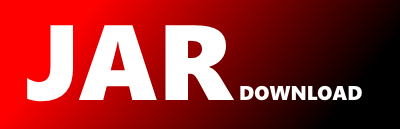
com.pulumi.azurenative.kusto.outputs.GetClusterResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.kusto.outputs;
import com.pulumi.azurenative.kusto.outputs.AcceptedAudiencesResponse;
import com.pulumi.azurenative.kusto.outputs.AzureSkuResponse;
import com.pulumi.azurenative.kusto.outputs.IdentityResponse;
import com.pulumi.azurenative.kusto.outputs.KeyVaultPropertiesResponse;
import com.pulumi.azurenative.kusto.outputs.LanguageExtensionsListResponse;
import com.pulumi.azurenative.kusto.outputs.OptimizedAutoscaleResponse;
import com.pulumi.azurenative.kusto.outputs.PrivateEndpointConnectionResponse;
import com.pulumi.azurenative.kusto.outputs.SystemDataResponse;
import com.pulumi.azurenative.kusto.outputs.TrustedExternalTenantResponse;
import com.pulumi.azurenative.kusto.outputs.VirtualNetworkConfigurationResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetClusterResult {
/**
* @return The cluster's accepted audiences.
*
*/
private @Nullable List acceptedAudiences;
/**
* @return List of allowed FQDNs(Fully Qualified Domain Name) for egress from Cluster.
*
*/
private @Nullable List allowedFqdnList;
/**
* @return The list of ips in the format of CIDR allowed to connect to the cluster.
*
*/
private @Nullable List allowedIpRangeList;
/**
* @return The cluster data ingestion URI.
*
*/
private String dataIngestionUri;
/**
* @return A boolean value that indicates if the cluster could be automatically stopped (due to lack of data or no activity for many days).
*
*/
private @Nullable Boolean enableAutoStop;
/**
* @return A boolean value that indicates if the cluster's disks are encrypted.
*
*/
private @Nullable Boolean enableDiskEncryption;
/**
* @return A boolean value that indicates if double encryption is enabled.
*
*/
private @Nullable Boolean enableDoubleEncryption;
/**
* @return A boolean value that indicates if the purge operations are enabled.
*
*/
private @Nullable Boolean enablePurge;
/**
* @return A boolean value that indicates if the streaming ingest is enabled.
*
*/
private @Nullable Boolean enableStreamingIngest;
/**
* @return The engine type
*
*/
private @Nullable String engineType;
/**
* @return A unique read-only string that changes whenever the resource is updated.
*
*/
private String etag;
/**
* @return Fully qualified resource ID for the resource. Ex - /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}
*
*/
private String id;
/**
* @return The identity of the cluster, if configured.
*
*/
private @Nullable IdentityResponse identity;
/**
* @return KeyVault properties for the cluster encryption.
*
*/
private @Nullable KeyVaultPropertiesResponse keyVaultProperties;
/**
* @return List of the cluster's language extensions.
*
*/
private @Nullable LanguageExtensionsListResponse languageExtensions;
/**
* @return The geo-location where the resource lives
*
*/
private String location;
/**
* @return The name of the resource
*
*/
private String name;
/**
* @return Optimized auto scale definition.
*
*/
private @Nullable OptimizedAutoscaleResponse optimizedAutoscale;
/**
* @return A list of private endpoint connections.
*
*/
private List privateEndpointConnections;
/**
* @return The provisioned state of the resource.
*
*/
private String provisioningState;
/**
* @return Indicates what public IP type to create - IPv4 (default), or DualStack (both IPv4 and IPv6)
*
*/
private @Nullable String publicIPType;
/**
* @return Public network access to the cluster is enabled by default. When disabled, only private endpoint connection to the cluster is allowed
*
*/
private @Nullable String publicNetworkAccess;
/**
* @return Whether or not to restrict outbound network access. Value is optional but if passed in, must be 'Enabled' or 'Disabled'
*
*/
private @Nullable String restrictOutboundNetworkAccess;
/**
* @return The SKU of the cluster.
*
*/
private AzureSkuResponse sku;
/**
* @return The state of the resource.
*
*/
private String state;
/**
* @return The reason for the cluster's current state.
*
*/
private String stateReason;
/**
* @return Metadata pertaining to creation and last modification of the resource.
*
*/
private SystemDataResponse systemData;
/**
* @return Resource tags.
*
*/
private @Nullable Map tags;
/**
* @return The cluster's external tenants.
*
*/
private @Nullable List trustedExternalTenants;
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
private String type;
/**
* @return The cluster URI.
*
*/
private String uri;
/**
* @return Virtual network definition.
*
*/
private @Nullable VirtualNetworkConfigurationResponse virtualNetworkConfiguration;
/**
* @return The availability zones of the cluster.
*
*/
private @Nullable List zones;
private GetClusterResult() {}
/**
* @return The cluster's accepted audiences.
*
*/
public List acceptedAudiences() {
return this.acceptedAudiences == null ? List.of() : this.acceptedAudiences;
}
/**
* @return List of allowed FQDNs(Fully Qualified Domain Name) for egress from Cluster.
*
*/
public List allowedFqdnList() {
return this.allowedFqdnList == null ? List.of() : this.allowedFqdnList;
}
/**
* @return The list of ips in the format of CIDR allowed to connect to the cluster.
*
*/
public List allowedIpRangeList() {
return this.allowedIpRangeList == null ? List.of() : this.allowedIpRangeList;
}
/**
* @return The cluster data ingestion URI.
*
*/
public String dataIngestionUri() {
return this.dataIngestionUri;
}
/**
* @return A boolean value that indicates if the cluster could be automatically stopped (due to lack of data or no activity for many days).
*
*/
public Optional enableAutoStop() {
return Optional.ofNullable(this.enableAutoStop);
}
/**
* @return A boolean value that indicates if the cluster's disks are encrypted.
*
*/
public Optional enableDiskEncryption() {
return Optional.ofNullable(this.enableDiskEncryption);
}
/**
* @return A boolean value that indicates if double encryption is enabled.
*
*/
public Optional enableDoubleEncryption() {
return Optional.ofNullable(this.enableDoubleEncryption);
}
/**
* @return A boolean value that indicates if the purge operations are enabled.
*
*/
public Optional enablePurge() {
return Optional.ofNullable(this.enablePurge);
}
/**
* @return A boolean value that indicates if the streaming ingest is enabled.
*
*/
public Optional enableStreamingIngest() {
return Optional.ofNullable(this.enableStreamingIngest);
}
/**
* @return The engine type
*
*/
public Optional engineType() {
return Optional.ofNullable(this.engineType);
}
/**
* @return A unique read-only string that changes whenever the resource is updated.
*
*/
public String etag() {
return this.etag;
}
/**
* @return Fully qualified resource ID for the resource. Ex - /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}
*
*/
public String id() {
return this.id;
}
/**
* @return The identity of the cluster, if configured.
*
*/
public Optional identity() {
return Optional.ofNullable(this.identity);
}
/**
* @return KeyVault properties for the cluster encryption.
*
*/
public Optional keyVaultProperties() {
return Optional.ofNullable(this.keyVaultProperties);
}
/**
* @return List of the cluster's language extensions.
*
*/
public Optional languageExtensions() {
return Optional.ofNullable(this.languageExtensions);
}
/**
* @return The geo-location where the resource lives
*
*/
public String location() {
return this.location;
}
/**
* @return The name of the resource
*
*/
public String name() {
return this.name;
}
/**
* @return Optimized auto scale definition.
*
*/
public Optional optimizedAutoscale() {
return Optional.ofNullable(this.optimizedAutoscale);
}
/**
* @return A list of private endpoint connections.
*
*/
public List privateEndpointConnections() {
return this.privateEndpointConnections;
}
/**
* @return The provisioned state of the resource.
*
*/
public String provisioningState() {
return this.provisioningState;
}
/**
* @return Indicates what public IP type to create - IPv4 (default), or DualStack (both IPv4 and IPv6)
*
*/
public Optional publicIPType() {
return Optional.ofNullable(this.publicIPType);
}
/**
* @return Public network access to the cluster is enabled by default. When disabled, only private endpoint connection to the cluster is allowed
*
*/
public Optional publicNetworkAccess() {
return Optional.ofNullable(this.publicNetworkAccess);
}
/**
* @return Whether or not to restrict outbound network access. Value is optional but if passed in, must be 'Enabled' or 'Disabled'
*
*/
public Optional restrictOutboundNetworkAccess() {
return Optional.ofNullable(this.restrictOutboundNetworkAccess);
}
/**
* @return The SKU of the cluster.
*
*/
public AzureSkuResponse sku() {
return this.sku;
}
/**
* @return The state of the resource.
*
*/
public String state() {
return this.state;
}
/**
* @return The reason for the cluster's current state.
*
*/
public String stateReason() {
return this.stateReason;
}
/**
* @return Metadata pertaining to creation and last modification of the resource.
*
*/
public SystemDataResponse systemData() {
return this.systemData;
}
/**
* @return Resource tags.
*
*/
public Map tags() {
return this.tags == null ? Map.of() : this.tags;
}
/**
* @return The cluster's external tenants.
*
*/
public List trustedExternalTenants() {
return this.trustedExternalTenants == null ? List.of() : this.trustedExternalTenants;
}
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
public String type() {
return this.type;
}
/**
* @return The cluster URI.
*
*/
public String uri() {
return this.uri;
}
/**
* @return Virtual network definition.
*
*/
public Optional virtualNetworkConfiguration() {
return Optional.ofNullable(this.virtualNetworkConfiguration);
}
/**
* @return The availability zones of the cluster.
*
*/
public List zones() {
return this.zones == null ? List.of() : this.zones;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetClusterResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable List acceptedAudiences;
private @Nullable List allowedFqdnList;
private @Nullable List allowedIpRangeList;
private String dataIngestionUri;
private @Nullable Boolean enableAutoStop;
private @Nullable Boolean enableDiskEncryption;
private @Nullable Boolean enableDoubleEncryption;
private @Nullable Boolean enablePurge;
private @Nullable Boolean enableStreamingIngest;
private @Nullable String engineType;
private String etag;
private String id;
private @Nullable IdentityResponse identity;
private @Nullable KeyVaultPropertiesResponse keyVaultProperties;
private @Nullable LanguageExtensionsListResponse languageExtensions;
private String location;
private String name;
private @Nullable OptimizedAutoscaleResponse optimizedAutoscale;
private List privateEndpointConnections;
private String provisioningState;
private @Nullable String publicIPType;
private @Nullable String publicNetworkAccess;
private @Nullable String restrictOutboundNetworkAccess;
private AzureSkuResponse sku;
private String state;
private String stateReason;
private SystemDataResponse systemData;
private @Nullable Map tags;
private @Nullable List trustedExternalTenants;
private String type;
private String uri;
private @Nullable VirtualNetworkConfigurationResponse virtualNetworkConfiguration;
private @Nullable List zones;
public Builder() {}
public Builder(GetClusterResult defaults) {
Objects.requireNonNull(defaults);
this.acceptedAudiences = defaults.acceptedAudiences;
this.allowedFqdnList = defaults.allowedFqdnList;
this.allowedIpRangeList = defaults.allowedIpRangeList;
this.dataIngestionUri = defaults.dataIngestionUri;
this.enableAutoStop = defaults.enableAutoStop;
this.enableDiskEncryption = defaults.enableDiskEncryption;
this.enableDoubleEncryption = defaults.enableDoubleEncryption;
this.enablePurge = defaults.enablePurge;
this.enableStreamingIngest = defaults.enableStreamingIngest;
this.engineType = defaults.engineType;
this.etag = defaults.etag;
this.id = defaults.id;
this.identity = defaults.identity;
this.keyVaultProperties = defaults.keyVaultProperties;
this.languageExtensions = defaults.languageExtensions;
this.location = defaults.location;
this.name = defaults.name;
this.optimizedAutoscale = defaults.optimizedAutoscale;
this.privateEndpointConnections = defaults.privateEndpointConnections;
this.provisioningState = defaults.provisioningState;
this.publicIPType = defaults.publicIPType;
this.publicNetworkAccess = defaults.publicNetworkAccess;
this.restrictOutboundNetworkAccess = defaults.restrictOutboundNetworkAccess;
this.sku = defaults.sku;
this.state = defaults.state;
this.stateReason = defaults.stateReason;
this.systemData = defaults.systemData;
this.tags = defaults.tags;
this.trustedExternalTenants = defaults.trustedExternalTenants;
this.type = defaults.type;
this.uri = defaults.uri;
this.virtualNetworkConfiguration = defaults.virtualNetworkConfiguration;
this.zones = defaults.zones;
}
@CustomType.Setter
public Builder acceptedAudiences(@Nullable List acceptedAudiences) {
this.acceptedAudiences = acceptedAudiences;
return this;
}
public Builder acceptedAudiences(AcceptedAudiencesResponse... acceptedAudiences) {
return acceptedAudiences(List.of(acceptedAudiences));
}
@CustomType.Setter
public Builder allowedFqdnList(@Nullable List allowedFqdnList) {
this.allowedFqdnList = allowedFqdnList;
return this;
}
public Builder allowedFqdnList(String... allowedFqdnList) {
return allowedFqdnList(List.of(allowedFqdnList));
}
@CustomType.Setter
public Builder allowedIpRangeList(@Nullable List allowedIpRangeList) {
this.allowedIpRangeList = allowedIpRangeList;
return this;
}
public Builder allowedIpRangeList(String... allowedIpRangeList) {
return allowedIpRangeList(List.of(allowedIpRangeList));
}
@CustomType.Setter
public Builder dataIngestionUri(String dataIngestionUri) {
if (dataIngestionUri == null) {
throw new MissingRequiredPropertyException("GetClusterResult", "dataIngestionUri");
}
this.dataIngestionUri = dataIngestionUri;
return this;
}
@CustomType.Setter
public Builder enableAutoStop(@Nullable Boolean enableAutoStop) {
this.enableAutoStop = enableAutoStop;
return this;
}
@CustomType.Setter
public Builder enableDiskEncryption(@Nullable Boolean enableDiskEncryption) {
this.enableDiskEncryption = enableDiskEncryption;
return this;
}
@CustomType.Setter
public Builder enableDoubleEncryption(@Nullable Boolean enableDoubleEncryption) {
this.enableDoubleEncryption = enableDoubleEncryption;
return this;
}
@CustomType.Setter
public Builder enablePurge(@Nullable Boolean enablePurge) {
this.enablePurge = enablePurge;
return this;
}
@CustomType.Setter
public Builder enableStreamingIngest(@Nullable Boolean enableStreamingIngest) {
this.enableStreamingIngest = enableStreamingIngest;
return this;
}
@CustomType.Setter
public Builder engineType(@Nullable String engineType) {
this.engineType = engineType;
return this;
}
@CustomType.Setter
public Builder etag(String etag) {
if (etag == null) {
throw new MissingRequiredPropertyException("GetClusterResult", "etag");
}
this.etag = etag;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetClusterResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder identity(@Nullable IdentityResponse identity) {
this.identity = identity;
return this;
}
@CustomType.Setter
public Builder keyVaultProperties(@Nullable KeyVaultPropertiesResponse keyVaultProperties) {
this.keyVaultProperties = keyVaultProperties;
return this;
}
@CustomType.Setter
public Builder languageExtensions(@Nullable LanguageExtensionsListResponse languageExtensions) {
this.languageExtensions = languageExtensions;
return this;
}
@CustomType.Setter
public Builder location(String location) {
if (location == null) {
throw new MissingRequiredPropertyException("GetClusterResult", "location");
}
this.location = location;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetClusterResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder optimizedAutoscale(@Nullable OptimizedAutoscaleResponse optimizedAutoscale) {
this.optimizedAutoscale = optimizedAutoscale;
return this;
}
@CustomType.Setter
public Builder privateEndpointConnections(List privateEndpointConnections) {
if (privateEndpointConnections == null) {
throw new MissingRequiredPropertyException("GetClusterResult", "privateEndpointConnections");
}
this.privateEndpointConnections = privateEndpointConnections;
return this;
}
public Builder privateEndpointConnections(PrivateEndpointConnectionResponse... privateEndpointConnections) {
return privateEndpointConnections(List.of(privateEndpointConnections));
}
@CustomType.Setter
public Builder provisioningState(String provisioningState) {
if (provisioningState == null) {
throw new MissingRequiredPropertyException("GetClusterResult", "provisioningState");
}
this.provisioningState = provisioningState;
return this;
}
@CustomType.Setter
public Builder publicIPType(@Nullable String publicIPType) {
this.publicIPType = publicIPType;
return this;
}
@CustomType.Setter
public Builder publicNetworkAccess(@Nullable String publicNetworkAccess) {
this.publicNetworkAccess = publicNetworkAccess;
return this;
}
@CustomType.Setter
public Builder restrictOutboundNetworkAccess(@Nullable String restrictOutboundNetworkAccess) {
this.restrictOutboundNetworkAccess = restrictOutboundNetworkAccess;
return this;
}
@CustomType.Setter
public Builder sku(AzureSkuResponse sku) {
if (sku == null) {
throw new MissingRequiredPropertyException("GetClusterResult", "sku");
}
this.sku = sku;
return this;
}
@CustomType.Setter
public Builder state(String state) {
if (state == null) {
throw new MissingRequiredPropertyException("GetClusterResult", "state");
}
this.state = state;
return this;
}
@CustomType.Setter
public Builder stateReason(String stateReason) {
if (stateReason == null) {
throw new MissingRequiredPropertyException("GetClusterResult", "stateReason");
}
this.stateReason = stateReason;
return this;
}
@CustomType.Setter
public Builder systemData(SystemDataResponse systemData) {
if (systemData == null) {
throw new MissingRequiredPropertyException("GetClusterResult", "systemData");
}
this.systemData = systemData;
return this;
}
@CustomType.Setter
public Builder tags(@Nullable Map tags) {
this.tags = tags;
return this;
}
@CustomType.Setter
public Builder trustedExternalTenants(@Nullable List trustedExternalTenants) {
this.trustedExternalTenants = trustedExternalTenants;
return this;
}
public Builder trustedExternalTenants(TrustedExternalTenantResponse... trustedExternalTenants) {
return trustedExternalTenants(List.of(trustedExternalTenants));
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetClusterResult", "type");
}
this.type = type;
return this;
}
@CustomType.Setter
public Builder uri(String uri) {
if (uri == null) {
throw new MissingRequiredPropertyException("GetClusterResult", "uri");
}
this.uri = uri;
return this;
}
@CustomType.Setter
public Builder virtualNetworkConfiguration(@Nullable VirtualNetworkConfigurationResponse virtualNetworkConfiguration) {
this.virtualNetworkConfiguration = virtualNetworkConfiguration;
return this;
}
@CustomType.Setter
public Builder zones(@Nullable List zones) {
this.zones = zones;
return this;
}
public Builder zones(String... zones) {
return zones(List.of(zones));
}
public GetClusterResult build() {
final var _resultValue = new GetClusterResult();
_resultValue.acceptedAudiences = acceptedAudiences;
_resultValue.allowedFqdnList = allowedFqdnList;
_resultValue.allowedIpRangeList = allowedIpRangeList;
_resultValue.dataIngestionUri = dataIngestionUri;
_resultValue.enableAutoStop = enableAutoStop;
_resultValue.enableDiskEncryption = enableDiskEncryption;
_resultValue.enableDoubleEncryption = enableDoubleEncryption;
_resultValue.enablePurge = enablePurge;
_resultValue.enableStreamingIngest = enableStreamingIngest;
_resultValue.engineType = engineType;
_resultValue.etag = etag;
_resultValue.id = id;
_resultValue.identity = identity;
_resultValue.keyVaultProperties = keyVaultProperties;
_resultValue.languageExtensions = languageExtensions;
_resultValue.location = location;
_resultValue.name = name;
_resultValue.optimizedAutoscale = optimizedAutoscale;
_resultValue.privateEndpointConnections = privateEndpointConnections;
_resultValue.provisioningState = provisioningState;
_resultValue.publicIPType = publicIPType;
_resultValue.publicNetworkAccess = publicNetworkAccess;
_resultValue.restrictOutboundNetworkAccess = restrictOutboundNetworkAccess;
_resultValue.sku = sku;
_resultValue.state = state;
_resultValue.stateReason = stateReason;
_resultValue.systemData = systemData;
_resultValue.tags = tags;
_resultValue.trustedExternalTenants = trustedExternalTenants;
_resultValue.type = type;
_resultValue.uri = uri;
_resultValue.virtualNetworkConfiguration = virtualNetworkConfiguration;
_resultValue.zones = zones;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy