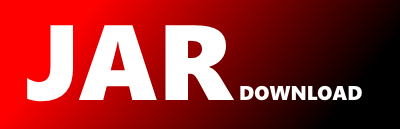
com.pulumi.azurenative.managednetworkfabric.NetworkFabricArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.managednetworkfabric;
import com.pulumi.azurenative.managednetworkfabric.inputs.ManagementNetworkConfigurationArgs;
import com.pulumi.azurenative.managednetworkfabric.inputs.TerminalServerConfigurationArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Integer;
import java.lang.String;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class NetworkFabricArgs extends com.pulumi.resources.ResourceArgs {
public static final NetworkFabricArgs Empty = new NetworkFabricArgs();
/**
* Switch configuration description.
*
*/
@Import(name="annotation")
private @Nullable Output annotation;
/**
* @return Switch configuration description.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy