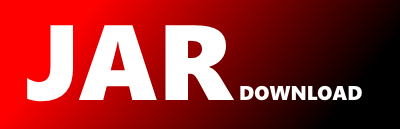
com.pulumi.azurenative.kusto.EventHubDataConnectionArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.kusto;
import com.pulumi.azurenative.kusto.enums.Compression;
import com.pulumi.azurenative.kusto.enums.DatabaseRouting;
import com.pulumi.azurenative.kusto.enums.EventHubDataFormat;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class EventHubDataConnectionArgs extends com.pulumi.resources.ResourceArgs {
public static final EventHubDataConnectionArgs Empty = new EventHubDataConnectionArgs();
/**
* The name of the Kusto cluster.
*
*/
@Import(name="clusterName", required=true)
private Output clusterName;
/**
* @return The name of the Kusto cluster.
*
*/
public Output clusterName() {
return this.clusterName;
}
/**
* The event hub messages compression type
*
*/
@Import(name="compression")
private @Nullable Output> compression;
/**
* @return The event hub messages compression type
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy