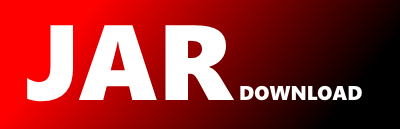
com.pulumi.azurenative.netapp.outputs.QuotaReportResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.netapp.outputs;
import com.pulumi.core.annotations.CustomType;
import java.lang.Boolean;
import java.lang.Double;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class QuotaReportResponse {
/**
* @return Flag to indicate whether the quota is derived from default quota.
*
*/
private @Nullable Boolean isDerivedQuota;
/**
* @return Percentage of used size compared to total size.
*
*/
private @Nullable Double percentageUsed;
/**
* @return Specifies the total size limit in kibibytes for the user/group quota.
*
*/
private @Nullable Double quotaLimitTotalInKiBs;
/**
* @return Specifies the current usage in kibibytes for the user/group quota.
*
*/
private @Nullable Double quotaLimitUsedInKiBs;
/**
* @return UserID/GroupID/SID based on the quota target type. UserID and groupID can be found by running ‘id’ or ‘getent’ command for the user or group and SID can be found by running <wmic useraccount where name='user-name' get sid>
*
*/
private @Nullable String quotaTarget;
/**
* @return Type of quota
*
*/
private @Nullable String quotaType;
private QuotaReportResponse() {}
/**
* @return Flag to indicate whether the quota is derived from default quota.
*
*/
public Optional isDerivedQuota() {
return Optional.ofNullable(this.isDerivedQuota);
}
/**
* @return Percentage of used size compared to total size.
*
*/
public Optional percentageUsed() {
return Optional.ofNullable(this.percentageUsed);
}
/**
* @return Specifies the total size limit in kibibytes for the user/group quota.
*
*/
public Optional quotaLimitTotalInKiBs() {
return Optional.ofNullable(this.quotaLimitTotalInKiBs);
}
/**
* @return Specifies the current usage in kibibytes for the user/group quota.
*
*/
public Optional quotaLimitUsedInKiBs() {
return Optional.ofNullable(this.quotaLimitUsedInKiBs);
}
/**
* @return UserID/GroupID/SID based on the quota target type. UserID and groupID can be found by running ‘id’ or ‘getent’ command for the user or group and SID can be found by running <wmic useraccount where name='user-name' get sid>
*
*/
public Optional quotaTarget() {
return Optional.ofNullable(this.quotaTarget);
}
/**
* @return Type of quota
*
*/
public Optional quotaType() {
return Optional.ofNullable(this.quotaType);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(QuotaReportResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable Boolean isDerivedQuota;
private @Nullable Double percentageUsed;
private @Nullable Double quotaLimitTotalInKiBs;
private @Nullable Double quotaLimitUsedInKiBs;
private @Nullable String quotaTarget;
private @Nullable String quotaType;
public Builder() {}
public Builder(QuotaReportResponse defaults) {
Objects.requireNonNull(defaults);
this.isDerivedQuota = defaults.isDerivedQuota;
this.percentageUsed = defaults.percentageUsed;
this.quotaLimitTotalInKiBs = defaults.quotaLimitTotalInKiBs;
this.quotaLimitUsedInKiBs = defaults.quotaLimitUsedInKiBs;
this.quotaTarget = defaults.quotaTarget;
this.quotaType = defaults.quotaType;
}
@CustomType.Setter
public Builder isDerivedQuota(@Nullable Boolean isDerivedQuota) {
this.isDerivedQuota = isDerivedQuota;
return this;
}
@CustomType.Setter
public Builder percentageUsed(@Nullable Double percentageUsed) {
this.percentageUsed = percentageUsed;
return this;
}
@CustomType.Setter
public Builder quotaLimitTotalInKiBs(@Nullable Double quotaLimitTotalInKiBs) {
this.quotaLimitTotalInKiBs = quotaLimitTotalInKiBs;
return this;
}
@CustomType.Setter
public Builder quotaLimitUsedInKiBs(@Nullable Double quotaLimitUsedInKiBs) {
this.quotaLimitUsedInKiBs = quotaLimitUsedInKiBs;
return this;
}
@CustomType.Setter
public Builder quotaTarget(@Nullable String quotaTarget) {
this.quotaTarget = quotaTarget;
return this;
}
@CustomType.Setter
public Builder quotaType(@Nullable String quotaType) {
this.quotaType = quotaType;
return this;
}
public QuotaReportResponse build() {
final var _resultValue = new QuotaReportResponse();
_resultValue.isDerivedQuota = isDerivedQuota;
_resultValue.percentageUsed = percentageUsed;
_resultValue.quotaLimitTotalInKiBs = quotaLimitTotalInKiBs;
_resultValue.quotaLimitUsedInKiBs = quotaLimitUsedInKiBs;
_resultValue.quotaTarget = quotaTarget;
_resultValue.quotaType = quotaType;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy