlsVersion;
_resultValue.networkProperties = networkProperties;
_resultValue.osType = osType;
_resultValue.privateEndpointConnections = privateEndpointConnections;
_resultValue.privateLinkConfigurations = privateLinkConfigurations;
_resultValue.provisioningState = provisioningState;
_resultValue.quotaInfo = quotaInfo;
_resultValue.securityProfile = securityProfile;
_resultValue.storageProfile = storageProfile;
_resultValue.tier = tier;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy