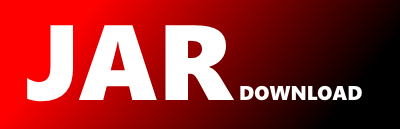
com.pulumi.azurenative.machinelearningservices.outputs.DataContainerResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.machinelearningservices.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class DataContainerResponse {
/**
* @return [Required] Specifies the type of data.
*
*/
private String dataType;
/**
* @return The asset description text.
*
*/
private @Nullable String description;
/**
* @return Is the asset archived?
*
*/
private @Nullable Boolean isArchived;
/**
* @return The latest version inside this container.
*
*/
private String latestVersion;
/**
* @return The next auto incremental version
*
*/
private String nextVersion;
/**
* @return The asset property dictionary.
*
*/
private @Nullable Map properties;
/**
* @return Tag dictionary. Tags can be added, removed, and updated.
*
*/
private @Nullable Map tags;
private DataContainerResponse() {}
/**
* @return [Required] Specifies the type of data.
*
*/
public String dataType() {
return this.dataType;
}
/**
* @return The asset description text.
*
*/
public Optional description() {
return Optional.ofNullable(this.description);
}
/**
* @return Is the asset archived?
*
*/
public Optional isArchived() {
return Optional.ofNullable(this.isArchived);
}
/**
* @return The latest version inside this container.
*
*/
public String latestVersion() {
return this.latestVersion;
}
/**
* @return The next auto incremental version
*
*/
public String nextVersion() {
return this.nextVersion;
}
/**
* @return The asset property dictionary.
*
*/
public Map properties() {
return this.properties == null ? Map.of() : this.properties;
}
/**
* @return Tag dictionary. Tags can be added, removed, and updated.
*
*/
public Map tags() {
return this.tags == null ? Map.of() : this.tags;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(DataContainerResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String dataType;
private @Nullable String description;
private @Nullable Boolean isArchived;
private String latestVersion;
private String nextVersion;
private @Nullable Map properties;
private @Nullable Map tags;
public Builder() {}
public Builder(DataContainerResponse defaults) {
Objects.requireNonNull(defaults);
this.dataType = defaults.dataType;
this.description = defaults.description;
this.isArchived = defaults.isArchived;
this.latestVersion = defaults.latestVersion;
this.nextVersion = defaults.nextVersion;
this.properties = defaults.properties;
this.tags = defaults.tags;
}
@CustomType.Setter
public Builder dataType(String dataType) {
if (dataType == null) {
throw new MissingRequiredPropertyException("DataContainerResponse", "dataType");
}
this.dataType = dataType;
return this;
}
@CustomType.Setter
public Builder description(@Nullable String description) {
this.description = description;
return this;
}
@CustomType.Setter
public Builder isArchived(@Nullable Boolean isArchived) {
this.isArchived = isArchived;
return this;
}
@CustomType.Setter
public Builder latestVersion(String latestVersion) {
if (latestVersion == null) {
throw new MissingRequiredPropertyException("DataContainerResponse", "latestVersion");
}
this.latestVersion = latestVersion;
return this;
}
@CustomType.Setter
public Builder nextVersion(String nextVersion) {
if (nextVersion == null) {
throw new MissingRequiredPropertyException("DataContainerResponse", "nextVersion");
}
this.nextVersion = nextVersion;
return this;
}
@CustomType.Setter
public Builder properties(@Nullable Map properties) {
this.properties = properties;
return this;
}
@CustomType.Setter
public Builder tags(@Nullable Map tags) {
this.tags = tags;
return this;
}
public DataContainerResponse build() {
final var _resultValue = new DataContainerResponse();
_resultValue.dataType = dataType;
_resultValue.description = description;
_resultValue.isArchived = isArchived;
_resultValue.latestVersion = latestVersion;
_resultValue.nextVersion = nextVersion;
_resultValue.properties = properties;
_resultValue.tags = tags;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy