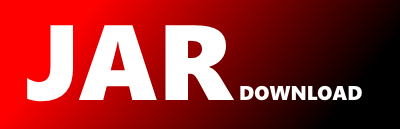
com.pulumi.azurenative.media.outputs.LiveEventStreamEventDataResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.media.outputs;
import com.pulumi.core.annotations.CustomType;
import java.lang.Double;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class LiveEventStreamEventDataResponse {
/**
* @return Bitrate of the track.
*
*/
private @Nullable Double bitrate;
/**
* @return Current fragment timestamp in timescale.
*
*/
private @Nullable String currentFragmentTimestamp;
/**
* @return Length of the discontinuity gap in timescale.
*
*/
private @Nullable Double discontinuityGap;
/**
* @return Fragment duration.
*
*/
private @Nullable String duration;
/**
* @return Reason the fragment was dropped.
*
*/
private @Nullable String fragmentDropReason;
/**
* @return Duration of first fragment used to make a comparison, in timescale.
*
*/
private @Nullable String fragmentOneDuration;
/**
* @return Timestamp of first fragment used to make a comparison, in timescale.
*
*/
private @Nullable String fragmentOneTimestamp;
/**
* @return Duration of second fragment used to make a comparison, in timescale.
*
*/
private @Nullable String fragmentTwoDuration;
/**
* @return Timestamp of second fragment used to make a comparison, in timescale.
*
*/
private @Nullable String fragmentTwoTimestamp;
/**
* @return The larger timestamp of the two fragments compared.
*
*/
private @Nullable String maxTime;
/**
* @return The media type of the larger timestamp of two fragments compared.
*
*/
private @Nullable String maxTimeMediaType;
/**
* @return Fragment timestamp in timescale.
*
*/
private @Nullable String mediaTimestamp;
/**
* @return Type of the track.
*
*/
private @Nullable String mediaType;
/**
* @return The smaller timestamp of the two fragments compared.
*
*/
private @Nullable String minTime;
/**
* @return The media type of the smaller timestamp of two fragments compared.
*
*/
private @Nullable String minTimeMediaType;
/**
* @return Previous fragment duration in timescale.
*
*/
private @Nullable String previousFragmentDuration;
/**
* @return Previous fragment timestamp in timescale.
*
*/
private @Nullable String previousFragmentTimestamp;
/**
* @return Truncated IP of the encoder.
*
*/
private @Nullable String remoteIp;
/**
* @return Port of the encoder.
*
*/
private @Nullable String remotePort;
/**
* @return Width x Height for video, null otherwise.
*
*/
private @Nullable String resolution;
/**
* @return Result code.
*
*/
private @Nullable String resultCode;
/**
* @return Result message.
*
*/
private @Nullable String resultMessage;
/**
* @return Stream ID in the format "trackName_bitrate"
*
*/
private @Nullable String streamId;
/**
* @return Identifier of the stream or connection. Encoder or customer is responsible to add this ID in the ingest URL.
*
*/
private @Nullable String streamName;
/**
* @return Timescale in which timestamps are expressed.
*
*/
private @Nullable String timescale;
/**
* @return Timescale of the fragment with the larger timestamp.
*
*/
private @Nullable String timescaleOfMaxTime;
/**
* @return Timescale of the fragment with the smaller timestamp.
*
*/
private @Nullable String timescaleOfMinTime;
/**
* @return Track index.
*
*/
private @Nullable Integer trackId;
/**
* @return Name of the track.
*
*/
private @Nullable String trackName;
private LiveEventStreamEventDataResponse() {}
/**
* @return Bitrate of the track.
*
*/
public Optional bitrate() {
return Optional.ofNullable(this.bitrate);
}
/**
* @return Current fragment timestamp in timescale.
*
*/
public Optional currentFragmentTimestamp() {
return Optional.ofNullable(this.currentFragmentTimestamp);
}
/**
* @return Length of the discontinuity gap in timescale.
*
*/
public Optional discontinuityGap() {
return Optional.ofNullable(this.discontinuityGap);
}
/**
* @return Fragment duration.
*
*/
public Optional duration() {
return Optional.ofNullable(this.duration);
}
/**
* @return Reason the fragment was dropped.
*
*/
public Optional fragmentDropReason() {
return Optional.ofNullable(this.fragmentDropReason);
}
/**
* @return Duration of first fragment used to make a comparison, in timescale.
*
*/
public Optional fragmentOneDuration() {
return Optional.ofNullable(this.fragmentOneDuration);
}
/**
* @return Timestamp of first fragment used to make a comparison, in timescale.
*
*/
public Optional fragmentOneTimestamp() {
return Optional.ofNullable(this.fragmentOneTimestamp);
}
/**
* @return Duration of second fragment used to make a comparison, in timescale.
*
*/
public Optional fragmentTwoDuration() {
return Optional.ofNullable(this.fragmentTwoDuration);
}
/**
* @return Timestamp of second fragment used to make a comparison, in timescale.
*
*/
public Optional fragmentTwoTimestamp() {
return Optional.ofNullable(this.fragmentTwoTimestamp);
}
/**
* @return The larger timestamp of the two fragments compared.
*
*/
public Optional maxTime() {
return Optional.ofNullable(this.maxTime);
}
/**
* @return The media type of the larger timestamp of two fragments compared.
*
*/
public Optional maxTimeMediaType() {
return Optional.ofNullable(this.maxTimeMediaType);
}
/**
* @return Fragment timestamp in timescale.
*
*/
public Optional mediaTimestamp() {
return Optional.ofNullable(this.mediaTimestamp);
}
/**
* @return Type of the track.
*
*/
public Optional mediaType() {
return Optional.ofNullable(this.mediaType);
}
/**
* @return The smaller timestamp of the two fragments compared.
*
*/
public Optional minTime() {
return Optional.ofNullable(this.minTime);
}
/**
* @return The media type of the smaller timestamp of two fragments compared.
*
*/
public Optional minTimeMediaType() {
return Optional.ofNullable(this.minTimeMediaType);
}
/**
* @return Previous fragment duration in timescale.
*
*/
public Optional previousFragmentDuration() {
return Optional.ofNullable(this.previousFragmentDuration);
}
/**
* @return Previous fragment timestamp in timescale.
*
*/
public Optional previousFragmentTimestamp() {
return Optional.ofNullable(this.previousFragmentTimestamp);
}
/**
* @return Truncated IP of the encoder.
*
*/
public Optional remoteIp() {
return Optional.ofNullable(this.remoteIp);
}
/**
* @return Port of the encoder.
*
*/
public Optional remotePort() {
return Optional.ofNullable(this.remotePort);
}
/**
* @return Width x Height for video, null otherwise.
*
*/
public Optional resolution() {
return Optional.ofNullable(this.resolution);
}
/**
* @return Result code.
*
*/
public Optional resultCode() {
return Optional.ofNullable(this.resultCode);
}
/**
* @return Result message.
*
*/
public Optional resultMessage() {
return Optional.ofNullable(this.resultMessage);
}
/**
* @return Stream ID in the format "trackName_bitrate"
*
*/
public Optional streamId() {
return Optional.ofNullable(this.streamId);
}
/**
* @return Identifier of the stream or connection. Encoder or customer is responsible to add this ID in the ingest URL.
*
*/
public Optional streamName() {
return Optional.ofNullable(this.streamName);
}
/**
* @return Timescale in which timestamps are expressed.
*
*/
public Optional timescale() {
return Optional.ofNullable(this.timescale);
}
/**
* @return Timescale of the fragment with the larger timestamp.
*
*/
public Optional timescaleOfMaxTime() {
return Optional.ofNullable(this.timescaleOfMaxTime);
}
/**
* @return Timescale of the fragment with the smaller timestamp.
*
*/
public Optional timescaleOfMinTime() {
return Optional.ofNullable(this.timescaleOfMinTime);
}
/**
* @return Track index.
*
*/
public Optional trackId() {
return Optional.ofNullable(this.trackId);
}
/**
* @return Name of the track.
*
*/
public Optional trackName() {
return Optional.ofNullable(this.trackName);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(LiveEventStreamEventDataResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable Double bitrate;
private @Nullable String currentFragmentTimestamp;
private @Nullable Double discontinuityGap;
private @Nullable String duration;
private @Nullable String fragmentDropReason;
private @Nullable String fragmentOneDuration;
private @Nullable String fragmentOneTimestamp;
private @Nullable String fragmentTwoDuration;
private @Nullable String fragmentTwoTimestamp;
private @Nullable String maxTime;
private @Nullable String maxTimeMediaType;
private @Nullable String mediaTimestamp;
private @Nullable String mediaType;
private @Nullable String minTime;
private @Nullable String minTimeMediaType;
private @Nullable String previousFragmentDuration;
private @Nullable String previousFragmentTimestamp;
private @Nullable String remoteIp;
private @Nullable String remotePort;
private @Nullable String resolution;
private @Nullable String resultCode;
private @Nullable String resultMessage;
private @Nullable String streamId;
private @Nullable String streamName;
private @Nullable String timescale;
private @Nullable String timescaleOfMaxTime;
private @Nullable String timescaleOfMinTime;
private @Nullable Integer trackId;
private @Nullable String trackName;
public Builder() {}
public Builder(LiveEventStreamEventDataResponse defaults) {
Objects.requireNonNull(defaults);
this.bitrate = defaults.bitrate;
this.currentFragmentTimestamp = defaults.currentFragmentTimestamp;
this.discontinuityGap = defaults.discontinuityGap;
this.duration = defaults.duration;
this.fragmentDropReason = defaults.fragmentDropReason;
this.fragmentOneDuration = defaults.fragmentOneDuration;
this.fragmentOneTimestamp = defaults.fragmentOneTimestamp;
this.fragmentTwoDuration = defaults.fragmentTwoDuration;
this.fragmentTwoTimestamp = defaults.fragmentTwoTimestamp;
this.maxTime = defaults.maxTime;
this.maxTimeMediaType = defaults.maxTimeMediaType;
this.mediaTimestamp = defaults.mediaTimestamp;
this.mediaType = defaults.mediaType;
this.minTime = defaults.minTime;
this.minTimeMediaType = defaults.minTimeMediaType;
this.previousFragmentDuration = defaults.previousFragmentDuration;
this.previousFragmentTimestamp = defaults.previousFragmentTimestamp;
this.remoteIp = defaults.remoteIp;
this.remotePort = defaults.remotePort;
this.resolution = defaults.resolution;
this.resultCode = defaults.resultCode;
this.resultMessage = defaults.resultMessage;
this.streamId = defaults.streamId;
this.streamName = defaults.streamName;
this.timescale = defaults.timescale;
this.timescaleOfMaxTime = defaults.timescaleOfMaxTime;
this.timescaleOfMinTime = defaults.timescaleOfMinTime;
this.trackId = defaults.trackId;
this.trackName = defaults.trackName;
}
@CustomType.Setter
public Builder bitrate(@Nullable Double bitrate) {
this.bitrate = bitrate;
return this;
}
@CustomType.Setter
public Builder currentFragmentTimestamp(@Nullable String currentFragmentTimestamp) {
this.currentFragmentTimestamp = currentFragmentTimestamp;
return this;
}
@CustomType.Setter
public Builder discontinuityGap(@Nullable Double discontinuityGap) {
this.discontinuityGap = discontinuityGap;
return this;
}
@CustomType.Setter
public Builder duration(@Nullable String duration) {
this.duration = duration;
return this;
}
@CustomType.Setter
public Builder fragmentDropReason(@Nullable String fragmentDropReason) {
this.fragmentDropReason = fragmentDropReason;
return this;
}
@CustomType.Setter
public Builder fragmentOneDuration(@Nullable String fragmentOneDuration) {
this.fragmentOneDuration = fragmentOneDuration;
return this;
}
@CustomType.Setter
public Builder fragmentOneTimestamp(@Nullable String fragmentOneTimestamp) {
this.fragmentOneTimestamp = fragmentOneTimestamp;
return this;
}
@CustomType.Setter
public Builder fragmentTwoDuration(@Nullable String fragmentTwoDuration) {
this.fragmentTwoDuration = fragmentTwoDuration;
return this;
}
@CustomType.Setter
public Builder fragmentTwoTimestamp(@Nullable String fragmentTwoTimestamp) {
this.fragmentTwoTimestamp = fragmentTwoTimestamp;
return this;
}
@CustomType.Setter
public Builder maxTime(@Nullable String maxTime) {
this.maxTime = maxTime;
return this;
}
@CustomType.Setter
public Builder maxTimeMediaType(@Nullable String maxTimeMediaType) {
this.maxTimeMediaType = maxTimeMediaType;
return this;
}
@CustomType.Setter
public Builder mediaTimestamp(@Nullable String mediaTimestamp) {
this.mediaTimestamp = mediaTimestamp;
return this;
}
@CustomType.Setter
public Builder mediaType(@Nullable String mediaType) {
this.mediaType = mediaType;
return this;
}
@CustomType.Setter
public Builder minTime(@Nullable String minTime) {
this.minTime = minTime;
return this;
}
@CustomType.Setter
public Builder minTimeMediaType(@Nullable String minTimeMediaType) {
this.minTimeMediaType = minTimeMediaType;
return this;
}
@CustomType.Setter
public Builder previousFragmentDuration(@Nullable String previousFragmentDuration) {
this.previousFragmentDuration = previousFragmentDuration;
return this;
}
@CustomType.Setter
public Builder previousFragmentTimestamp(@Nullable String previousFragmentTimestamp) {
this.previousFragmentTimestamp = previousFragmentTimestamp;
return this;
}
@CustomType.Setter
public Builder remoteIp(@Nullable String remoteIp) {
this.remoteIp = remoteIp;
return this;
}
@CustomType.Setter
public Builder remotePort(@Nullable String remotePort) {
this.remotePort = remotePort;
return this;
}
@CustomType.Setter
public Builder resolution(@Nullable String resolution) {
this.resolution = resolution;
return this;
}
@CustomType.Setter
public Builder resultCode(@Nullable String resultCode) {
this.resultCode = resultCode;
return this;
}
@CustomType.Setter
public Builder resultMessage(@Nullable String resultMessage) {
this.resultMessage = resultMessage;
return this;
}
@CustomType.Setter
public Builder streamId(@Nullable String streamId) {
this.streamId = streamId;
return this;
}
@CustomType.Setter
public Builder streamName(@Nullable String streamName) {
this.streamName = streamName;
return this;
}
@CustomType.Setter
public Builder timescale(@Nullable String timescale) {
this.timescale = timescale;
return this;
}
@CustomType.Setter
public Builder timescaleOfMaxTime(@Nullable String timescaleOfMaxTime) {
this.timescaleOfMaxTime = timescaleOfMaxTime;
return this;
}
@CustomType.Setter
public Builder timescaleOfMinTime(@Nullable String timescaleOfMinTime) {
this.timescaleOfMinTime = timescaleOfMinTime;
return this;
}
@CustomType.Setter
public Builder trackId(@Nullable Integer trackId) {
this.trackId = trackId;
return this;
}
@CustomType.Setter
public Builder trackName(@Nullable String trackName) {
this.trackName = trackName;
return this;
}
public LiveEventStreamEventDataResponse build() {
final var _resultValue = new LiveEventStreamEventDataResponse();
_resultValue.bitrate = bitrate;
_resultValue.currentFragmentTimestamp = currentFragmentTimestamp;
_resultValue.discontinuityGap = discontinuityGap;
_resultValue.duration = duration;
_resultValue.fragmentDropReason = fragmentDropReason;
_resultValue.fragmentOneDuration = fragmentOneDuration;
_resultValue.fragmentOneTimestamp = fragmentOneTimestamp;
_resultValue.fragmentTwoDuration = fragmentTwoDuration;
_resultValue.fragmentTwoTimestamp = fragmentTwoTimestamp;
_resultValue.maxTime = maxTime;
_resultValue.maxTimeMediaType = maxTimeMediaType;
_resultValue.mediaTimestamp = mediaTimestamp;
_resultValue.mediaType = mediaType;
_resultValue.minTime = minTime;
_resultValue.minTimeMediaType = minTimeMediaType;
_resultValue.previousFragmentDuration = previousFragmentDuration;
_resultValue.previousFragmentTimestamp = previousFragmentTimestamp;
_resultValue.remoteIp = remoteIp;
_resultValue.remotePort = remotePort;
_resultValue.resolution = resolution;
_resultValue.resultCode = resultCode;
_resultValue.resultMessage = resultMessage;
_resultValue.streamId = streamId;
_resultValue.streamName = streamName;
_resultValue.timescale = timescale;
_resultValue.timescaleOfMaxTime = timescaleOfMaxTime;
_resultValue.timescaleOfMinTime = timescaleOfMinTime;
_resultValue.trackId = trackId;
_resultValue.trackName = trackName;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy