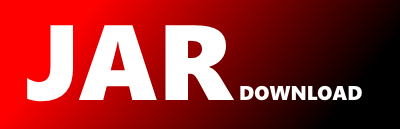
com.pulumi.azurenative.management.ManagementFunctions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.management;
import com.pulumi.azurenative.Utilities;
import com.pulumi.azurenative.management.inputs.GetEntityArgs;
import com.pulumi.azurenative.management.inputs.GetEntityPlainArgs;
import com.pulumi.azurenative.management.inputs.GetHierarchySettingArgs;
import com.pulumi.azurenative.management.inputs.GetHierarchySettingPlainArgs;
import com.pulumi.azurenative.management.inputs.GetManagementGroupArgs;
import com.pulumi.azurenative.management.inputs.GetManagementGroupPlainArgs;
import com.pulumi.azurenative.management.inputs.GetManagementGroupSubscriptionArgs;
import com.pulumi.azurenative.management.inputs.GetManagementGroupSubscriptionPlainArgs;
import com.pulumi.azurenative.management.outputs.GetEntityResult;
import com.pulumi.azurenative.management.outputs.GetHierarchySettingResult;
import com.pulumi.azurenative.management.outputs.GetManagementGroupResult;
import com.pulumi.azurenative.management.outputs.GetManagementGroupSubscriptionResult;
import com.pulumi.core.Output;
import com.pulumi.core.TypeShape;
import com.pulumi.deployment.Deployment;
import com.pulumi.deployment.InvokeOptions;
import java.util.concurrent.CompletableFuture;
public final class ManagementFunctions {
/**
* List all entities (Management Groups, Subscriptions, etc.) for the authenticated user.
*
* Azure REST API version: 2021-04-01.
*
* Other available API versions: 2018-01-01-preview, 2018-03-01-preview, 2019-11-01, 2020-02-01, 2020-05-01, 2020-10-01, 2023-04-01.
*
*/
public static Output getEntity() {
return getEntity(GetEntityArgs.Empty, InvokeOptions.Empty);
}
/**
* List all entities (Management Groups, Subscriptions, etc.) for the authenticated user.
*
* Azure REST API version: 2021-04-01.
*
* Other available API versions: 2018-01-01-preview, 2018-03-01-preview, 2019-11-01, 2020-02-01, 2020-05-01, 2020-10-01, 2023-04-01.
*
*/
public static CompletableFuture getEntityPlain() {
return getEntityPlain(GetEntityPlainArgs.Empty, InvokeOptions.Empty);
}
/**
* List all entities (Management Groups, Subscriptions, etc.) for the authenticated user.
*
* Azure REST API version: 2021-04-01.
*
* Other available API versions: 2018-01-01-preview, 2018-03-01-preview, 2019-11-01, 2020-02-01, 2020-05-01, 2020-10-01, 2023-04-01.
*
*/
public static Output getEntity(GetEntityArgs args) {
return getEntity(args, InvokeOptions.Empty);
}
/**
* List all entities (Management Groups, Subscriptions, etc.) for the authenticated user.
*
* Azure REST API version: 2021-04-01.
*
* Other available API versions: 2018-01-01-preview, 2018-03-01-preview, 2019-11-01, 2020-02-01, 2020-05-01, 2020-10-01, 2023-04-01.
*
*/
public static CompletableFuture getEntityPlain(GetEntityPlainArgs args) {
return getEntityPlain(args, InvokeOptions.Empty);
}
/**
* List all entities (Management Groups, Subscriptions, etc.) for the authenticated user.
*
* Azure REST API version: 2021-04-01.
*
* Other available API versions: 2018-01-01-preview, 2018-03-01-preview, 2019-11-01, 2020-02-01, 2020-05-01, 2020-10-01, 2023-04-01.
*
*/
public static Output getEntity(GetEntityArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:management:getEntity", TypeShape.of(GetEntityResult.class), args, Utilities.withVersion(options));
}
/**
* List all entities (Management Groups, Subscriptions, etc.) for the authenticated user.
*
* Azure REST API version: 2021-04-01.
*
* Other available API versions: 2018-01-01-preview, 2018-03-01-preview, 2019-11-01, 2020-02-01, 2020-05-01, 2020-10-01, 2023-04-01.
*
*/
public static CompletableFuture getEntityPlain(GetEntityPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:management:getEntity", TypeShape.of(GetEntityResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the hierarchy settings defined at the Management Group level. Settings can only be set on the root Management Group of the hierarchy.
*
* Azure REST API version: 2021-04-01.
*
* Other available API versions: 2023-04-01.
*
*/
public static Output getHierarchySetting(GetHierarchySettingArgs args) {
return getHierarchySetting(args, InvokeOptions.Empty);
}
/**
* Gets the hierarchy settings defined at the Management Group level. Settings can only be set on the root Management Group of the hierarchy.
*
* Azure REST API version: 2021-04-01.
*
* Other available API versions: 2023-04-01.
*
*/
public static CompletableFuture getHierarchySettingPlain(GetHierarchySettingPlainArgs args) {
return getHierarchySettingPlain(args, InvokeOptions.Empty);
}
/**
* Gets the hierarchy settings defined at the Management Group level. Settings can only be set on the root Management Group of the hierarchy.
*
* Azure REST API version: 2021-04-01.
*
* Other available API versions: 2023-04-01.
*
*/
public static Output getHierarchySetting(GetHierarchySettingArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:management:getHierarchySetting", TypeShape.of(GetHierarchySettingResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the hierarchy settings defined at the Management Group level. Settings can only be set on the root Management Group of the hierarchy.
*
* Azure REST API version: 2021-04-01.
*
* Other available API versions: 2023-04-01.
*
*/
public static CompletableFuture getHierarchySettingPlain(GetHierarchySettingPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:management:getHierarchySetting", TypeShape.of(GetHierarchySettingResult.class), args, Utilities.withVersion(options));
}
/**
* Get the details of the management group.
*
* Azure REST API version: 2021-04-01.
*
* Other available API versions: 2017-11-01-preview, 2020-02-01, 2023-04-01.
*
*/
public static Output getManagementGroup(GetManagementGroupArgs args) {
return getManagementGroup(args, InvokeOptions.Empty);
}
/**
* Get the details of the management group.
*
* Azure REST API version: 2021-04-01.
*
* Other available API versions: 2017-11-01-preview, 2020-02-01, 2023-04-01.
*
*/
public static CompletableFuture getManagementGroupPlain(GetManagementGroupPlainArgs args) {
return getManagementGroupPlain(args, InvokeOptions.Empty);
}
/**
* Get the details of the management group.
*
* Azure REST API version: 2021-04-01.
*
* Other available API versions: 2017-11-01-preview, 2020-02-01, 2023-04-01.
*
*/
public static Output getManagementGroup(GetManagementGroupArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:management:getManagementGroup", TypeShape.of(GetManagementGroupResult.class), args, Utilities.withVersion(options));
}
/**
* Get the details of the management group.
*
* Azure REST API version: 2021-04-01.
*
* Other available API versions: 2017-11-01-preview, 2020-02-01, 2023-04-01.
*
*/
public static CompletableFuture getManagementGroupPlain(GetManagementGroupPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:management:getManagementGroup", TypeShape.of(GetManagementGroupResult.class), args, Utilities.withVersion(options));
}
/**
* Retrieves details about given subscription which is associated with the management group.
*
* Azure REST API version: 2021-04-01.
*
* Other available API versions: 2023-04-01.
*
*/
public static Output getManagementGroupSubscription(GetManagementGroupSubscriptionArgs args) {
return getManagementGroupSubscription(args, InvokeOptions.Empty);
}
/**
* Retrieves details about given subscription which is associated with the management group.
*
* Azure REST API version: 2021-04-01.
*
* Other available API versions: 2023-04-01.
*
*/
public static CompletableFuture getManagementGroupSubscriptionPlain(GetManagementGroupSubscriptionPlainArgs args) {
return getManagementGroupSubscriptionPlain(args, InvokeOptions.Empty);
}
/**
* Retrieves details about given subscription which is associated with the management group.
*
* Azure REST API version: 2021-04-01.
*
* Other available API versions: 2023-04-01.
*
*/
public static Output getManagementGroupSubscription(GetManagementGroupSubscriptionArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:management:getManagementGroupSubscription", TypeShape.of(GetManagementGroupSubscriptionResult.class), args, Utilities.withVersion(options));
}
/**
* Retrieves details about given subscription which is associated with the management group.
*
* Azure REST API version: 2021-04-01.
*
* Other available API versions: 2023-04-01.
*
*/
public static CompletableFuture getManagementGroupSubscriptionPlain(GetManagementGroupSubscriptionPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:management:getManagementGroupSubscription", TypeShape.of(GetManagementGroupSubscriptionResult.class), args, Utilities.withVersion(options));
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy