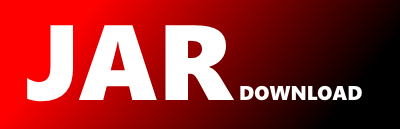
com.pulumi.azurenative.iotoperations.inputs.DataFlowBuiltInTransformationMapArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.iotoperations.inputs;
import com.pulumi.azurenative.iotoperations.enums.DataFlowMappingType;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* DataFlow BuiltIn Transformation map properties
*
*/
public final class DataFlowBuiltInTransformationMapArgs extends com.pulumi.resources.ResourceArgs {
public static final DataFlowBuiltInTransformationMapArgs Empty = new DataFlowBuiltInTransformationMapArgs();
/**
* A user provided optional description of the mapping function.
*
*/
@Import(name="description")
private @Nullable Output description;
/**
* @return A user provided optional description of the mapping function.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy