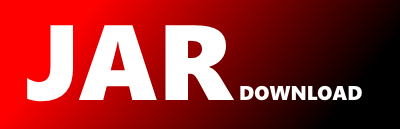
com.pulumi.azurenative.kubernetesruntime.StorageClassArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.kubernetesruntime;
import com.pulumi.azurenative.kubernetesruntime.enums.AccessMode;
import com.pulumi.azurenative.kubernetesruntime.enums.DataResilienceTier;
import com.pulumi.azurenative.kubernetesruntime.enums.FailoverTier;
import com.pulumi.azurenative.kubernetesruntime.enums.PerformanceTier;
import com.pulumi.azurenative.kubernetesruntime.enums.VolumeBindingMode;
import com.pulumi.azurenative.kubernetesruntime.enums.VolumeExpansion;
import com.pulumi.azurenative.kubernetesruntime.inputs.BlobStorageClassTypePropertiesArgs;
import com.pulumi.azurenative.kubernetesruntime.inputs.NativeStorageClassTypePropertiesArgs;
import com.pulumi.azurenative.kubernetesruntime.inputs.NfsStorageClassTypePropertiesArgs;
import com.pulumi.azurenative.kubernetesruntime.inputs.RwxStorageClassTypePropertiesArgs;
import com.pulumi.azurenative.kubernetesruntime.inputs.SmbStorageClassTypePropertiesArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Double;
import java.lang.Object;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class StorageClassArgs extends com.pulumi.resources.ResourceArgs {
public static final StorageClassArgs Empty = new StorageClassArgs();
/**
* The access mode: [ReadWriteOnce, ReadWriteMany] or [ReadWriteOnce]
*
*/
@Import(name="accessModes")
private @Nullable Output>> accessModes;
/**
* @return The access mode: [ReadWriteOnce, ReadWriteMany] or [ReadWriteOnce]
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy