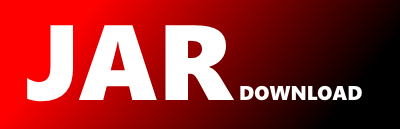
com.pulumi.azurenative.migrate.outputs.AKSAssessmentSettingsResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.migrate.outputs;
import com.pulumi.azurenative.migrate.outputs.PerfDataSettingsResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Double;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class AKSAssessmentSettingsResponse {
/**
* @return Gets or sets azure location.
*
*/
private String azureLocation;
/**
* @return Gets or sets azure VM category.
*
*/
private String category;
/**
* @return Gets or sets consolidation type.
*
*/
private String consolidation;
/**
* @return Gets or sets currency.
*
*/
private String currency;
/**
* @return Gets or sets discount percentage.
*
*/
private @Nullable Double discountPercentage;
/**
* @return Gets or sets environment type.
*
*/
private String environmentType;
/**
* @return Gets or sets licensing program.
*
*/
private String licensingProgram;
/**
* @return Gets or sets performance data settings.
*
*/
private @Nullable PerfDataSettingsResponse performanceData;
/**
* @return Gets or sets pricing tier.
*
*/
private String pricingTier;
/**
* @return Gets or sets savings options.
*
*/
private String savingsOptions;
/**
* @return Gets or sets scaling factor.
*
*/
private @Nullable Double scalingFactor;
/**
* @return Gets or sets sizing criteria.
*
*/
private String sizingCriteria;
private AKSAssessmentSettingsResponse() {}
/**
* @return Gets or sets azure location.
*
*/
public String azureLocation() {
return this.azureLocation;
}
/**
* @return Gets or sets azure VM category.
*
*/
public String category() {
return this.category;
}
/**
* @return Gets or sets consolidation type.
*
*/
public String consolidation() {
return this.consolidation;
}
/**
* @return Gets or sets currency.
*
*/
public String currency() {
return this.currency;
}
/**
* @return Gets or sets discount percentage.
*
*/
public Optional discountPercentage() {
return Optional.ofNullable(this.discountPercentage);
}
/**
* @return Gets or sets environment type.
*
*/
public String environmentType() {
return this.environmentType;
}
/**
* @return Gets or sets licensing program.
*
*/
public String licensingProgram() {
return this.licensingProgram;
}
/**
* @return Gets or sets performance data settings.
*
*/
public Optional performanceData() {
return Optional.ofNullable(this.performanceData);
}
/**
* @return Gets or sets pricing tier.
*
*/
public String pricingTier() {
return this.pricingTier;
}
/**
* @return Gets or sets savings options.
*
*/
public String savingsOptions() {
return this.savingsOptions;
}
/**
* @return Gets or sets scaling factor.
*
*/
public Optional scalingFactor() {
return Optional.ofNullable(this.scalingFactor);
}
/**
* @return Gets or sets sizing criteria.
*
*/
public String sizingCriteria() {
return this.sizingCriteria;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(AKSAssessmentSettingsResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String azureLocation;
private String category;
private String consolidation;
private String currency;
private @Nullable Double discountPercentage;
private String environmentType;
private String licensingProgram;
private @Nullable PerfDataSettingsResponse performanceData;
private String pricingTier;
private String savingsOptions;
private @Nullable Double scalingFactor;
private String sizingCriteria;
public Builder() {}
public Builder(AKSAssessmentSettingsResponse defaults) {
Objects.requireNonNull(defaults);
this.azureLocation = defaults.azureLocation;
this.category = defaults.category;
this.consolidation = defaults.consolidation;
this.currency = defaults.currency;
this.discountPercentage = defaults.discountPercentage;
this.environmentType = defaults.environmentType;
this.licensingProgram = defaults.licensingProgram;
this.performanceData = defaults.performanceData;
this.pricingTier = defaults.pricingTier;
this.savingsOptions = defaults.savingsOptions;
this.scalingFactor = defaults.scalingFactor;
this.sizingCriteria = defaults.sizingCriteria;
}
@CustomType.Setter
public Builder azureLocation(String azureLocation) {
if (azureLocation == null) {
throw new MissingRequiredPropertyException("AKSAssessmentSettingsResponse", "azureLocation");
}
this.azureLocation = azureLocation;
return this;
}
@CustomType.Setter
public Builder category(String category) {
if (category == null) {
throw new MissingRequiredPropertyException("AKSAssessmentSettingsResponse", "category");
}
this.category = category;
return this;
}
@CustomType.Setter
public Builder consolidation(String consolidation) {
if (consolidation == null) {
throw new MissingRequiredPropertyException("AKSAssessmentSettingsResponse", "consolidation");
}
this.consolidation = consolidation;
return this;
}
@CustomType.Setter
public Builder currency(String currency) {
if (currency == null) {
throw new MissingRequiredPropertyException("AKSAssessmentSettingsResponse", "currency");
}
this.currency = currency;
return this;
}
@CustomType.Setter
public Builder discountPercentage(@Nullable Double discountPercentage) {
this.discountPercentage = discountPercentage;
return this;
}
@CustomType.Setter
public Builder environmentType(String environmentType) {
if (environmentType == null) {
throw new MissingRequiredPropertyException("AKSAssessmentSettingsResponse", "environmentType");
}
this.environmentType = environmentType;
return this;
}
@CustomType.Setter
public Builder licensingProgram(String licensingProgram) {
if (licensingProgram == null) {
throw new MissingRequiredPropertyException("AKSAssessmentSettingsResponse", "licensingProgram");
}
this.licensingProgram = licensingProgram;
return this;
}
@CustomType.Setter
public Builder performanceData(@Nullable PerfDataSettingsResponse performanceData) {
this.performanceData = performanceData;
return this;
}
@CustomType.Setter
public Builder pricingTier(String pricingTier) {
if (pricingTier == null) {
throw new MissingRequiredPropertyException("AKSAssessmentSettingsResponse", "pricingTier");
}
this.pricingTier = pricingTier;
return this;
}
@CustomType.Setter
public Builder savingsOptions(String savingsOptions) {
if (savingsOptions == null) {
throw new MissingRequiredPropertyException("AKSAssessmentSettingsResponse", "savingsOptions");
}
this.savingsOptions = savingsOptions;
return this;
}
@CustomType.Setter
public Builder scalingFactor(@Nullable Double scalingFactor) {
this.scalingFactor = scalingFactor;
return this;
}
@CustomType.Setter
public Builder sizingCriteria(String sizingCriteria) {
if (sizingCriteria == null) {
throw new MissingRequiredPropertyException("AKSAssessmentSettingsResponse", "sizingCriteria");
}
this.sizingCriteria = sizingCriteria;
return this;
}
public AKSAssessmentSettingsResponse build() {
final var _resultValue = new AKSAssessmentSettingsResponse();
_resultValue.azureLocation = azureLocation;
_resultValue.category = category;
_resultValue.consolidation = consolidation;
_resultValue.currency = currency;
_resultValue.discountPercentage = discountPercentage;
_resultValue.environmentType = environmentType;
_resultValue.licensingProgram = licensingProgram;
_resultValue.performanceData = performanceData;
_resultValue.pricingTier = pricingTier;
_resultValue.savingsOptions = savingsOptions;
_resultValue.scalingFactor = scalingFactor;
_resultValue.sizingCriteria = sizingCriteria;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy