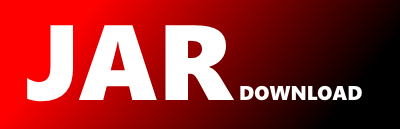
com.pulumi.azurenative.insights.outputs.GetComponentResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.insights.outputs;
import com.pulumi.azurenative.insights.outputs.PrivateLinkScopedResourceResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Double;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetComponentResult {
/**
* @return Application Insights Unique ID for your Application.
*
*/
private String appId;
/**
* @return The unique ID of your application. This field mirrors the 'Name' field and cannot be changed.
*
*/
private String applicationId;
/**
* @return Type of application being monitored.
*
*/
private String applicationType;
/**
* @return Application Insights component connection string.
*
*/
private String connectionString;
/**
* @return Creation Date for the Application Insights component, in ISO 8601 format.
*
*/
private String creationDate;
/**
* @return Disable IP masking.
*
*/
private @Nullable Boolean disableIpMasking;
/**
* @return Disable Non-AAD based Auth.
*
*/
private @Nullable Boolean disableLocalAuth;
/**
* @return Resource etag
*
*/
private @Nullable String etag;
/**
* @return Used by the Application Insights system to determine what kind of flow this component was created by. This is to be set to 'Bluefield' when creating/updating a component via the REST API.
*
*/
private @Nullable String flowType;
/**
* @return Force users to create their own storage account for profiler and debugger.
*
*/
private @Nullable Boolean forceCustomerStorageForProfiler;
/**
* @return The unique application ID created when a new application is added to HockeyApp, used for communications with HockeyApp.
*
*/
private @Nullable String hockeyAppId;
/**
* @return Token used to authenticate communications with between Application Insights and HockeyApp.
*
*/
private String hockeyAppToken;
/**
* @return Azure resource Id
*
*/
private String id;
/**
* @return Purge data immediately after 30 days.
*
*/
private @Nullable Boolean immediatePurgeDataOn30Days;
/**
* @return Indicates the flow of the ingestion.
*
*/
private @Nullable String ingestionMode;
/**
* @return Application Insights Instrumentation key. A read-only value that applications can use to identify the destination for all telemetry sent to Azure Application Insights. This value will be supplied upon construction of each new Application Insights component.
*
*/
private String instrumentationKey;
/**
* @return The kind of application that this component refers to, used to customize UI. This value is a freeform string, values should typically be one of the following: web, ios, other, store, java, phone.
*
*/
private String kind;
/**
* @return The date which the component got migrated to LA, in ISO 8601 format.
*
*/
private String laMigrationDate;
/**
* @return Resource location
*
*/
private String location;
/**
* @return Azure resource name
*
*/
private String name;
/**
* @return List of linked private link scope resources.
*
*/
private List privateLinkScopedResources;
/**
* @return Current state of this component: whether or not is has been provisioned within the resource group it is defined. Users cannot change this value but are able to read from it. Values will include Succeeded, Deploying, Canceled, and Failed.
*
*/
private String provisioningState;
/**
* @return The network access type for accessing Application Insights ingestion.
*
*/
private @Nullable String publicNetworkAccessForIngestion;
/**
* @return The network access type for accessing Application Insights query.
*
*/
private @Nullable String publicNetworkAccessForQuery;
/**
* @return Describes what tool created this Application Insights component. Customers using this API should set this to the default 'rest'.
*
*/
private @Nullable String requestSource;
/**
* @return Retention period in days.
*
*/
private @Nullable Integer retentionInDays;
/**
* @return Percentage of the data produced by the application being monitored that is being sampled for Application Insights telemetry.
*
*/
private @Nullable Double samplingPercentage;
/**
* @return Resource tags
*
*/
private @Nullable Map tags;
/**
* @return Azure Tenant Id.
*
*/
private String tenantId;
/**
* @return Azure resource type
*
*/
private String type;
/**
* @return Resource Id of the log analytics workspace which the data will be ingested to. This property is required to create an application with this API version. Applications from older versions will not have this property.
*
*/
private @Nullable String workspaceResourceId;
private GetComponentResult() {}
/**
* @return Application Insights Unique ID for your Application.
*
*/
public String appId() {
return this.appId;
}
/**
* @return The unique ID of your application. This field mirrors the 'Name' field and cannot be changed.
*
*/
public String applicationId() {
return this.applicationId;
}
/**
* @return Type of application being monitored.
*
*/
public String applicationType() {
return this.applicationType;
}
/**
* @return Application Insights component connection string.
*
*/
public String connectionString() {
return this.connectionString;
}
/**
* @return Creation Date for the Application Insights component, in ISO 8601 format.
*
*/
public String creationDate() {
return this.creationDate;
}
/**
* @return Disable IP masking.
*
*/
public Optional disableIpMasking() {
return Optional.ofNullable(this.disableIpMasking);
}
/**
* @return Disable Non-AAD based Auth.
*
*/
public Optional disableLocalAuth() {
return Optional.ofNullable(this.disableLocalAuth);
}
/**
* @return Resource etag
*
*/
public Optional etag() {
return Optional.ofNullable(this.etag);
}
/**
* @return Used by the Application Insights system to determine what kind of flow this component was created by. This is to be set to 'Bluefield' when creating/updating a component via the REST API.
*
*/
public Optional flowType() {
return Optional.ofNullable(this.flowType);
}
/**
* @return Force users to create their own storage account for profiler and debugger.
*
*/
public Optional forceCustomerStorageForProfiler() {
return Optional.ofNullable(this.forceCustomerStorageForProfiler);
}
/**
* @return The unique application ID created when a new application is added to HockeyApp, used for communications with HockeyApp.
*
*/
public Optional hockeyAppId() {
return Optional.ofNullable(this.hockeyAppId);
}
/**
* @return Token used to authenticate communications with between Application Insights and HockeyApp.
*
*/
public String hockeyAppToken() {
return this.hockeyAppToken;
}
/**
* @return Azure resource Id
*
*/
public String id() {
return this.id;
}
/**
* @return Purge data immediately after 30 days.
*
*/
public Optional immediatePurgeDataOn30Days() {
return Optional.ofNullable(this.immediatePurgeDataOn30Days);
}
/**
* @return Indicates the flow of the ingestion.
*
*/
public Optional ingestionMode() {
return Optional.ofNullable(this.ingestionMode);
}
/**
* @return Application Insights Instrumentation key. A read-only value that applications can use to identify the destination for all telemetry sent to Azure Application Insights. This value will be supplied upon construction of each new Application Insights component.
*
*/
public String instrumentationKey() {
return this.instrumentationKey;
}
/**
* @return The kind of application that this component refers to, used to customize UI. This value is a freeform string, values should typically be one of the following: web, ios, other, store, java, phone.
*
*/
public String kind() {
return this.kind;
}
/**
* @return The date which the component got migrated to LA, in ISO 8601 format.
*
*/
public String laMigrationDate() {
return this.laMigrationDate;
}
/**
* @return Resource location
*
*/
public String location() {
return this.location;
}
/**
* @return Azure resource name
*
*/
public String name() {
return this.name;
}
/**
* @return List of linked private link scope resources.
*
*/
public List privateLinkScopedResources() {
return this.privateLinkScopedResources;
}
/**
* @return Current state of this component: whether or not is has been provisioned within the resource group it is defined. Users cannot change this value but are able to read from it. Values will include Succeeded, Deploying, Canceled, and Failed.
*
*/
public String provisioningState() {
return this.provisioningState;
}
/**
* @return The network access type for accessing Application Insights ingestion.
*
*/
public Optional publicNetworkAccessForIngestion() {
return Optional.ofNullable(this.publicNetworkAccessForIngestion);
}
/**
* @return The network access type for accessing Application Insights query.
*
*/
public Optional publicNetworkAccessForQuery() {
return Optional.ofNullable(this.publicNetworkAccessForQuery);
}
/**
* @return Describes what tool created this Application Insights component. Customers using this API should set this to the default 'rest'.
*
*/
public Optional requestSource() {
return Optional.ofNullable(this.requestSource);
}
/**
* @return Retention period in days.
*
*/
public Optional retentionInDays() {
return Optional.ofNullable(this.retentionInDays);
}
/**
* @return Percentage of the data produced by the application being monitored that is being sampled for Application Insights telemetry.
*
*/
public Optional samplingPercentage() {
return Optional.ofNullable(this.samplingPercentage);
}
/**
* @return Resource tags
*
*/
public Map tags() {
return this.tags == null ? Map.of() : this.tags;
}
/**
* @return Azure Tenant Id.
*
*/
public String tenantId() {
return this.tenantId;
}
/**
* @return Azure resource type
*
*/
public String type() {
return this.type;
}
/**
* @return Resource Id of the log analytics workspace which the data will be ingested to. This property is required to create an application with this API version. Applications from older versions will not have this property.
*
*/
public Optional workspaceResourceId() {
return Optional.ofNullable(this.workspaceResourceId);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetComponentResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String appId;
private String applicationId;
private String applicationType;
private String connectionString;
private String creationDate;
private @Nullable Boolean disableIpMasking;
private @Nullable Boolean disableLocalAuth;
private @Nullable String etag;
private @Nullable String flowType;
private @Nullable Boolean forceCustomerStorageForProfiler;
private @Nullable String hockeyAppId;
private String hockeyAppToken;
private String id;
private @Nullable Boolean immediatePurgeDataOn30Days;
private @Nullable String ingestionMode;
private String instrumentationKey;
private String kind;
private String laMigrationDate;
private String location;
private String name;
private List privateLinkScopedResources;
private String provisioningState;
private @Nullable String publicNetworkAccessForIngestion;
private @Nullable String publicNetworkAccessForQuery;
private @Nullable String requestSource;
private @Nullable Integer retentionInDays;
private @Nullable Double samplingPercentage;
private @Nullable Map tags;
private String tenantId;
private String type;
private @Nullable String workspaceResourceId;
public Builder() {}
public Builder(GetComponentResult defaults) {
Objects.requireNonNull(defaults);
this.appId = defaults.appId;
this.applicationId = defaults.applicationId;
this.applicationType = defaults.applicationType;
this.connectionString = defaults.connectionString;
this.creationDate = defaults.creationDate;
this.disableIpMasking = defaults.disableIpMasking;
this.disableLocalAuth = defaults.disableLocalAuth;
this.etag = defaults.etag;
this.flowType = defaults.flowType;
this.forceCustomerStorageForProfiler = defaults.forceCustomerStorageForProfiler;
this.hockeyAppId = defaults.hockeyAppId;
this.hockeyAppToken = defaults.hockeyAppToken;
this.id = defaults.id;
this.immediatePurgeDataOn30Days = defaults.immediatePurgeDataOn30Days;
this.ingestionMode = defaults.ingestionMode;
this.instrumentationKey = defaults.instrumentationKey;
this.kind = defaults.kind;
this.laMigrationDate = defaults.laMigrationDate;
this.location = defaults.location;
this.name = defaults.name;
this.privateLinkScopedResources = defaults.privateLinkScopedResources;
this.provisioningState = defaults.provisioningState;
this.publicNetworkAccessForIngestion = defaults.publicNetworkAccessForIngestion;
this.publicNetworkAccessForQuery = defaults.publicNetworkAccessForQuery;
this.requestSource = defaults.requestSource;
this.retentionInDays = defaults.retentionInDays;
this.samplingPercentage = defaults.samplingPercentage;
this.tags = defaults.tags;
this.tenantId = defaults.tenantId;
this.type = defaults.type;
this.workspaceResourceId = defaults.workspaceResourceId;
}
@CustomType.Setter
public Builder appId(String appId) {
if (appId == null) {
throw new MissingRequiredPropertyException("GetComponentResult", "appId");
}
this.appId = appId;
return this;
}
@CustomType.Setter
public Builder applicationId(String applicationId) {
if (applicationId == null) {
throw new MissingRequiredPropertyException("GetComponentResult", "applicationId");
}
this.applicationId = applicationId;
return this;
}
@CustomType.Setter
public Builder applicationType(String applicationType) {
if (applicationType == null) {
throw new MissingRequiredPropertyException("GetComponentResult", "applicationType");
}
this.applicationType = applicationType;
return this;
}
@CustomType.Setter
public Builder connectionString(String connectionString) {
if (connectionString == null) {
throw new MissingRequiredPropertyException("GetComponentResult", "connectionString");
}
this.connectionString = connectionString;
return this;
}
@CustomType.Setter
public Builder creationDate(String creationDate) {
if (creationDate == null) {
throw new MissingRequiredPropertyException("GetComponentResult", "creationDate");
}
this.creationDate = creationDate;
return this;
}
@CustomType.Setter
public Builder disableIpMasking(@Nullable Boolean disableIpMasking) {
this.disableIpMasking = disableIpMasking;
return this;
}
@CustomType.Setter
public Builder disableLocalAuth(@Nullable Boolean disableLocalAuth) {
this.disableLocalAuth = disableLocalAuth;
return this;
}
@CustomType.Setter
public Builder etag(@Nullable String etag) {
this.etag = etag;
return this;
}
@CustomType.Setter
public Builder flowType(@Nullable String flowType) {
this.flowType = flowType;
return this;
}
@CustomType.Setter
public Builder forceCustomerStorageForProfiler(@Nullable Boolean forceCustomerStorageForProfiler) {
this.forceCustomerStorageForProfiler = forceCustomerStorageForProfiler;
return this;
}
@CustomType.Setter
public Builder hockeyAppId(@Nullable String hockeyAppId) {
this.hockeyAppId = hockeyAppId;
return this;
}
@CustomType.Setter
public Builder hockeyAppToken(String hockeyAppToken) {
if (hockeyAppToken == null) {
throw new MissingRequiredPropertyException("GetComponentResult", "hockeyAppToken");
}
this.hockeyAppToken = hockeyAppToken;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetComponentResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder immediatePurgeDataOn30Days(@Nullable Boolean immediatePurgeDataOn30Days) {
this.immediatePurgeDataOn30Days = immediatePurgeDataOn30Days;
return this;
}
@CustomType.Setter
public Builder ingestionMode(@Nullable String ingestionMode) {
this.ingestionMode = ingestionMode;
return this;
}
@CustomType.Setter
public Builder instrumentationKey(String instrumentationKey) {
if (instrumentationKey == null) {
throw new MissingRequiredPropertyException("GetComponentResult", "instrumentationKey");
}
this.instrumentationKey = instrumentationKey;
return this;
}
@CustomType.Setter
public Builder kind(String kind) {
if (kind == null) {
throw new MissingRequiredPropertyException("GetComponentResult", "kind");
}
this.kind = kind;
return this;
}
@CustomType.Setter
public Builder laMigrationDate(String laMigrationDate) {
if (laMigrationDate == null) {
throw new MissingRequiredPropertyException("GetComponentResult", "laMigrationDate");
}
this.laMigrationDate = laMigrationDate;
return this;
}
@CustomType.Setter
public Builder location(String location) {
if (location == null) {
throw new MissingRequiredPropertyException("GetComponentResult", "location");
}
this.location = location;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetComponentResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder privateLinkScopedResources(List privateLinkScopedResources) {
if (privateLinkScopedResources == null) {
throw new MissingRequiredPropertyException("GetComponentResult", "privateLinkScopedResources");
}
this.privateLinkScopedResources = privateLinkScopedResources;
return this;
}
public Builder privateLinkScopedResources(PrivateLinkScopedResourceResponse... privateLinkScopedResources) {
return privateLinkScopedResources(List.of(privateLinkScopedResources));
}
@CustomType.Setter
public Builder provisioningState(String provisioningState) {
if (provisioningState == null) {
throw new MissingRequiredPropertyException("GetComponentResult", "provisioningState");
}
this.provisioningState = provisioningState;
return this;
}
@CustomType.Setter
public Builder publicNetworkAccessForIngestion(@Nullable String publicNetworkAccessForIngestion) {
this.publicNetworkAccessForIngestion = publicNetworkAccessForIngestion;
return this;
}
@CustomType.Setter
public Builder publicNetworkAccessForQuery(@Nullable String publicNetworkAccessForQuery) {
this.publicNetworkAccessForQuery = publicNetworkAccessForQuery;
return this;
}
@CustomType.Setter
public Builder requestSource(@Nullable String requestSource) {
this.requestSource = requestSource;
return this;
}
@CustomType.Setter
public Builder retentionInDays(@Nullable Integer retentionInDays) {
this.retentionInDays = retentionInDays;
return this;
}
@CustomType.Setter
public Builder samplingPercentage(@Nullable Double samplingPercentage) {
this.samplingPercentage = samplingPercentage;
return this;
}
@CustomType.Setter
public Builder tags(@Nullable Map tags) {
this.tags = tags;
return this;
}
@CustomType.Setter
public Builder tenantId(String tenantId) {
if (tenantId == null) {
throw new MissingRequiredPropertyException("GetComponentResult", "tenantId");
}
this.tenantId = tenantId;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetComponentResult", "type");
}
this.type = type;
return this;
}
@CustomType.Setter
public Builder workspaceResourceId(@Nullable String workspaceResourceId) {
this.workspaceResourceId = workspaceResourceId;
return this;
}
public GetComponentResult build() {
final var _resultValue = new GetComponentResult();
_resultValue.appId = appId;
_resultValue.applicationId = applicationId;
_resultValue.applicationType = applicationType;
_resultValue.connectionString = connectionString;
_resultValue.creationDate = creationDate;
_resultValue.disableIpMasking = disableIpMasking;
_resultValue.disableLocalAuth = disableLocalAuth;
_resultValue.etag = etag;
_resultValue.flowType = flowType;
_resultValue.forceCustomerStorageForProfiler = forceCustomerStorageForProfiler;
_resultValue.hockeyAppId = hockeyAppId;
_resultValue.hockeyAppToken = hockeyAppToken;
_resultValue.id = id;
_resultValue.immediatePurgeDataOn30Days = immediatePurgeDataOn30Days;
_resultValue.ingestionMode = ingestionMode;
_resultValue.instrumentationKey = instrumentationKey;
_resultValue.kind = kind;
_resultValue.laMigrationDate = laMigrationDate;
_resultValue.location = location;
_resultValue.name = name;
_resultValue.privateLinkScopedResources = privateLinkScopedResources;
_resultValue.provisioningState = provisioningState;
_resultValue.publicNetworkAccessForIngestion = publicNetworkAccessForIngestion;
_resultValue.publicNetworkAccessForQuery = publicNetworkAccessForQuery;
_resultValue.requestSource = requestSource;
_resultValue.retentionInDays = retentionInDays;
_resultValue.samplingPercentage = samplingPercentage;
_resultValue.tags = tags;
_resultValue.tenantId = tenantId;
_resultValue.type = type;
_resultValue.workspaceResourceId = workspaceResourceId;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy