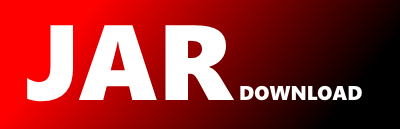
com.pulumi.azurenative.media.LiveOutputArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.media;
import com.pulumi.azurenative.media.inputs.HlsArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Double;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class LiveOutputArgs extends com.pulumi.resources.ResourceArgs {
public static final LiveOutputArgs Empty = new LiveOutputArgs();
/**
* The Media Services account name.
*
*/
@Import(name="accountName", required=true)
private Output accountName;
/**
* @return The Media Services account name.
*
*/
public Output accountName() {
return this.accountName;
}
/**
* ISO 8601 time between 1 minute to 25 hours to indicate the maximum content length that can be archived in the asset for this live output. This also sets the maximum content length for the rewind window. For example, use PT1H30M to indicate 1 hour and 30 minutes of archive window.
*
*/
@Import(name="archiveWindowLength", required=true)
private Output archiveWindowLength;
/**
* @return ISO 8601 time between 1 minute to 25 hours to indicate the maximum content length that can be archived in the asset for this live output. This also sets the maximum content length for the rewind window. For example, use PT1H30M to indicate 1 hour and 30 minutes of archive window.
*
*/
public Output archiveWindowLength() {
return this.archiveWindowLength;
}
/**
* The asset that the live output will write to.
*
*/
@Import(name="assetName", required=true)
private Output assetName;
/**
* @return The asset that the live output will write to.
*
*/
public Output assetName() {
return this.assetName;
}
/**
* The description of the live output.
*
*/
@Import(name="description")
private @Nullable Output description;
/**
* @return The description of the live output.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy