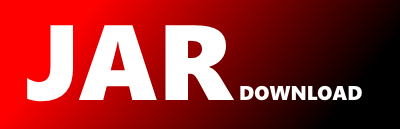
com.pulumi.azurenative.management.inputs.GetEntityPlainArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.management.inputs;
import com.pulumi.core.annotations.Import;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class GetEntityPlainArgs extends com.pulumi.resources.InvokeArgs {
public static final GetEntityPlainArgs Empty = new GetEntityPlainArgs();
/**
* The filter parameter allows you to filter on the the name or display name fields. You can check for equality on the name field (e.g. name eq '{entityName}') and you can check for substrings on either the name or display name fields(e.g. contains(name, '{substringToSearch}'), contains(displayName, '{substringToSearch')). Note that the '{entityName}' and '{substringToSearch}' fields are checked case insensitively.
*
*/
@Import(name="filter")
private @Nullable String filter;
/**
* @return The filter parameter allows you to filter on the the name or display name fields. You can check for equality on the name field (e.g. name eq '{entityName}') and you can check for substrings on either the name or display name fields(e.g. contains(name, '{substringToSearch}'), contains(displayName, '{substringToSearch')). Note that the '{entityName}' and '{substringToSearch}' fields are checked case insensitively.
*
*/
public Optional filter() {
return Optional.ofNullable(this.filter);
}
/**
* A filter which allows the get entities call to focus on a particular group (i.e. "$filter=name eq 'groupName'")
*
*/
@Import(name="groupName")
private @Nullable String groupName;
/**
* @return A filter which allows the get entities call to focus on a particular group (i.e. "$filter=name eq 'groupName'")
*
*/
public Optional groupName() {
return Optional.ofNullable(this.groupName);
}
/**
* The $search parameter is used in conjunction with the $filter parameter to return three different outputs depending on the parameter passed in.
* With $search=AllowedParents the API will return the entity info of all groups that the requested entity will be able to reparent to as determined by the user's permissions.
* With $search=AllowedChildren the API will return the entity info of all entities that can be added as children of the requested entity.
* With $search=ParentAndFirstLevelChildren the API will return the parent and first level of children that the user has either direct access to or indirect access via one of their descendants.
* With $search=ParentOnly the API will return only the group if the user has access to at least one of the descendants of the group.
* With $search=ChildrenOnly the API will return only the first level of children of the group entity info specified in $filter. The user must have direct access to the children entities or one of it's descendants for it to show up in the results.
*
*/
@Import(name="search")
private @Nullable String search;
/**
* @return The $search parameter is used in conjunction with the $filter parameter to return three different outputs depending on the parameter passed in.
* With $search=AllowedParents the API will return the entity info of all groups that the requested entity will be able to reparent to as determined by the user's permissions.
* With $search=AllowedChildren the API will return the entity info of all entities that can be added as children of the requested entity.
* With $search=ParentAndFirstLevelChildren the API will return the parent and first level of children that the user has either direct access to or indirect access via one of their descendants.
* With $search=ParentOnly the API will return only the group if the user has access to at least one of the descendants of the group.
* With $search=ChildrenOnly the API will return only the first level of children of the group entity info specified in $filter. The user must have direct access to the children entities or one of it's descendants for it to show up in the results.
*
*/
public Optional search() {
return Optional.ofNullable(this.search);
}
/**
* This parameter specifies the fields to include in the response. Can include any combination of Name,DisplayName,Type,ParentDisplayNameChain,ParentChain, e.g. '$select=Name,DisplayName,Type,ParentDisplayNameChain,ParentNameChain'. When specified the $select parameter can override select in $skipToken.
*
*/
@Import(name="select")
private @Nullable String select;
/**
* @return This parameter specifies the fields to include in the response. Can include any combination of Name,DisplayName,Type,ParentDisplayNameChain,ParentChain, e.g. '$select=Name,DisplayName,Type,ParentDisplayNameChain,ParentNameChain'. When specified the $select parameter can override select in $skipToken.
*
*/
public Optional select() {
return Optional.ofNullable(this.select);
}
/**
* Number of entities to skip over when retrieving results. Passing this in will override $skipToken.
*
*/
@Import(name="skip")
private @Nullable Integer skip;
/**
* @return Number of entities to skip over when retrieving results. Passing this in will override $skipToken.
*
*/
public Optional skip() {
return Optional.ofNullable(this.skip);
}
/**
* Page continuation token is only used if a previous operation returned a partial result.
* If a previous response contains a nextLink element, the value of the nextLink element will include a token parameter that specifies a starting point to use for subsequent calls.
*
*/
@Import(name="skiptoken")
private @Nullable String skiptoken;
/**
* @return Page continuation token is only used if a previous operation returned a partial result.
* If a previous response contains a nextLink element, the value of the nextLink element will include a token parameter that specifies a starting point to use for subsequent calls.
*
*/
public Optional skiptoken() {
return Optional.ofNullable(this.skiptoken);
}
/**
* Number of elements to return when retrieving results. Passing this in will override $skipToken.
*
*/
@Import(name="top")
private @Nullable Integer top;
/**
* @return Number of elements to return when retrieving results. Passing this in will override $skipToken.
*
*/
public Optional top() {
return Optional.ofNullable(this.top);
}
/**
* The view parameter allows clients to filter the type of data that is returned by the getEntities call.
*
*/
@Import(name="view")
private @Nullable String view;
/**
* @return The view parameter allows clients to filter the type of data that is returned by the getEntities call.
*
*/
public Optional view() {
return Optional.ofNullable(this.view);
}
private GetEntityPlainArgs() {}
private GetEntityPlainArgs(GetEntityPlainArgs $) {
this.filter = $.filter;
this.groupName = $.groupName;
this.search = $.search;
this.select = $.select;
this.skip = $.skip;
this.skiptoken = $.skiptoken;
this.top = $.top;
this.view = $.view;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetEntityPlainArgs defaults) {
return new Builder(defaults);
}
public static final class Builder {
private GetEntityPlainArgs $;
public Builder() {
$ = new GetEntityPlainArgs();
}
public Builder(GetEntityPlainArgs defaults) {
$ = new GetEntityPlainArgs(Objects.requireNonNull(defaults));
}
/**
* @param filter The filter parameter allows you to filter on the the name or display name fields. You can check for equality on the name field (e.g. name eq '{entityName}') and you can check for substrings on either the name or display name fields(e.g. contains(name, '{substringToSearch}'), contains(displayName, '{substringToSearch')). Note that the '{entityName}' and '{substringToSearch}' fields are checked case insensitively.
*
* @return builder
*
*/
public Builder filter(@Nullable String filter) {
$.filter = filter;
return this;
}
/**
* @param groupName A filter which allows the get entities call to focus on a particular group (i.e. "$filter=name eq 'groupName'")
*
* @return builder
*
*/
public Builder groupName(@Nullable String groupName) {
$.groupName = groupName;
return this;
}
/**
* @param search The $search parameter is used in conjunction with the $filter parameter to return three different outputs depending on the parameter passed in.
* With $search=AllowedParents the API will return the entity info of all groups that the requested entity will be able to reparent to as determined by the user's permissions.
* With $search=AllowedChildren the API will return the entity info of all entities that can be added as children of the requested entity.
* With $search=ParentAndFirstLevelChildren the API will return the parent and first level of children that the user has either direct access to or indirect access via one of their descendants.
* With $search=ParentOnly the API will return only the group if the user has access to at least one of the descendants of the group.
* With $search=ChildrenOnly the API will return only the first level of children of the group entity info specified in $filter. The user must have direct access to the children entities or one of it's descendants for it to show up in the results.
*
* @return builder
*
*/
public Builder search(@Nullable String search) {
$.search = search;
return this;
}
/**
* @param select This parameter specifies the fields to include in the response. Can include any combination of Name,DisplayName,Type,ParentDisplayNameChain,ParentChain, e.g. '$select=Name,DisplayName,Type,ParentDisplayNameChain,ParentNameChain'. When specified the $select parameter can override select in $skipToken.
*
* @return builder
*
*/
public Builder select(@Nullable String select) {
$.select = select;
return this;
}
/**
* @param skip Number of entities to skip over when retrieving results. Passing this in will override $skipToken.
*
* @return builder
*
*/
public Builder skip(@Nullable Integer skip) {
$.skip = skip;
return this;
}
/**
* @param skiptoken Page continuation token is only used if a previous operation returned a partial result.
* If a previous response contains a nextLink element, the value of the nextLink element will include a token parameter that specifies a starting point to use for subsequent calls.
*
* @return builder
*
*/
public Builder skiptoken(@Nullable String skiptoken) {
$.skiptoken = skiptoken;
return this;
}
/**
* @param top Number of elements to return when retrieving results. Passing this in will override $skipToken.
*
* @return builder
*
*/
public Builder top(@Nullable Integer top) {
$.top = top;
return this;
}
/**
* @param view The view parameter allows clients to filter the type of data that is returned by the getEntities call.
*
* @return builder
*
*/
public Builder view(@Nullable String view) {
$.view = view;
return this;
}
public GetEntityPlainArgs build() {
return $;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy