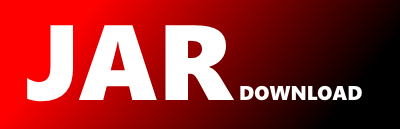
com.pulumi.azurenative.mobilenetwork.MobilenetworkFunctions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.mobilenetwork;
import com.pulumi.azurenative.Utilities;
import com.pulumi.azurenative.mobilenetwork.inputs.GetAttachedDataNetworkArgs;
import com.pulumi.azurenative.mobilenetwork.inputs.GetAttachedDataNetworkPlainArgs;
import com.pulumi.azurenative.mobilenetwork.inputs.GetDataNetworkArgs;
import com.pulumi.azurenative.mobilenetwork.inputs.GetDataNetworkPlainArgs;
import com.pulumi.azurenative.mobilenetwork.inputs.GetDiagnosticsPackageArgs;
import com.pulumi.azurenative.mobilenetwork.inputs.GetDiagnosticsPackagePlainArgs;
import com.pulumi.azurenative.mobilenetwork.inputs.GetMobileNetworkArgs;
import com.pulumi.azurenative.mobilenetwork.inputs.GetMobileNetworkPlainArgs;
import com.pulumi.azurenative.mobilenetwork.inputs.GetPacketCaptureArgs;
import com.pulumi.azurenative.mobilenetwork.inputs.GetPacketCapturePlainArgs;
import com.pulumi.azurenative.mobilenetwork.inputs.GetPacketCoreControlPlaneArgs;
import com.pulumi.azurenative.mobilenetwork.inputs.GetPacketCoreControlPlanePlainArgs;
import com.pulumi.azurenative.mobilenetwork.inputs.GetPacketCoreDataPlaneArgs;
import com.pulumi.azurenative.mobilenetwork.inputs.GetPacketCoreDataPlanePlainArgs;
import com.pulumi.azurenative.mobilenetwork.inputs.GetServiceArgs;
import com.pulumi.azurenative.mobilenetwork.inputs.GetServicePlainArgs;
import com.pulumi.azurenative.mobilenetwork.inputs.GetSimArgs;
import com.pulumi.azurenative.mobilenetwork.inputs.GetSimGroupArgs;
import com.pulumi.azurenative.mobilenetwork.inputs.GetSimGroupPlainArgs;
import com.pulumi.azurenative.mobilenetwork.inputs.GetSimPlainArgs;
import com.pulumi.azurenative.mobilenetwork.inputs.GetSimPolicyArgs;
import com.pulumi.azurenative.mobilenetwork.inputs.GetSimPolicyPlainArgs;
import com.pulumi.azurenative.mobilenetwork.inputs.GetSiteArgs;
import com.pulumi.azurenative.mobilenetwork.inputs.GetSitePlainArgs;
import com.pulumi.azurenative.mobilenetwork.inputs.GetSliceArgs;
import com.pulumi.azurenative.mobilenetwork.inputs.GetSlicePlainArgs;
import com.pulumi.azurenative.mobilenetwork.inputs.ListMobileNetworkSimGroupsArgs;
import com.pulumi.azurenative.mobilenetwork.inputs.ListMobileNetworkSimGroupsPlainArgs;
import com.pulumi.azurenative.mobilenetwork.inputs.ListMobileNetworkSimIdsArgs;
import com.pulumi.azurenative.mobilenetwork.inputs.ListMobileNetworkSimIdsPlainArgs;
import com.pulumi.azurenative.mobilenetwork.outputs.GetAttachedDataNetworkResult;
import com.pulumi.azurenative.mobilenetwork.outputs.GetDataNetworkResult;
import com.pulumi.azurenative.mobilenetwork.outputs.GetDiagnosticsPackageResult;
import com.pulumi.azurenative.mobilenetwork.outputs.GetMobileNetworkResult;
import com.pulumi.azurenative.mobilenetwork.outputs.GetPacketCaptureResult;
import com.pulumi.azurenative.mobilenetwork.outputs.GetPacketCoreControlPlaneResult;
import com.pulumi.azurenative.mobilenetwork.outputs.GetPacketCoreDataPlaneResult;
import com.pulumi.azurenative.mobilenetwork.outputs.GetServiceResult;
import com.pulumi.azurenative.mobilenetwork.outputs.GetSimGroupResult;
import com.pulumi.azurenative.mobilenetwork.outputs.GetSimPolicyResult;
import com.pulumi.azurenative.mobilenetwork.outputs.GetSimResult;
import com.pulumi.azurenative.mobilenetwork.outputs.GetSiteResult;
import com.pulumi.azurenative.mobilenetwork.outputs.GetSliceResult;
import com.pulumi.azurenative.mobilenetwork.outputs.ListMobileNetworkSimGroupsResult;
import com.pulumi.azurenative.mobilenetwork.outputs.ListMobileNetworkSimIdsResult;
import com.pulumi.core.Output;
import com.pulumi.core.TypeShape;
import com.pulumi.deployment.Deployment;
import com.pulumi.deployment.InvokeOptions;
import java.util.concurrent.CompletableFuture;
public final class MobilenetworkFunctions {
/**
* Gets information about the specified attached data network.
* Azure REST API version: 2023-06-01.
*
* Other available API versions: 2022-04-01-preview, 2022-11-01, 2023-09-01, 2024-02-01, 2024-04-01.
*
*/
public static Output getAttachedDataNetwork(GetAttachedDataNetworkArgs args) {
return getAttachedDataNetwork(args, InvokeOptions.Empty);
}
/**
* Gets information about the specified attached data network.
* Azure REST API version: 2023-06-01.
*
* Other available API versions: 2022-04-01-preview, 2022-11-01, 2023-09-01, 2024-02-01, 2024-04-01.
*
*/
public static CompletableFuture getAttachedDataNetworkPlain(GetAttachedDataNetworkPlainArgs args) {
return getAttachedDataNetworkPlain(args, InvokeOptions.Empty);
}
/**
* Gets information about the specified attached data network.
* Azure REST API version: 2023-06-01.
*
* Other available API versions: 2022-04-01-preview, 2022-11-01, 2023-09-01, 2024-02-01, 2024-04-01.
*
*/
public static Output getAttachedDataNetwork(GetAttachedDataNetworkArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:mobilenetwork:getAttachedDataNetwork", TypeShape.of(GetAttachedDataNetworkResult.class), args, Utilities.withVersion(options));
}
/**
* Gets information about the specified attached data network.
* Azure REST API version: 2023-06-01.
*
* Other available API versions: 2022-04-01-preview, 2022-11-01, 2023-09-01, 2024-02-01, 2024-04-01.
*
*/
public static CompletableFuture getAttachedDataNetworkPlain(GetAttachedDataNetworkPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:mobilenetwork:getAttachedDataNetwork", TypeShape.of(GetAttachedDataNetworkResult.class), args, Utilities.withVersion(options));
}
/**
* Gets information about the specified data network.
* Azure REST API version: 2023-06-01.
*
* Other available API versions: 2022-04-01-preview, 2022-11-01, 2023-09-01, 2024-02-01, 2024-04-01.
*
*/
public static Output getDataNetwork(GetDataNetworkArgs args) {
return getDataNetwork(args, InvokeOptions.Empty);
}
/**
* Gets information about the specified data network.
* Azure REST API version: 2023-06-01.
*
* Other available API versions: 2022-04-01-preview, 2022-11-01, 2023-09-01, 2024-02-01, 2024-04-01.
*
*/
public static CompletableFuture getDataNetworkPlain(GetDataNetworkPlainArgs args) {
return getDataNetworkPlain(args, InvokeOptions.Empty);
}
/**
* Gets information about the specified data network.
* Azure REST API version: 2023-06-01.
*
* Other available API versions: 2022-04-01-preview, 2022-11-01, 2023-09-01, 2024-02-01, 2024-04-01.
*
*/
public static Output getDataNetwork(GetDataNetworkArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:mobilenetwork:getDataNetwork", TypeShape.of(GetDataNetworkResult.class), args, Utilities.withVersion(options));
}
/**
* Gets information about the specified data network.
* Azure REST API version: 2023-06-01.
*
* Other available API versions: 2022-04-01-preview, 2022-11-01, 2023-09-01, 2024-02-01, 2024-04-01.
*
*/
public static CompletableFuture getDataNetworkPlain(GetDataNetworkPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:mobilenetwork:getDataNetwork", TypeShape.of(GetDataNetworkResult.class), args, Utilities.withVersion(options));
}
/**
* Gets information about the specified diagnostics package.
* Azure REST API version: 2023-06-01.
*
* Other available API versions: 2023-09-01, 2024-02-01, 2024-04-01.
*
*/
public static Output getDiagnosticsPackage(GetDiagnosticsPackageArgs args) {
return getDiagnosticsPackage(args, InvokeOptions.Empty);
}
/**
* Gets information about the specified diagnostics package.
* Azure REST API version: 2023-06-01.
*
* Other available API versions: 2023-09-01, 2024-02-01, 2024-04-01.
*
*/
public static CompletableFuture getDiagnosticsPackagePlain(GetDiagnosticsPackagePlainArgs args) {
return getDiagnosticsPackagePlain(args, InvokeOptions.Empty);
}
/**
* Gets information about the specified diagnostics package.
* Azure REST API version: 2023-06-01.
*
* Other available API versions: 2023-09-01, 2024-02-01, 2024-04-01.
*
*/
public static Output getDiagnosticsPackage(GetDiagnosticsPackageArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:mobilenetwork:getDiagnosticsPackage", TypeShape.of(GetDiagnosticsPackageResult.class), args, Utilities.withVersion(options));
}
/**
* Gets information about the specified diagnostics package.
* Azure REST API version: 2023-06-01.
*
* Other available API versions: 2023-09-01, 2024-02-01, 2024-04-01.
*
*/
public static CompletableFuture getDiagnosticsPackagePlain(GetDiagnosticsPackagePlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:mobilenetwork:getDiagnosticsPackage", TypeShape.of(GetDiagnosticsPackageResult.class), args, Utilities.withVersion(options));
}
/**
* Gets information about the specified mobile network.
* Azure REST API version: 2023-06-01.
*
* Other available API versions: 2022-04-01-preview, 2022-11-01, 2023-09-01, 2024-02-01, 2024-04-01.
*
*/
public static Output getMobileNetwork(GetMobileNetworkArgs args) {
return getMobileNetwork(args, InvokeOptions.Empty);
}
/**
* Gets information about the specified mobile network.
* Azure REST API version: 2023-06-01.
*
* Other available API versions: 2022-04-01-preview, 2022-11-01, 2023-09-01, 2024-02-01, 2024-04-01.
*
*/
public static CompletableFuture getMobileNetworkPlain(GetMobileNetworkPlainArgs args) {
return getMobileNetworkPlain(args, InvokeOptions.Empty);
}
/**
* Gets information about the specified mobile network.
* Azure REST API version: 2023-06-01.
*
* Other available API versions: 2022-04-01-preview, 2022-11-01, 2023-09-01, 2024-02-01, 2024-04-01.
*
*/
public static Output getMobileNetwork(GetMobileNetworkArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:mobilenetwork:getMobileNetwork", TypeShape.of(GetMobileNetworkResult.class), args, Utilities.withVersion(options));
}
/**
* Gets information about the specified mobile network.
* Azure REST API version: 2023-06-01.
*
* Other available API versions: 2022-04-01-preview, 2022-11-01, 2023-09-01, 2024-02-01, 2024-04-01.
*
*/
public static CompletableFuture getMobileNetworkPlain(GetMobileNetworkPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:mobilenetwork:getMobileNetwork", TypeShape.of(GetMobileNetworkResult.class), args, Utilities.withVersion(options));
}
/**
* Gets information about the specified packet capture session.
* Azure REST API version: 2023-06-01.
*
* Other available API versions: 2023-09-01, 2024-02-01, 2024-04-01.
*
*/
public static Output getPacketCapture(GetPacketCaptureArgs args) {
return getPacketCapture(args, InvokeOptions.Empty);
}
/**
* Gets information about the specified packet capture session.
* Azure REST API version: 2023-06-01.
*
* Other available API versions: 2023-09-01, 2024-02-01, 2024-04-01.
*
*/
public static CompletableFuture getPacketCapturePlain(GetPacketCapturePlainArgs args) {
return getPacketCapturePlain(args, InvokeOptions.Empty);
}
/**
* Gets information about the specified packet capture session.
* Azure REST API version: 2023-06-01.
*
* Other available API versions: 2023-09-01, 2024-02-01, 2024-04-01.
*
*/
public static Output getPacketCapture(GetPacketCaptureArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:mobilenetwork:getPacketCapture", TypeShape.of(GetPacketCaptureResult.class), args, Utilities.withVersion(options));
}
/**
* Gets information about the specified packet capture session.
* Azure REST API version: 2023-06-01.
*
* Other available API versions: 2023-09-01, 2024-02-01, 2024-04-01.
*
*/
public static CompletableFuture getPacketCapturePlain(GetPacketCapturePlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:mobilenetwork:getPacketCapture", TypeShape.of(GetPacketCaptureResult.class), args, Utilities.withVersion(options));
}
/**
* Gets information about the specified packet core control plane.
* Azure REST API version: 2023-06-01.
*
* Other available API versions: 2022-03-01-preview, 2022-04-01-preview, 2022-11-01, 2023-09-01, 2024-02-01, 2024-04-01.
*
*/
public static Output getPacketCoreControlPlane(GetPacketCoreControlPlaneArgs args) {
return getPacketCoreControlPlane(args, InvokeOptions.Empty);
}
/**
* Gets information about the specified packet core control plane.
* Azure REST API version: 2023-06-01.
*
* Other available API versions: 2022-03-01-preview, 2022-04-01-preview, 2022-11-01, 2023-09-01, 2024-02-01, 2024-04-01.
*
*/
public static CompletableFuture getPacketCoreControlPlanePlain(GetPacketCoreControlPlanePlainArgs args) {
return getPacketCoreControlPlanePlain(args, InvokeOptions.Empty);
}
/**
* Gets information about the specified packet core control plane.
* Azure REST API version: 2023-06-01.
*
* Other available API versions: 2022-03-01-preview, 2022-04-01-preview, 2022-11-01, 2023-09-01, 2024-02-01, 2024-04-01.
*
*/
public static Output getPacketCoreControlPlane(GetPacketCoreControlPlaneArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:mobilenetwork:getPacketCoreControlPlane", TypeShape.of(GetPacketCoreControlPlaneResult.class), args, Utilities.withVersion(options));
}
/**
* Gets information about the specified packet core control plane.
* Azure REST API version: 2023-06-01.
*
* Other available API versions: 2022-03-01-preview, 2022-04-01-preview, 2022-11-01, 2023-09-01, 2024-02-01, 2024-04-01.
*
*/
public static CompletableFuture getPacketCoreControlPlanePlain(GetPacketCoreControlPlanePlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:mobilenetwork:getPacketCoreControlPlane", TypeShape.of(GetPacketCoreControlPlaneResult.class), args, Utilities.withVersion(options));
}
/**
* Gets information about the specified packet core data plane.
* Azure REST API version: 2023-06-01.
*
* Other available API versions: 2022-04-01-preview, 2022-11-01, 2023-09-01, 2024-02-01, 2024-04-01.
*
*/
public static Output getPacketCoreDataPlane(GetPacketCoreDataPlaneArgs args) {
return getPacketCoreDataPlane(args, InvokeOptions.Empty);
}
/**
* Gets information about the specified packet core data plane.
* Azure REST API version: 2023-06-01.
*
* Other available API versions: 2022-04-01-preview, 2022-11-01, 2023-09-01, 2024-02-01, 2024-04-01.
*
*/
public static CompletableFuture getPacketCoreDataPlanePlain(GetPacketCoreDataPlanePlainArgs args) {
return getPacketCoreDataPlanePlain(args, InvokeOptions.Empty);
}
/**
* Gets information about the specified packet core data plane.
* Azure REST API version: 2023-06-01.
*
* Other available API versions: 2022-04-01-preview, 2022-11-01, 2023-09-01, 2024-02-01, 2024-04-01.
*
*/
public static Output getPacketCoreDataPlane(GetPacketCoreDataPlaneArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:mobilenetwork:getPacketCoreDataPlane", TypeShape.of(GetPacketCoreDataPlaneResult.class), args, Utilities.withVersion(options));
}
/**
* Gets information about the specified packet core data plane.
* Azure REST API version: 2023-06-01.
*
* Other available API versions: 2022-04-01-preview, 2022-11-01, 2023-09-01, 2024-02-01, 2024-04-01.
*
*/
public static CompletableFuture getPacketCoreDataPlanePlain(GetPacketCoreDataPlanePlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:mobilenetwork:getPacketCoreDataPlane", TypeShape.of(GetPacketCoreDataPlaneResult.class), args, Utilities.withVersion(options));
}
/**
* Gets information about the specified service.
* Azure REST API version: 2023-06-01.
*
* Other available API versions: 2022-04-01-preview, 2022-11-01, 2023-09-01, 2024-02-01, 2024-04-01.
*
*/
public static Output getService(GetServiceArgs args) {
return getService(args, InvokeOptions.Empty);
}
/**
* Gets information about the specified service.
* Azure REST API version: 2023-06-01.
*
* Other available API versions: 2022-04-01-preview, 2022-11-01, 2023-09-01, 2024-02-01, 2024-04-01.
*
*/
public static CompletableFuture getServicePlain(GetServicePlainArgs args) {
return getServicePlain(args, InvokeOptions.Empty);
}
/**
* Gets information about the specified service.
* Azure REST API version: 2023-06-01.
*
* Other available API versions: 2022-04-01-preview, 2022-11-01, 2023-09-01, 2024-02-01, 2024-04-01.
*
*/
public static Output getService(GetServiceArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:mobilenetwork:getService", TypeShape.of(GetServiceResult.class), args, Utilities.withVersion(options));
}
/**
* Gets information about the specified service.
* Azure REST API version: 2023-06-01.
*
* Other available API versions: 2022-04-01-preview, 2022-11-01, 2023-09-01, 2024-02-01, 2024-04-01.
*
*/
public static CompletableFuture getServicePlain(GetServicePlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:mobilenetwork:getService", TypeShape.of(GetServiceResult.class), args, Utilities.withVersion(options));
}
/**
* Gets information about the specified SIM.
* Azure REST API version: 2023-06-01.
*
* Other available API versions: 2022-03-01-preview, 2022-04-01-preview, 2022-11-01, 2023-09-01, 2024-02-01, 2024-04-01.
*
*/
public static Output getSim(GetSimArgs args) {
return getSim(args, InvokeOptions.Empty);
}
/**
* Gets information about the specified SIM.
* Azure REST API version: 2023-06-01.
*
* Other available API versions: 2022-03-01-preview, 2022-04-01-preview, 2022-11-01, 2023-09-01, 2024-02-01, 2024-04-01.
*
*/
public static CompletableFuture getSimPlain(GetSimPlainArgs args) {
return getSimPlain(args, InvokeOptions.Empty);
}
/**
* Gets information about the specified SIM.
* Azure REST API version: 2023-06-01.
*
* Other available API versions: 2022-03-01-preview, 2022-04-01-preview, 2022-11-01, 2023-09-01, 2024-02-01, 2024-04-01.
*
*/
public static Output getSim(GetSimArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:mobilenetwork:getSim", TypeShape.of(GetSimResult.class), args, Utilities.withVersion(options));
}
/**
* Gets information about the specified SIM.
* Azure REST API version: 2023-06-01.
*
* Other available API versions: 2022-03-01-preview, 2022-04-01-preview, 2022-11-01, 2023-09-01, 2024-02-01, 2024-04-01.
*
*/
public static CompletableFuture getSimPlain(GetSimPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:mobilenetwork:getSim", TypeShape.of(GetSimResult.class), args, Utilities.withVersion(options));
}
/**
* Gets information about the specified SIM group.
* Azure REST API version: 2023-06-01.
*
* Other available API versions: 2022-04-01-preview, 2022-11-01, 2023-09-01, 2024-02-01, 2024-04-01.
*
*/
public static Output getSimGroup(GetSimGroupArgs args) {
return getSimGroup(args, InvokeOptions.Empty);
}
/**
* Gets information about the specified SIM group.
* Azure REST API version: 2023-06-01.
*
* Other available API versions: 2022-04-01-preview, 2022-11-01, 2023-09-01, 2024-02-01, 2024-04-01.
*
*/
public static CompletableFuture getSimGroupPlain(GetSimGroupPlainArgs args) {
return getSimGroupPlain(args, InvokeOptions.Empty);
}
/**
* Gets information about the specified SIM group.
* Azure REST API version: 2023-06-01.
*
* Other available API versions: 2022-04-01-preview, 2022-11-01, 2023-09-01, 2024-02-01, 2024-04-01.
*
*/
public static Output getSimGroup(GetSimGroupArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:mobilenetwork:getSimGroup", TypeShape.of(GetSimGroupResult.class), args, Utilities.withVersion(options));
}
/**
* Gets information about the specified SIM group.
* Azure REST API version: 2023-06-01.
*
* Other available API versions: 2022-04-01-preview, 2022-11-01, 2023-09-01, 2024-02-01, 2024-04-01.
*
*/
public static CompletableFuture getSimGroupPlain(GetSimGroupPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:mobilenetwork:getSimGroup", TypeShape.of(GetSimGroupResult.class), args, Utilities.withVersion(options));
}
/**
* Gets information about the specified SIM policy.
* Azure REST API version: 2023-06-01.
*
* Other available API versions: 2022-04-01-preview, 2022-11-01, 2023-09-01, 2024-02-01, 2024-04-01.
*
*/
public static Output getSimPolicy(GetSimPolicyArgs args) {
return getSimPolicy(args, InvokeOptions.Empty);
}
/**
* Gets information about the specified SIM policy.
* Azure REST API version: 2023-06-01.
*
* Other available API versions: 2022-04-01-preview, 2022-11-01, 2023-09-01, 2024-02-01, 2024-04-01.
*
*/
public static CompletableFuture getSimPolicyPlain(GetSimPolicyPlainArgs args) {
return getSimPolicyPlain(args, InvokeOptions.Empty);
}
/**
* Gets information about the specified SIM policy.
* Azure REST API version: 2023-06-01.
*
* Other available API versions: 2022-04-01-preview, 2022-11-01, 2023-09-01, 2024-02-01, 2024-04-01.
*
*/
public static Output getSimPolicy(GetSimPolicyArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:mobilenetwork:getSimPolicy", TypeShape.of(GetSimPolicyResult.class), args, Utilities.withVersion(options));
}
/**
* Gets information about the specified SIM policy.
* Azure REST API version: 2023-06-01.
*
* Other available API versions: 2022-04-01-preview, 2022-11-01, 2023-09-01, 2024-02-01, 2024-04-01.
*
*/
public static CompletableFuture getSimPolicyPlain(GetSimPolicyPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:mobilenetwork:getSimPolicy", TypeShape.of(GetSimPolicyResult.class), args, Utilities.withVersion(options));
}
/**
* Gets information about the specified mobile network site.
* Azure REST API version: 2023-06-01.
*
* Other available API versions: 2022-04-01-preview, 2022-11-01, 2023-09-01, 2024-02-01, 2024-04-01.
*
*/
public static Output getSite(GetSiteArgs args) {
return getSite(args, InvokeOptions.Empty);
}
/**
* Gets information about the specified mobile network site.
* Azure REST API version: 2023-06-01.
*
* Other available API versions: 2022-04-01-preview, 2022-11-01, 2023-09-01, 2024-02-01, 2024-04-01.
*
*/
public static CompletableFuture getSitePlain(GetSitePlainArgs args) {
return getSitePlain(args, InvokeOptions.Empty);
}
/**
* Gets information about the specified mobile network site.
* Azure REST API version: 2023-06-01.
*
* Other available API versions: 2022-04-01-preview, 2022-11-01, 2023-09-01, 2024-02-01, 2024-04-01.
*
*/
public static Output getSite(GetSiteArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:mobilenetwork:getSite", TypeShape.of(GetSiteResult.class), args, Utilities.withVersion(options));
}
/**
* Gets information about the specified mobile network site.
* Azure REST API version: 2023-06-01.
*
* Other available API versions: 2022-04-01-preview, 2022-11-01, 2023-09-01, 2024-02-01, 2024-04-01.
*
*/
public static CompletableFuture getSitePlain(GetSitePlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:mobilenetwork:getSite", TypeShape.of(GetSiteResult.class), args, Utilities.withVersion(options));
}
/**
* Gets information about the specified network slice.
* Azure REST API version: 2023-06-01.
*
* Other available API versions: 2022-04-01-preview, 2022-11-01, 2023-09-01, 2024-02-01, 2024-04-01.
*
*/
public static Output getSlice(GetSliceArgs args) {
return getSlice(args, InvokeOptions.Empty);
}
/**
* Gets information about the specified network slice.
* Azure REST API version: 2023-06-01.
*
* Other available API versions: 2022-04-01-preview, 2022-11-01, 2023-09-01, 2024-02-01, 2024-04-01.
*
*/
public static CompletableFuture getSlicePlain(GetSlicePlainArgs args) {
return getSlicePlain(args, InvokeOptions.Empty);
}
/**
* Gets information about the specified network slice.
* Azure REST API version: 2023-06-01.
*
* Other available API versions: 2022-04-01-preview, 2022-11-01, 2023-09-01, 2024-02-01, 2024-04-01.
*
*/
public static Output getSlice(GetSliceArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:mobilenetwork:getSlice", TypeShape.of(GetSliceResult.class), args, Utilities.withVersion(options));
}
/**
* Gets information about the specified network slice.
* Azure REST API version: 2023-06-01.
*
* Other available API versions: 2022-04-01-preview, 2022-11-01, 2023-09-01, 2024-02-01, 2024-04-01.
*
*/
public static CompletableFuture getSlicePlain(GetSlicePlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:mobilenetwork:getSlice", TypeShape.of(GetSliceResult.class), args, Utilities.withVersion(options));
}
/**
* Gets all the SIM groups assigned to a mobile network.
* Azure REST API version: 2024-04-01.
*
*/
public static Output listMobileNetworkSimGroups(ListMobileNetworkSimGroupsArgs args) {
return listMobileNetworkSimGroups(args, InvokeOptions.Empty);
}
/**
* Gets all the SIM groups assigned to a mobile network.
* Azure REST API version: 2024-04-01.
*
*/
public static CompletableFuture listMobileNetworkSimGroupsPlain(ListMobileNetworkSimGroupsPlainArgs args) {
return listMobileNetworkSimGroupsPlain(args, InvokeOptions.Empty);
}
/**
* Gets all the SIM groups assigned to a mobile network.
* Azure REST API version: 2024-04-01.
*
*/
public static Output listMobileNetworkSimGroups(ListMobileNetworkSimGroupsArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:mobilenetwork:listMobileNetworkSimGroups", TypeShape.of(ListMobileNetworkSimGroupsResult.class), args, Utilities.withVersion(options));
}
/**
* Gets all the SIM groups assigned to a mobile network.
* Azure REST API version: 2024-04-01.
*
*/
public static CompletableFuture listMobileNetworkSimGroupsPlain(ListMobileNetworkSimGroupsPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:mobilenetwork:listMobileNetworkSimGroups", TypeShape.of(ListMobileNetworkSimGroupsResult.class), args, Utilities.withVersion(options));
}
/**
* Lists the IDs of all provisioned SIMs in a mobile network
* Azure REST API version: 2022-04-01-preview.
*
*/
public static Output listMobileNetworkSimIds(ListMobileNetworkSimIdsArgs args) {
return listMobileNetworkSimIds(args, InvokeOptions.Empty);
}
/**
* Lists the IDs of all provisioned SIMs in a mobile network
* Azure REST API version: 2022-04-01-preview.
*
*/
public static CompletableFuture listMobileNetworkSimIdsPlain(ListMobileNetworkSimIdsPlainArgs args) {
return listMobileNetworkSimIdsPlain(args, InvokeOptions.Empty);
}
/**
* Lists the IDs of all provisioned SIMs in a mobile network
* Azure REST API version: 2022-04-01-preview.
*
*/
public static Output listMobileNetworkSimIds(ListMobileNetworkSimIdsArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:mobilenetwork:listMobileNetworkSimIds", TypeShape.of(ListMobileNetworkSimIdsResult.class), args, Utilities.withVersion(options));
}
/**
* Lists the IDs of all provisioned SIMs in a mobile network
* Azure REST API version: 2022-04-01-preview.
*
*/
public static CompletableFuture listMobileNetworkSimIdsPlain(ListMobileNetworkSimIdsPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:mobilenetwork:listMobileNetworkSimIds", TypeShape.of(ListMobileNetworkSimIdsResult.class), args, Utilities.withVersion(options));
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy