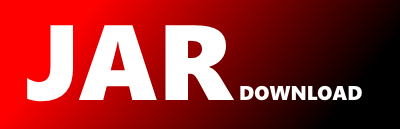
com.pulumi.azurenative.network.AzureFirewallArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.network;
import com.pulumi.azurenative.network.enums.AzureFirewallThreatIntelMode;
import com.pulumi.azurenative.network.inputs.AzureFirewallApplicationRuleCollectionArgs;
import com.pulumi.azurenative.network.inputs.AzureFirewallIPConfigurationArgs;
import com.pulumi.azurenative.network.inputs.AzureFirewallNatRuleCollectionArgs;
import com.pulumi.azurenative.network.inputs.AzureFirewallNetworkRuleCollectionArgs;
import com.pulumi.azurenative.network.inputs.AzureFirewallSkuArgs;
import com.pulumi.azurenative.network.inputs.HubIPAddressesArgs;
import com.pulumi.azurenative.network.inputs.SubResourceArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class AzureFirewallArgs extends com.pulumi.resources.ResourceArgs {
public static final AzureFirewallArgs Empty = new AzureFirewallArgs();
/**
* The additional properties used to further config this azure firewall.
*
*/
@Import(name="additionalProperties")
private @Nullable Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy