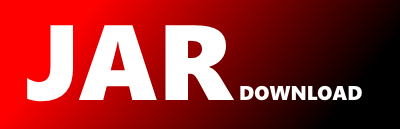
com.pulumi.azurenative.network.InboundNatRuleArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.network;
import com.pulumi.azurenative.network.enums.TransportProtocol;
import com.pulumi.azurenative.network.inputs.SubResourceArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class InboundNatRuleArgs extends com.pulumi.resources.ResourceArgs {
public static final InboundNatRuleArgs Empty = new InboundNatRuleArgs();
/**
* A reference to backendAddressPool resource.
*
*/
@Import(name="backendAddressPool")
private @Nullable Output backendAddressPool;
/**
* @return A reference to backendAddressPool resource.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy