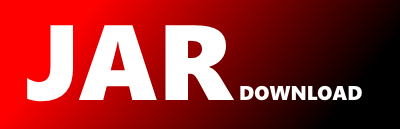
com.pulumi.azurenative.managednetworkfabric.outputs.GetNetworkTapRuleResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.managednetworkfabric.outputs;
import com.pulumi.azurenative.managednetworkfabric.outputs.CommonDynamicMatchConfigurationResponse;
import com.pulumi.azurenative.managednetworkfabric.outputs.NetworkTapRuleMatchConfigurationResponse;
import com.pulumi.azurenative.managednetworkfabric.outputs.SystemDataResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetNetworkTapRuleResult {
/**
* @return Administrative state of the resource.
*
*/
private String administrativeState;
/**
* @return Switch configuration description.
*
*/
private @Nullable String annotation;
/**
* @return Configuration state of the resource.
*
*/
private String configurationState;
/**
* @return Input method to configure Network Tap Rule.
*
*/
private String configurationType;
/**
* @return List of dynamic match configurations.
*
*/
private @Nullable List dynamicMatchConfigurations;
/**
* @return Fully qualified resource ID for the resource. E.g. "/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}"
*
*/
private String id;
/**
* @return The last sync timestamp.
*
*/
private String lastSyncedTime;
/**
* @return The geo-location where the resource lives
*
*/
private String location;
/**
* @return List of match configurations.
*
*/
private @Nullable List matchConfigurations;
/**
* @return The name of the resource
*
*/
private String name;
/**
* @return The ARM resource Id of the NetworkTap.
*
*/
private String networkTapId;
/**
* @return Polling interval in seconds.
*
*/
private @Nullable Integer pollingIntervalInSeconds;
/**
* @return Provisioning state of the resource.
*
*/
private String provisioningState;
/**
* @return Azure Resource Manager metadata containing createdBy and modifiedBy information.
*
*/
private SystemDataResponse systemData;
/**
* @return Resource tags.
*
*/
private @Nullable Map tags;
/**
* @return Network Tap Rules file URL.
*
*/
private @Nullable String tapRulesUrl;
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
private String type;
private GetNetworkTapRuleResult() {}
/**
* @return Administrative state of the resource.
*
*/
public String administrativeState() {
return this.administrativeState;
}
/**
* @return Switch configuration description.
*
*/
public Optional annotation() {
return Optional.ofNullable(this.annotation);
}
/**
* @return Configuration state of the resource.
*
*/
public String configurationState() {
return this.configurationState;
}
/**
* @return Input method to configure Network Tap Rule.
*
*/
public String configurationType() {
return this.configurationType;
}
/**
* @return List of dynamic match configurations.
*
*/
public List dynamicMatchConfigurations() {
return this.dynamicMatchConfigurations == null ? List.of() : this.dynamicMatchConfigurations;
}
/**
* @return Fully qualified resource ID for the resource. E.g. "/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}"
*
*/
public String id() {
return this.id;
}
/**
* @return The last sync timestamp.
*
*/
public String lastSyncedTime() {
return this.lastSyncedTime;
}
/**
* @return The geo-location where the resource lives
*
*/
public String location() {
return this.location;
}
/**
* @return List of match configurations.
*
*/
public List matchConfigurations() {
return this.matchConfigurations == null ? List.of() : this.matchConfigurations;
}
/**
* @return The name of the resource
*
*/
public String name() {
return this.name;
}
/**
* @return The ARM resource Id of the NetworkTap.
*
*/
public String networkTapId() {
return this.networkTapId;
}
/**
* @return Polling interval in seconds.
*
*/
public Optional pollingIntervalInSeconds() {
return Optional.ofNullable(this.pollingIntervalInSeconds);
}
/**
* @return Provisioning state of the resource.
*
*/
public String provisioningState() {
return this.provisioningState;
}
/**
* @return Azure Resource Manager metadata containing createdBy and modifiedBy information.
*
*/
public SystemDataResponse systemData() {
return this.systemData;
}
/**
* @return Resource tags.
*
*/
public Map tags() {
return this.tags == null ? Map.of() : this.tags;
}
/**
* @return Network Tap Rules file URL.
*
*/
public Optional tapRulesUrl() {
return Optional.ofNullable(this.tapRulesUrl);
}
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
public String type() {
return this.type;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetNetworkTapRuleResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String administrativeState;
private @Nullable String annotation;
private String configurationState;
private String configurationType;
private @Nullable List dynamicMatchConfigurations;
private String id;
private String lastSyncedTime;
private String location;
private @Nullable List matchConfigurations;
private String name;
private String networkTapId;
private @Nullable Integer pollingIntervalInSeconds;
private String provisioningState;
private SystemDataResponse systemData;
private @Nullable Map tags;
private @Nullable String tapRulesUrl;
private String type;
public Builder() {}
public Builder(GetNetworkTapRuleResult defaults) {
Objects.requireNonNull(defaults);
this.administrativeState = defaults.administrativeState;
this.annotation = defaults.annotation;
this.configurationState = defaults.configurationState;
this.configurationType = defaults.configurationType;
this.dynamicMatchConfigurations = defaults.dynamicMatchConfigurations;
this.id = defaults.id;
this.lastSyncedTime = defaults.lastSyncedTime;
this.location = defaults.location;
this.matchConfigurations = defaults.matchConfigurations;
this.name = defaults.name;
this.networkTapId = defaults.networkTapId;
this.pollingIntervalInSeconds = defaults.pollingIntervalInSeconds;
this.provisioningState = defaults.provisioningState;
this.systemData = defaults.systemData;
this.tags = defaults.tags;
this.tapRulesUrl = defaults.tapRulesUrl;
this.type = defaults.type;
}
@CustomType.Setter
public Builder administrativeState(String administrativeState) {
if (administrativeState == null) {
throw new MissingRequiredPropertyException("GetNetworkTapRuleResult", "administrativeState");
}
this.administrativeState = administrativeState;
return this;
}
@CustomType.Setter
public Builder annotation(@Nullable String annotation) {
this.annotation = annotation;
return this;
}
@CustomType.Setter
public Builder configurationState(String configurationState) {
if (configurationState == null) {
throw new MissingRequiredPropertyException("GetNetworkTapRuleResult", "configurationState");
}
this.configurationState = configurationState;
return this;
}
@CustomType.Setter
public Builder configurationType(String configurationType) {
if (configurationType == null) {
throw new MissingRequiredPropertyException("GetNetworkTapRuleResult", "configurationType");
}
this.configurationType = configurationType;
return this;
}
@CustomType.Setter
public Builder dynamicMatchConfigurations(@Nullable List dynamicMatchConfigurations) {
this.dynamicMatchConfigurations = dynamicMatchConfigurations;
return this;
}
public Builder dynamicMatchConfigurations(CommonDynamicMatchConfigurationResponse... dynamicMatchConfigurations) {
return dynamicMatchConfigurations(List.of(dynamicMatchConfigurations));
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetNetworkTapRuleResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder lastSyncedTime(String lastSyncedTime) {
if (lastSyncedTime == null) {
throw new MissingRequiredPropertyException("GetNetworkTapRuleResult", "lastSyncedTime");
}
this.lastSyncedTime = lastSyncedTime;
return this;
}
@CustomType.Setter
public Builder location(String location) {
if (location == null) {
throw new MissingRequiredPropertyException("GetNetworkTapRuleResult", "location");
}
this.location = location;
return this;
}
@CustomType.Setter
public Builder matchConfigurations(@Nullable List matchConfigurations) {
this.matchConfigurations = matchConfigurations;
return this;
}
public Builder matchConfigurations(NetworkTapRuleMatchConfigurationResponse... matchConfigurations) {
return matchConfigurations(List.of(matchConfigurations));
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetNetworkTapRuleResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder networkTapId(String networkTapId) {
if (networkTapId == null) {
throw new MissingRequiredPropertyException("GetNetworkTapRuleResult", "networkTapId");
}
this.networkTapId = networkTapId;
return this;
}
@CustomType.Setter
public Builder pollingIntervalInSeconds(@Nullable Integer pollingIntervalInSeconds) {
this.pollingIntervalInSeconds = pollingIntervalInSeconds;
return this;
}
@CustomType.Setter
public Builder provisioningState(String provisioningState) {
if (provisioningState == null) {
throw new MissingRequiredPropertyException("GetNetworkTapRuleResult", "provisioningState");
}
this.provisioningState = provisioningState;
return this;
}
@CustomType.Setter
public Builder systemData(SystemDataResponse systemData) {
if (systemData == null) {
throw new MissingRequiredPropertyException("GetNetworkTapRuleResult", "systemData");
}
this.systemData = systemData;
return this;
}
@CustomType.Setter
public Builder tags(@Nullable Map tags) {
this.tags = tags;
return this;
}
@CustomType.Setter
public Builder tapRulesUrl(@Nullable String tapRulesUrl) {
this.tapRulesUrl = tapRulesUrl;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetNetworkTapRuleResult", "type");
}
this.type = type;
return this;
}
public GetNetworkTapRuleResult build() {
final var _resultValue = new GetNetworkTapRuleResult();
_resultValue.administrativeState = administrativeState;
_resultValue.annotation = annotation;
_resultValue.configurationState = configurationState;
_resultValue.configurationType = configurationType;
_resultValue.dynamicMatchConfigurations = dynamicMatchConfigurations;
_resultValue.id = id;
_resultValue.lastSyncedTime = lastSyncedTime;
_resultValue.location = location;
_resultValue.matchConfigurations = matchConfigurations;
_resultValue.name = name;
_resultValue.networkTapId = networkTapId;
_resultValue.pollingIntervalInSeconds = pollingIntervalInSeconds;
_resultValue.provisioningState = provisioningState;
_resultValue.systemData = systemData;
_resultValue.tags = tags;
_resultValue.tapRulesUrl = tapRulesUrl;
_resultValue.type = type;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy