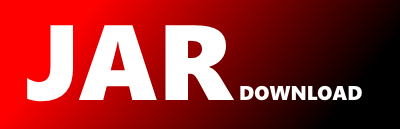
com.pulumi.azurenative.keyvault.SecretArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.keyvault;
import com.pulumi.azurenative.keyvault.inputs.SecretPropertiesArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class SecretArgs extends com.pulumi.resources.ResourceArgs {
public static final SecretArgs Empty = new SecretArgs();
/**
* Properties of the secret
*
*/
@Import(name="properties", required=true)
private Output properties;
/**
* @return Properties of the secret
*
*/
public Output properties() {
return this.properties;
}
/**
* The name of the Resource Group to which the vault belongs.
*
*/
@Import(name="resourceGroupName", required=true)
private Output resourceGroupName;
/**
* @return The name of the Resource Group to which the vault belongs.
*
*/
public Output resourceGroupName() {
return this.resourceGroupName;
}
/**
* Name of the secret. The value you provide may be copied globally for the purpose of running the service. The value provided should not include personally identifiable or sensitive information.
*
*/
@Import(name="secretName")
private @Nullable Output secretName;
/**
* @return Name of the secret. The value you provide may be copied globally for the purpose of running the service. The value provided should not include personally identifiable or sensitive information.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy