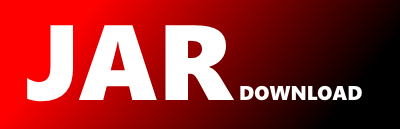
com.pulumi.azurenative.mobilepacketcore.MobilepacketcoreFunctions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.mobilepacketcore;
import com.pulumi.azurenative.Utilities;
import com.pulumi.azurenative.mobilepacketcore.inputs.GetAmfDeploymentArgs;
import com.pulumi.azurenative.mobilepacketcore.inputs.GetAmfDeploymentPlainArgs;
import com.pulumi.azurenative.mobilepacketcore.inputs.GetClusterServiceArgs;
import com.pulumi.azurenative.mobilepacketcore.inputs.GetClusterServicePlainArgs;
import com.pulumi.azurenative.mobilepacketcore.inputs.GetNetworkFunctionArgs;
import com.pulumi.azurenative.mobilepacketcore.inputs.GetNetworkFunctionPlainArgs;
import com.pulumi.azurenative.mobilepacketcore.inputs.GetNrfDeploymentArgs;
import com.pulumi.azurenative.mobilepacketcore.inputs.GetNrfDeploymentPlainArgs;
import com.pulumi.azurenative.mobilepacketcore.inputs.GetNssfDeploymentArgs;
import com.pulumi.azurenative.mobilepacketcore.inputs.GetNssfDeploymentPlainArgs;
import com.pulumi.azurenative.mobilepacketcore.inputs.GetObservabilityServiceArgs;
import com.pulumi.azurenative.mobilepacketcore.inputs.GetObservabilityServicePlainArgs;
import com.pulumi.azurenative.mobilepacketcore.inputs.GetSmfDeploymentArgs;
import com.pulumi.azurenative.mobilepacketcore.inputs.GetSmfDeploymentPlainArgs;
import com.pulumi.azurenative.mobilepacketcore.inputs.GetUpfDeploymentArgs;
import com.pulumi.azurenative.mobilepacketcore.inputs.GetUpfDeploymentPlainArgs;
import com.pulumi.azurenative.mobilepacketcore.outputs.GetAmfDeploymentResult;
import com.pulumi.azurenative.mobilepacketcore.outputs.GetClusterServiceResult;
import com.pulumi.azurenative.mobilepacketcore.outputs.GetNetworkFunctionResult;
import com.pulumi.azurenative.mobilepacketcore.outputs.GetNrfDeploymentResult;
import com.pulumi.azurenative.mobilepacketcore.outputs.GetNssfDeploymentResult;
import com.pulumi.azurenative.mobilepacketcore.outputs.GetObservabilityServiceResult;
import com.pulumi.azurenative.mobilepacketcore.outputs.GetSmfDeploymentResult;
import com.pulumi.azurenative.mobilepacketcore.outputs.GetUpfDeploymentResult;
import com.pulumi.core.Output;
import com.pulumi.core.TypeShape;
import com.pulumi.deployment.Deployment;
import com.pulumi.deployment.InvokeOptions;
import java.util.concurrent.CompletableFuture;
public final class MobilepacketcoreFunctions {
/**
* Get a AmfDeploymentResource
* Azure REST API version: 2023-10-15-preview.
*
*/
public static Output getAmfDeployment(GetAmfDeploymentArgs args) {
return getAmfDeployment(args, InvokeOptions.Empty);
}
/**
* Get a AmfDeploymentResource
* Azure REST API version: 2023-10-15-preview.
*
*/
public static CompletableFuture getAmfDeploymentPlain(GetAmfDeploymentPlainArgs args) {
return getAmfDeploymentPlain(args, InvokeOptions.Empty);
}
/**
* Get a AmfDeploymentResource
* Azure REST API version: 2023-10-15-preview.
*
*/
public static Output getAmfDeployment(GetAmfDeploymentArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:mobilepacketcore:getAmfDeployment", TypeShape.of(GetAmfDeploymentResult.class), args, Utilities.withVersion(options));
}
/**
* Get a AmfDeploymentResource
* Azure REST API version: 2023-10-15-preview.
*
*/
public static CompletableFuture getAmfDeploymentPlain(GetAmfDeploymentPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:mobilepacketcore:getAmfDeployment", TypeShape.of(GetAmfDeploymentResult.class), args, Utilities.withVersion(options));
}
/**
* Get a ClusterServiceResource
* Azure REST API version: 2023-10-15-preview.
*
*/
public static Output getClusterService(GetClusterServiceArgs args) {
return getClusterService(args, InvokeOptions.Empty);
}
/**
* Get a ClusterServiceResource
* Azure REST API version: 2023-10-15-preview.
*
*/
public static CompletableFuture getClusterServicePlain(GetClusterServicePlainArgs args) {
return getClusterServicePlain(args, InvokeOptions.Empty);
}
/**
* Get a ClusterServiceResource
* Azure REST API version: 2023-10-15-preview.
*
*/
public static Output getClusterService(GetClusterServiceArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:mobilepacketcore:getClusterService", TypeShape.of(GetClusterServiceResult.class), args, Utilities.withVersion(options));
}
/**
* Get a ClusterServiceResource
* Azure REST API version: 2023-10-15-preview.
*
*/
public static CompletableFuture getClusterServicePlain(GetClusterServicePlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:mobilepacketcore:getClusterService", TypeShape.of(GetClusterServiceResult.class), args, Utilities.withVersion(options));
}
/**
* Get a NetworkFunctionResource
* Azure REST API version: 2023-05-15-preview.
*
*/
public static Output getNetworkFunction(GetNetworkFunctionArgs args) {
return getNetworkFunction(args, InvokeOptions.Empty);
}
/**
* Get a NetworkFunctionResource
* Azure REST API version: 2023-05-15-preview.
*
*/
public static CompletableFuture getNetworkFunctionPlain(GetNetworkFunctionPlainArgs args) {
return getNetworkFunctionPlain(args, InvokeOptions.Empty);
}
/**
* Get a NetworkFunctionResource
* Azure REST API version: 2023-05-15-preview.
*
*/
public static Output getNetworkFunction(GetNetworkFunctionArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:mobilepacketcore:getNetworkFunction", TypeShape.of(GetNetworkFunctionResult.class), args, Utilities.withVersion(options));
}
/**
* Get a NetworkFunctionResource
* Azure REST API version: 2023-05-15-preview.
*
*/
public static CompletableFuture getNetworkFunctionPlain(GetNetworkFunctionPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:mobilepacketcore:getNetworkFunction", TypeShape.of(GetNetworkFunctionResult.class), args, Utilities.withVersion(options));
}
/**
* Get a NrfDeploymentResource
* Azure REST API version: 2023-10-15-preview.
*
*/
public static Output getNrfDeployment(GetNrfDeploymentArgs args) {
return getNrfDeployment(args, InvokeOptions.Empty);
}
/**
* Get a NrfDeploymentResource
* Azure REST API version: 2023-10-15-preview.
*
*/
public static CompletableFuture getNrfDeploymentPlain(GetNrfDeploymentPlainArgs args) {
return getNrfDeploymentPlain(args, InvokeOptions.Empty);
}
/**
* Get a NrfDeploymentResource
* Azure REST API version: 2023-10-15-preview.
*
*/
public static Output getNrfDeployment(GetNrfDeploymentArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:mobilepacketcore:getNrfDeployment", TypeShape.of(GetNrfDeploymentResult.class), args, Utilities.withVersion(options));
}
/**
* Get a NrfDeploymentResource
* Azure REST API version: 2023-10-15-preview.
*
*/
public static CompletableFuture getNrfDeploymentPlain(GetNrfDeploymentPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:mobilepacketcore:getNrfDeployment", TypeShape.of(GetNrfDeploymentResult.class), args, Utilities.withVersion(options));
}
/**
* Get a NssfDeploymentResource
* Azure REST API version: 2023-10-15-preview.
*
*/
public static Output getNssfDeployment(GetNssfDeploymentArgs args) {
return getNssfDeployment(args, InvokeOptions.Empty);
}
/**
* Get a NssfDeploymentResource
* Azure REST API version: 2023-10-15-preview.
*
*/
public static CompletableFuture getNssfDeploymentPlain(GetNssfDeploymentPlainArgs args) {
return getNssfDeploymentPlain(args, InvokeOptions.Empty);
}
/**
* Get a NssfDeploymentResource
* Azure REST API version: 2023-10-15-preview.
*
*/
public static Output getNssfDeployment(GetNssfDeploymentArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:mobilepacketcore:getNssfDeployment", TypeShape.of(GetNssfDeploymentResult.class), args, Utilities.withVersion(options));
}
/**
* Get a NssfDeploymentResource
* Azure REST API version: 2023-10-15-preview.
*
*/
public static CompletableFuture getNssfDeploymentPlain(GetNssfDeploymentPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:mobilepacketcore:getNssfDeployment", TypeShape.of(GetNssfDeploymentResult.class), args, Utilities.withVersion(options));
}
/**
* Get a ObservabilityServiceResource
* Azure REST API version: 2023-10-15-preview.
*
*/
public static Output getObservabilityService(GetObservabilityServiceArgs args) {
return getObservabilityService(args, InvokeOptions.Empty);
}
/**
* Get a ObservabilityServiceResource
* Azure REST API version: 2023-10-15-preview.
*
*/
public static CompletableFuture getObservabilityServicePlain(GetObservabilityServicePlainArgs args) {
return getObservabilityServicePlain(args, InvokeOptions.Empty);
}
/**
* Get a ObservabilityServiceResource
* Azure REST API version: 2023-10-15-preview.
*
*/
public static Output getObservabilityService(GetObservabilityServiceArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:mobilepacketcore:getObservabilityService", TypeShape.of(GetObservabilityServiceResult.class), args, Utilities.withVersion(options));
}
/**
* Get a ObservabilityServiceResource
* Azure REST API version: 2023-10-15-preview.
*
*/
public static CompletableFuture getObservabilityServicePlain(GetObservabilityServicePlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:mobilepacketcore:getObservabilityService", TypeShape.of(GetObservabilityServiceResult.class), args, Utilities.withVersion(options));
}
/**
* Get a SmfDeploymentResource
* Azure REST API version: 2023-10-15-preview.
*
*/
public static Output getSmfDeployment(GetSmfDeploymentArgs args) {
return getSmfDeployment(args, InvokeOptions.Empty);
}
/**
* Get a SmfDeploymentResource
* Azure REST API version: 2023-10-15-preview.
*
*/
public static CompletableFuture getSmfDeploymentPlain(GetSmfDeploymentPlainArgs args) {
return getSmfDeploymentPlain(args, InvokeOptions.Empty);
}
/**
* Get a SmfDeploymentResource
* Azure REST API version: 2023-10-15-preview.
*
*/
public static Output getSmfDeployment(GetSmfDeploymentArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:mobilepacketcore:getSmfDeployment", TypeShape.of(GetSmfDeploymentResult.class), args, Utilities.withVersion(options));
}
/**
* Get a SmfDeploymentResource
* Azure REST API version: 2023-10-15-preview.
*
*/
public static CompletableFuture getSmfDeploymentPlain(GetSmfDeploymentPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:mobilepacketcore:getSmfDeployment", TypeShape.of(GetSmfDeploymentResult.class), args, Utilities.withVersion(options));
}
/**
* Get a UpfDeploymentResource
* Azure REST API version: 2023-10-15-preview.
*
*/
public static Output getUpfDeployment(GetUpfDeploymentArgs args) {
return getUpfDeployment(args, InvokeOptions.Empty);
}
/**
* Get a UpfDeploymentResource
* Azure REST API version: 2023-10-15-preview.
*
*/
public static CompletableFuture getUpfDeploymentPlain(GetUpfDeploymentPlainArgs args) {
return getUpfDeploymentPlain(args, InvokeOptions.Empty);
}
/**
* Get a UpfDeploymentResource
* Azure REST API version: 2023-10-15-preview.
*
*/
public static Output getUpfDeployment(GetUpfDeploymentArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:mobilepacketcore:getUpfDeployment", TypeShape.of(GetUpfDeploymentResult.class), args, Utilities.withVersion(options));
}
/**
* Get a UpfDeploymentResource
* Azure REST API version: 2023-10-15-preview.
*
*/
public static CompletableFuture getUpfDeploymentPlain(GetUpfDeploymentPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:mobilepacketcore:getUpfDeployment", TypeShape.of(GetUpfDeploymentResult.class), args, Utilities.withVersion(options));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy