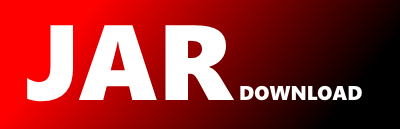
com.pulumi.azurenative.labservices.Lab Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.labservices;
import com.pulumi.azurenative.Utilities;
import com.pulumi.azurenative.labservices.LabArgs;
import com.pulumi.azurenative.labservices.outputs.AutoShutdownProfileResponse;
import com.pulumi.azurenative.labservices.outputs.ConnectionProfileResponse;
import com.pulumi.azurenative.labservices.outputs.LabNetworkProfileResponse;
import com.pulumi.azurenative.labservices.outputs.RosterProfileResponse;
import com.pulumi.azurenative.labservices.outputs.SecurityProfileResponse;
import com.pulumi.azurenative.labservices.outputs.SystemDataResponse;
import com.pulumi.azurenative.labservices.outputs.VirtualMachineProfileResponse;
import com.pulumi.core.Alias;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* The lab resource.
* Azure REST API version: 2022-08-01. Prior API version in Azure Native 1.x: 2018-10-15.
*
* Other available API versions: 2018-10-15, 2023-06-07.
*
* ## Example Usage
* ### putLab
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.labservices.Lab;
* import com.pulumi.azurenative.labservices.LabArgs;
* import com.pulumi.azurenative.labservices.inputs.AutoShutdownProfileArgs;
* import com.pulumi.azurenative.labservices.inputs.ConnectionProfileArgs;
* import com.pulumi.azurenative.labservices.inputs.LabNetworkProfileArgs;
* import com.pulumi.azurenative.labservices.inputs.SecurityProfileArgs;
* import com.pulumi.azurenative.labservices.inputs.VirtualMachineProfileArgs;
* import com.pulumi.azurenative.labservices.inputs.VirtualMachineAdditionalCapabilitiesArgs;
* import com.pulumi.azurenative.labservices.inputs.CredentialsArgs;
* import com.pulumi.azurenative.labservices.inputs.ImageReferenceArgs;
* import com.pulumi.azurenative.labservices.inputs.SkuArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var lab = new Lab("lab", LabArgs.builder()
* .autoShutdownProfile(AutoShutdownProfileArgs.builder()
* .disconnectDelay("PT5M")
* .idleDelay("PT5M")
* .noConnectDelay("PT5M")
* .shutdownOnDisconnect("Enabled")
* .shutdownOnIdle("UserAbsence")
* .shutdownWhenNotConnected("Enabled")
* .build())
* .connectionProfile(ConnectionProfileArgs.builder()
* .clientRdpAccess("Public")
* .clientSshAccess("Public")
* .webRdpAccess("None")
* .webSshAccess("None")
* .build())
* .description("This is a test lab.")
* .labName("testlab")
* .labPlanId("/subscriptions/34adfa4f-cedf-4dc0-ba29-b6d1a69ab345/resourceGroups/testrg123/providers/Microsoft.LabServices/labPlans/testlabplan")
* .location("westus")
* .networkProfile(LabNetworkProfileArgs.builder()
* .subnetId("/subscriptions/34adfa4f-cedf-4dc0-ba29-b6d1a69ab345/resourceGroups/testrg123/providers/Microsoft.Network/virtualNetworks/test-vnet/subnets/default")
* .build())
* .resourceGroupName("testrg123")
* .securityProfile(SecurityProfileArgs.builder()
* .openAccess("Disabled")
* .build())
* .title("Test Lab")
* .virtualMachineProfile(VirtualMachineProfileArgs.builder()
* .additionalCapabilities(VirtualMachineAdditionalCapabilitiesArgs.builder()
* .installGpuDrivers("Disabled")
* .build())
* .adminUser(CredentialsArgs.builder()
* .username("test-user")
* .build())
* .createOption("TemplateVM")
* .imageReference(ImageReferenceArgs.builder()
* .offer("WindowsServer")
* .publisher("Microsoft")
* .sku("2019-Datacenter")
* .version("2019.0.20190410")
* .build())
* .sku(SkuArgs.builder()
* .name("Medium")
* .build())
* .usageQuota("PT10H")
* .useSharedPassword("Disabled")
* .build())
* .build());
*
* }
* }
*
* }
*
*
* ## Import
*
* An existing resource can be imported using its type token, name, and identifier, e.g.
*
* ```sh
* $ pulumi import azure-native:labservices:Lab testlabplan /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.LabServices/labs/{labName}
* ```
*
*/
@ResourceType(type="azure-native:labservices:Lab")
public class Lab extends com.pulumi.resources.CustomResource {
/**
* The resource auto shutdown configuration for the lab. This controls whether actions are taken on resources that are sitting idle.
*
*/
@Export(name="autoShutdownProfile", refs={AutoShutdownProfileResponse.class}, tree="[0]")
private Output autoShutdownProfile;
/**
* @return The resource auto shutdown configuration for the lab. This controls whether actions are taken on resources that are sitting idle.
*
*/
public Output autoShutdownProfile() {
return this.autoShutdownProfile;
}
/**
* The connection profile for the lab. This controls settings such as web access to lab resources or whether RDP or SSH ports are open.
*
*/
@Export(name="connectionProfile", refs={ConnectionProfileResponse.class}, tree="[0]")
private Output connectionProfile;
/**
* @return The connection profile for the lab. This controls settings such as web access to lab resources or whether RDP or SSH ports are open.
*
*/
public Output connectionProfile() {
return this.connectionProfile;
}
/**
* The description of the lab.
*
*/
@Export(name="description", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> description;
/**
* @return The description of the lab.
*
*/
public Output> description() {
return Codegen.optional(this.description);
}
/**
* The ID of the lab plan. Used during resource creation to provide defaults and acts as a permission container when creating a lab via labs.azure.com. Setting a labPlanId on an existing lab provides organization..
*
*/
@Export(name="labPlanId", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> labPlanId;
/**
* @return The ID of the lab plan. Used during resource creation to provide defaults and acts as a permission container when creating a lab via labs.azure.com. Setting a labPlanId on an existing lab provides organization..
*
*/
public Output> labPlanId() {
return Codegen.optional(this.labPlanId);
}
/**
* The geo-location where the resource lives
*
*/
@Export(name="location", refs={String.class}, tree="[0]")
private Output location;
/**
* @return The geo-location where the resource lives
*
*/
public Output location() {
return this.location;
}
/**
* The name of the resource
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return The name of the resource
*
*/
public Output name() {
return this.name;
}
/**
* The network profile for the lab, typically applied via a lab plan. This profile cannot be modified once a lab has been created.
*
*/
@Export(name="networkProfile", refs={LabNetworkProfileResponse.class}, tree="[0]")
private Output* @Nullable */ LabNetworkProfileResponse> networkProfile;
/**
* @return The network profile for the lab, typically applied via a lab plan. This profile cannot be modified once a lab has been created.
*
*/
public Output> networkProfile() {
return Codegen.optional(this.networkProfile);
}
/**
* Current provisioning state of the lab.
*
*/
@Export(name="provisioningState", refs={String.class}, tree="[0]")
private Output provisioningState;
/**
* @return Current provisioning state of the lab.
*
*/
public Output provisioningState() {
return this.provisioningState;
}
/**
* The lab user list management profile.
*
*/
@Export(name="rosterProfile", refs={RosterProfileResponse.class}, tree="[0]")
private Output* @Nullable */ RosterProfileResponse> rosterProfile;
/**
* @return The lab user list management profile.
*
*/
public Output> rosterProfile() {
return Codegen.optional(this.rosterProfile);
}
/**
* The lab security profile.
*
*/
@Export(name="securityProfile", refs={SecurityProfileResponse.class}, tree="[0]")
private Output securityProfile;
/**
* @return The lab security profile.
*
*/
public Output securityProfile() {
return this.securityProfile;
}
/**
* The lab state.
*
*/
@Export(name="state", refs={String.class}, tree="[0]")
private Output state;
/**
* @return The lab state.
*
*/
public Output state() {
return this.state;
}
/**
* Metadata pertaining to creation and last modification of the lab.
*
*/
@Export(name="systemData", refs={SystemDataResponse.class}, tree="[0]")
private Output systemData;
/**
* @return Metadata pertaining to creation and last modification of the lab.
*
*/
public Output systemData() {
return this.systemData;
}
/**
* Resource tags.
*
*/
@Export(name="tags", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> tags;
/**
* @return Resource tags.
*
*/
public Output>> tags() {
return Codegen.optional(this.tags);
}
/**
* The title of the lab.
*
*/
@Export(name="title", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> title;
/**
* @return The title of the lab.
*
*/
public Output> title() {
return Codegen.optional(this.title);
}
/**
* The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
@Export(name="type", refs={String.class}, tree="[0]")
private Output type;
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
public Output type() {
return this.type;
}
/**
* The profile used for creating lab virtual machines.
*
*/
@Export(name="virtualMachineProfile", refs={VirtualMachineProfileResponse.class}, tree="[0]")
private Output virtualMachineProfile;
/**
* @return The profile used for creating lab virtual machines.
*
*/
public Output virtualMachineProfile() {
return this.virtualMachineProfile;
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public Lab(java.lang.String name) {
this(name, LabArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public Lab(java.lang.String name, LabArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public Lab(java.lang.String name, LabArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:labservices:Lab", name, makeArgs(args, options), makeResourceOptions(options, Codegen.empty()), false);
}
private Lab(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:labservices:Lab", name, null, makeResourceOptions(options, id), false);
}
private static LabArgs makeArgs(LabArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
if (options != null && options.getUrn().isPresent()) {
return null;
}
return args == null ? LabArgs.Empty : args;
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.aliases(List.of(
Output.of(Alias.builder().type("azure-native:labservices/v20211001preview:Lab").build()),
Output.of(Alias.builder().type("azure-native:labservices/v20211115preview:Lab").build()),
Output.of(Alias.builder().type("azure-native:labservices/v20220801:Lab").build()),
Output.of(Alias.builder().type("azure-native:labservices/v20230607:Lab").build())
))
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static Lab get(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new Lab(name, id, options);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy