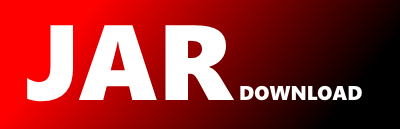
com.pulumi.azurenative.labservices.LabArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.labservices;
import com.pulumi.azurenative.labservices.inputs.AutoShutdownProfileArgs;
import com.pulumi.azurenative.labservices.inputs.ConnectionProfileArgs;
import com.pulumi.azurenative.labservices.inputs.LabNetworkProfileArgs;
import com.pulumi.azurenative.labservices.inputs.RosterProfileArgs;
import com.pulumi.azurenative.labservices.inputs.SecurityProfileArgs;
import com.pulumi.azurenative.labservices.inputs.VirtualMachineProfileArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class LabArgs extends com.pulumi.resources.ResourceArgs {
public static final LabArgs Empty = new LabArgs();
/**
* The resource auto shutdown configuration for the lab. This controls whether actions are taken on resources that are sitting idle.
*
*/
@Import(name="autoShutdownProfile", required=true)
private Output autoShutdownProfile;
/**
* @return The resource auto shutdown configuration for the lab. This controls whether actions are taken on resources that are sitting idle.
*
*/
public Output autoShutdownProfile() {
return this.autoShutdownProfile;
}
/**
* The connection profile for the lab. This controls settings such as web access to lab resources or whether RDP or SSH ports are open.
*
*/
@Import(name="connectionProfile", required=true)
private Output connectionProfile;
/**
* @return The connection profile for the lab. This controls settings such as web access to lab resources or whether RDP or SSH ports are open.
*
*/
public Output connectionProfile() {
return this.connectionProfile;
}
/**
* The description of the lab.
*
*/
@Import(name="description")
private @Nullable Output description;
/**
* @return The description of the lab.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy