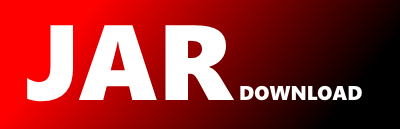
com.pulumi.azurenative.machinelearningservices.outputs.AccountModelResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.machinelearningservices.outputs;
import com.pulumi.azurenative.machinelearningservices.outputs.CallRateLimitResponse;
import com.pulumi.azurenative.machinelearningservices.outputs.DeploymentModelResponse;
import com.pulumi.azurenative.machinelearningservices.outputs.ModelDeprecationInfoResponse;
import com.pulumi.azurenative.machinelearningservices.outputs.ModelSkuResponse;
import com.pulumi.azurenative.machinelearningservices.outputs.SystemDataResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class AccountModelResponse {
/**
* @return Base Model Identifier.
*
*/
private @Nullable DeploymentModelResponse baseModel;
/**
* @return The call rate limit Cognitive Services account.
*
*/
private CallRateLimitResponse callRateLimit;
/**
* @return The capabilities.
*
*/
private @Nullable Map capabilities;
/**
* @return Cognitive Services account ModelDeprecationInfo.
*
*/
private @Nullable ModelDeprecationInfoResponse deprecation;
/**
* @return The capabilities for finetune models.
*
*/
private @Nullable Map finetuneCapabilities;
/**
* @return Deployment model format.
*
*/
private @Nullable String format;
/**
* @return If the model is default version.
*
*/
private @Nullable Boolean isDefaultVersion;
/**
* @return Model lifecycle status.
*
*/
private @Nullable String lifecycleStatus;
/**
* @return The max capacity.
*
*/
private @Nullable Integer maxCapacity;
/**
* @return Deployment model name.
*
*/
private @Nullable String name;
/**
* @return The list of Model Sku.
*
*/
private @Nullable List skus;
/**
* @return Optional. Deployment model source ARM resource ID.
*
*/
private @Nullable String source;
/**
* @return Metadata pertaining to creation and last modification of the resource.
*
*/
private SystemDataResponse systemData;
/**
* @return Optional. Deployment model version. If version is not specified, a default version will be assigned. The default version is different for different models and might change when there is new version available for a model. Default version for a model could be found from list models API.
*
*/
private @Nullable String version;
private AccountModelResponse() {}
/**
* @return Base Model Identifier.
*
*/
public Optional baseModel() {
return Optional.ofNullable(this.baseModel);
}
/**
* @return The call rate limit Cognitive Services account.
*
*/
public CallRateLimitResponse callRateLimit() {
return this.callRateLimit;
}
/**
* @return The capabilities.
*
*/
public Map capabilities() {
return this.capabilities == null ? Map.of() : this.capabilities;
}
/**
* @return Cognitive Services account ModelDeprecationInfo.
*
*/
public Optional deprecation() {
return Optional.ofNullable(this.deprecation);
}
/**
* @return The capabilities for finetune models.
*
*/
public Map finetuneCapabilities() {
return this.finetuneCapabilities == null ? Map.of() : this.finetuneCapabilities;
}
/**
* @return Deployment model format.
*
*/
public Optional format() {
return Optional.ofNullable(this.format);
}
/**
* @return If the model is default version.
*
*/
public Optional isDefaultVersion() {
return Optional.ofNullable(this.isDefaultVersion);
}
/**
* @return Model lifecycle status.
*
*/
public Optional lifecycleStatus() {
return Optional.ofNullable(this.lifecycleStatus);
}
/**
* @return The max capacity.
*
*/
public Optional maxCapacity() {
return Optional.ofNullable(this.maxCapacity);
}
/**
* @return Deployment model name.
*
*/
public Optional name() {
return Optional.ofNullable(this.name);
}
/**
* @return The list of Model Sku.
*
*/
public List skus() {
return this.skus == null ? List.of() : this.skus;
}
/**
* @return Optional. Deployment model source ARM resource ID.
*
*/
public Optional source() {
return Optional.ofNullable(this.source);
}
/**
* @return Metadata pertaining to creation and last modification of the resource.
*
*/
public SystemDataResponse systemData() {
return this.systemData;
}
/**
* @return Optional. Deployment model version. If version is not specified, a default version will be assigned. The default version is different for different models and might change when there is new version available for a model. Default version for a model could be found from list models API.
*
*/
public Optional version() {
return Optional.ofNullable(this.version);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(AccountModelResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable DeploymentModelResponse baseModel;
private CallRateLimitResponse callRateLimit;
private @Nullable Map capabilities;
private @Nullable ModelDeprecationInfoResponse deprecation;
private @Nullable Map finetuneCapabilities;
private @Nullable String format;
private @Nullable Boolean isDefaultVersion;
private @Nullable String lifecycleStatus;
private @Nullable Integer maxCapacity;
private @Nullable String name;
private @Nullable List skus;
private @Nullable String source;
private SystemDataResponse systemData;
private @Nullable String version;
public Builder() {}
public Builder(AccountModelResponse defaults) {
Objects.requireNonNull(defaults);
this.baseModel = defaults.baseModel;
this.callRateLimit = defaults.callRateLimit;
this.capabilities = defaults.capabilities;
this.deprecation = defaults.deprecation;
this.finetuneCapabilities = defaults.finetuneCapabilities;
this.format = defaults.format;
this.isDefaultVersion = defaults.isDefaultVersion;
this.lifecycleStatus = defaults.lifecycleStatus;
this.maxCapacity = defaults.maxCapacity;
this.name = defaults.name;
this.skus = defaults.skus;
this.source = defaults.source;
this.systemData = defaults.systemData;
this.version = defaults.version;
}
@CustomType.Setter
public Builder baseModel(@Nullable DeploymentModelResponse baseModel) {
this.baseModel = baseModel;
return this;
}
@CustomType.Setter
public Builder callRateLimit(CallRateLimitResponse callRateLimit) {
if (callRateLimit == null) {
throw new MissingRequiredPropertyException("AccountModelResponse", "callRateLimit");
}
this.callRateLimit = callRateLimit;
return this;
}
@CustomType.Setter
public Builder capabilities(@Nullable Map capabilities) {
this.capabilities = capabilities;
return this;
}
@CustomType.Setter
public Builder deprecation(@Nullable ModelDeprecationInfoResponse deprecation) {
this.deprecation = deprecation;
return this;
}
@CustomType.Setter
public Builder finetuneCapabilities(@Nullable Map finetuneCapabilities) {
this.finetuneCapabilities = finetuneCapabilities;
return this;
}
@CustomType.Setter
public Builder format(@Nullable String format) {
this.format = format;
return this;
}
@CustomType.Setter
public Builder isDefaultVersion(@Nullable Boolean isDefaultVersion) {
this.isDefaultVersion = isDefaultVersion;
return this;
}
@CustomType.Setter
public Builder lifecycleStatus(@Nullable String lifecycleStatus) {
this.lifecycleStatus = lifecycleStatus;
return this;
}
@CustomType.Setter
public Builder maxCapacity(@Nullable Integer maxCapacity) {
this.maxCapacity = maxCapacity;
return this;
}
@CustomType.Setter
public Builder name(@Nullable String name) {
this.name = name;
return this;
}
@CustomType.Setter
public Builder skus(@Nullable List skus) {
this.skus = skus;
return this;
}
public Builder skus(ModelSkuResponse... skus) {
return skus(List.of(skus));
}
@CustomType.Setter
public Builder source(@Nullable String source) {
this.source = source;
return this;
}
@CustomType.Setter
public Builder systemData(SystemDataResponse systemData) {
if (systemData == null) {
throw new MissingRequiredPropertyException("AccountModelResponse", "systemData");
}
this.systemData = systemData;
return this;
}
@CustomType.Setter
public Builder version(@Nullable String version) {
this.version = version;
return this;
}
public AccountModelResponse build() {
final var _resultValue = new AccountModelResponse();
_resultValue.baseModel = baseModel;
_resultValue.callRateLimit = callRateLimit;
_resultValue.capabilities = capabilities;
_resultValue.deprecation = deprecation;
_resultValue.finetuneCapabilities = finetuneCapabilities;
_resultValue.format = format;
_resultValue.isDefaultVersion = isDefaultVersion;
_resultValue.lifecycleStatus = lifecycleStatus;
_resultValue.maxCapacity = maxCapacity;
_resultValue.name = name;
_resultValue.skus = skus;
_resultValue.source = source;
_resultValue.systemData = systemData;
_resultValue.version = version;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy