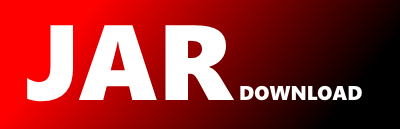
com.pulumi.azurenative.network.ConnectionMonitorArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.network;
import com.pulumi.azurenative.network.inputs.ConnectionMonitorDestinationArgs;
import com.pulumi.azurenative.network.inputs.ConnectionMonitorEndpointArgs;
import com.pulumi.azurenative.network.inputs.ConnectionMonitorOutputArgs;
import com.pulumi.azurenative.network.inputs.ConnectionMonitorSourceArgs;
import com.pulumi.azurenative.network.inputs.ConnectionMonitorTestConfigurationArgs;
import com.pulumi.azurenative.network.inputs.ConnectionMonitorTestGroupArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class ConnectionMonitorArgs extends com.pulumi.resources.ResourceArgs {
public static final ConnectionMonitorArgs Empty = new ConnectionMonitorArgs();
/**
* Determines if the connection monitor will start automatically once created.
*
*/
@Import(name="autoStart")
private @Nullable Output autoStart;
/**
* @return Determines if the connection monitor will start automatically once created.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy