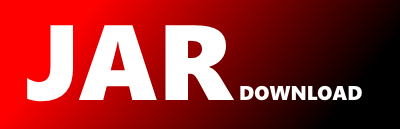
com.pulumi.azurenative.insights.outputs.GetAnalyticsItemResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.insights.outputs;
import com.pulumi.azurenative.insights.outputs.ApplicationInsightsComponentAnalyticsItemPropertiesResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetAnalyticsItemResult {
/**
* @return The content of this item
*
*/
private @Nullable String content;
/**
* @return Internally assigned unique id of the item definition.
*
*/
private @Nullable String id;
/**
* @return The user-defined name of the item.
*
*/
private @Nullable String name;
/**
* @return A set of properties that can be defined in the context of a specific item type. Each type may have its own properties.
*
*/
private ApplicationInsightsComponentAnalyticsItemPropertiesResponse properties;
/**
* @return Enum indicating if this item definition is owned by a specific user or is shared between all users with access to the Application Insights component.
*
*/
private @Nullable String scope;
/**
* @return Date and time in UTC when this item was created.
*
*/
private String timeCreated;
/**
* @return Date and time in UTC of the last modification that was made to this item.
*
*/
private String timeModified;
/**
* @return Enum indicating the type of the Analytics item.
*
*/
private @Nullable String type;
/**
* @return This instance's version of the data model. This can change as new features are added.
*
*/
private String version;
private GetAnalyticsItemResult() {}
/**
* @return The content of this item
*
*/
public Optional content() {
return Optional.ofNullable(this.content);
}
/**
* @return Internally assigned unique id of the item definition.
*
*/
public Optional id() {
return Optional.ofNullable(this.id);
}
/**
* @return The user-defined name of the item.
*
*/
public Optional name() {
return Optional.ofNullable(this.name);
}
/**
* @return A set of properties that can be defined in the context of a specific item type. Each type may have its own properties.
*
*/
public ApplicationInsightsComponentAnalyticsItemPropertiesResponse properties() {
return this.properties;
}
/**
* @return Enum indicating if this item definition is owned by a specific user or is shared between all users with access to the Application Insights component.
*
*/
public Optional scope() {
return Optional.ofNullable(this.scope);
}
/**
* @return Date and time in UTC when this item was created.
*
*/
public String timeCreated() {
return this.timeCreated;
}
/**
* @return Date and time in UTC of the last modification that was made to this item.
*
*/
public String timeModified() {
return this.timeModified;
}
/**
* @return Enum indicating the type of the Analytics item.
*
*/
public Optional type() {
return Optional.ofNullable(this.type);
}
/**
* @return This instance's version of the data model. This can change as new features are added.
*
*/
public String version() {
return this.version;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetAnalyticsItemResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String content;
private @Nullable String id;
private @Nullable String name;
private ApplicationInsightsComponentAnalyticsItemPropertiesResponse properties;
private @Nullable String scope;
private String timeCreated;
private String timeModified;
private @Nullable String type;
private String version;
public Builder() {}
public Builder(GetAnalyticsItemResult defaults) {
Objects.requireNonNull(defaults);
this.content = defaults.content;
this.id = defaults.id;
this.name = defaults.name;
this.properties = defaults.properties;
this.scope = defaults.scope;
this.timeCreated = defaults.timeCreated;
this.timeModified = defaults.timeModified;
this.type = defaults.type;
this.version = defaults.version;
}
@CustomType.Setter
public Builder content(@Nullable String content) {
this.content = content;
return this;
}
@CustomType.Setter
public Builder id(@Nullable String id) {
this.id = id;
return this;
}
@CustomType.Setter
public Builder name(@Nullable String name) {
this.name = name;
return this;
}
@CustomType.Setter
public Builder properties(ApplicationInsightsComponentAnalyticsItemPropertiesResponse properties) {
if (properties == null) {
throw new MissingRequiredPropertyException("GetAnalyticsItemResult", "properties");
}
this.properties = properties;
return this;
}
@CustomType.Setter
public Builder scope(@Nullable String scope) {
this.scope = scope;
return this;
}
@CustomType.Setter
public Builder timeCreated(String timeCreated) {
if (timeCreated == null) {
throw new MissingRequiredPropertyException("GetAnalyticsItemResult", "timeCreated");
}
this.timeCreated = timeCreated;
return this;
}
@CustomType.Setter
public Builder timeModified(String timeModified) {
if (timeModified == null) {
throw new MissingRequiredPropertyException("GetAnalyticsItemResult", "timeModified");
}
this.timeModified = timeModified;
return this;
}
@CustomType.Setter
public Builder type(@Nullable String type) {
this.type = type;
return this;
}
@CustomType.Setter
public Builder version(String version) {
if (version == null) {
throw new MissingRequiredPropertyException("GetAnalyticsItemResult", "version");
}
this.version = version;
return this;
}
public GetAnalyticsItemResult build() {
final var _resultValue = new GetAnalyticsItemResult();
_resultValue.content = content;
_resultValue.id = id;
_resultValue.name = name;
_resultValue.properties = properties;
_resultValue.scope = scope;
_resultValue.timeCreated = timeCreated;
_resultValue.timeModified = timeModified;
_resultValue.type = type;
_resultValue.version = version;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy