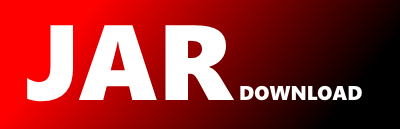
com.pulumi.azurenative.media.inputs.TransformOutputArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.media.inputs;
import com.pulumi.azurenative.media.enums.OnErrorType;
import com.pulumi.azurenative.media.enums.Priority;
import com.pulumi.azurenative.media.inputs.AudioAnalyzerPresetArgs;
import com.pulumi.azurenative.media.inputs.BuiltInStandardEncoderPresetArgs;
import com.pulumi.azurenative.media.inputs.FaceDetectorPresetArgs;
import com.pulumi.azurenative.media.inputs.StandardEncoderPresetArgs;
import com.pulumi.azurenative.media.inputs.VideoAnalyzerPresetArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Object;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Describes the properties of a TransformOutput, which are the rules to be applied while generating the desired output.
*
*/
public final class TransformOutputArgs extends com.pulumi.resources.ResourceArgs {
public static final TransformOutputArgs Empty = new TransformOutputArgs();
/**
* A Transform can define more than one outputs. This property defines what the service should do when one output fails - either continue to produce other outputs, or, stop the other outputs. The overall Job state will not reflect failures of outputs that are specified with 'ContinueJob'. The default is 'StopProcessingJob'.
*
*/
@Import(name="onError")
private @Nullable Output> onError;
/**
* @return A Transform can define more than one outputs. This property defines what the service should do when one output fails - either continue to produce other outputs, or, stop the other outputs. The overall Job state will not reflect failures of outputs that are specified with 'ContinueJob'. The default is 'StopProcessingJob'.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy