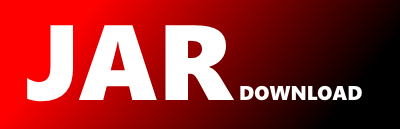
com.pulumi.azurenative.network.VpnConnection Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.network;
import com.pulumi.azurenative.Utilities;
import com.pulumi.azurenative.network.VpnConnectionArgs;
import com.pulumi.azurenative.network.outputs.IpsecPolicyResponse;
import com.pulumi.azurenative.network.outputs.RoutingConfigurationResponse;
import com.pulumi.azurenative.network.outputs.SubResourceResponse;
import com.pulumi.azurenative.network.outputs.TrafficSelectorPolicyResponse;
import com.pulumi.azurenative.network.outputs.VpnSiteLinkConnectionResponse;
import com.pulumi.core.Alias;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.Boolean;
import java.lang.Double;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* VpnConnection Resource.
* Azure REST API version: 2023-02-01. Prior API version in Azure Native 1.x: 2020-11-01.
*
* Other available API versions: 2018-04-01, 2018-07-01, 2023-04-01, 2023-05-01, 2023-06-01, 2023-09-01, 2023-11-01, 2024-01-01, 2024-03-01.
*
* ## Example Usage
* ### VpnConnectionPut
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.network.VpnConnection;
* import com.pulumi.azurenative.network.VpnConnectionArgs;
* import com.pulumi.azurenative.network.inputs.SubResourceArgs;
* import com.pulumi.azurenative.network.inputs.RoutingConfigurationArgs;
* import com.pulumi.azurenative.network.inputs.PropagatedRouteTableArgs;
* import com.pulumi.azurenative.network.inputs.VpnSiteLinkConnectionArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var vpnConnection = new VpnConnection("vpnConnection", VpnConnectionArgs.builder()
* .connectionName("vpnConnection1")
* .gatewayName("gateway1")
* .remoteVpnSite(SubResourceArgs.builder()
* .id("/subscriptions/subid/resourceGroups/rg1/providers/Microsoft.Network/vpnSites/vpnSite1")
* .build())
* .resourceGroupName("rg1")
* .routingConfiguration(RoutingConfigurationArgs.builder()
* .associatedRouteTable(SubResourceArgs.builder()
* .id("/subscriptions/subid/resourceGroups/rg1/providers/Microsoft.Network/virtualHubs/hub1/hubRouteTables/hubRouteTable1")
* .build())
* .inboundRouteMap(SubResourceArgs.builder()
* .id("/subscriptions/subid/resourceGroups/rg1/providers/Microsoft.Network/virtualHubs/virtualHub1/routeMaps/routeMap1")
* .build())
* .outboundRouteMap(SubResourceArgs.builder()
* .id("/subscriptions/subid/resourceGroups/rg1/providers/Microsoft.Network/virtualHubs/virtualHub1/routeMaps/routeMap2")
* .build())
* .propagatedRouteTables(PropagatedRouteTableArgs.builder()
* .ids(
* SubResourceArgs.builder()
* .id("/subscriptions/subid/resourceGroups/rg1/providers/Microsoft.Network/virtualHubs/hub1/hubRouteTables/hubRouteTable1")
* .build(),
* SubResourceArgs.builder()
* .id("/subscriptions/subid/resourceGroups/rg1/providers/Microsoft.Network/virtualHubs/hub1/hubRouteTables/hubRouteTable2")
* .build(),
* SubResourceArgs.builder()
* .id("/subscriptions/subid/resourceGroups/rg1/providers/Microsoft.Network/virtualHubs/hub1/hubRouteTables/hubRouteTable3")
* .build())
* .labels(
* "label1",
* "label2")
* .build())
* .build())
* .trafficSelectorPolicies()
* .vpnLinkConnections(VpnSiteLinkConnectionArgs.builder()
* .connectionBandwidth(200)
* .name("Connection-Link1")
* .sharedKey("key")
* .usePolicyBasedTrafficSelectors(false)
* .vpnConnectionProtocolType("IKEv2")
* .vpnLinkConnectionMode("Default")
* .vpnSiteLink(SubResourceArgs.builder()
* .id("/subscriptions/subid/resourceGroups/rg1/providers/Microsoft.Network/vpnSites/vpnSite1/vpnSiteLinks/siteLink1")
* .build())
* .build())
* .build());
*
* }
* }
*
* }
*
*
* ## Import
*
* An existing resource can be imported using its type token, name, and identifier, e.g.
*
* ```sh
* $ pulumi import azure-native:network:VpnConnection vpnConnection1 /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Network/vpnGateways/{gatewayName}/vpnConnections/{connectionName}
* ```
*
*/
@ResourceType(type="azure-native:network:VpnConnection")
public class VpnConnection extends com.pulumi.resources.CustomResource {
/**
* Expected bandwidth in MBPS.
*
*/
@Export(name="connectionBandwidth", refs={Integer.class}, tree="[0]")
private Output* @Nullable */ Integer> connectionBandwidth;
/**
* @return Expected bandwidth in MBPS.
*
*/
public Output> connectionBandwidth() {
return Codegen.optional(this.connectionBandwidth);
}
/**
* The connection status.
*
*/
@Export(name="connectionStatus", refs={String.class}, tree="[0]")
private Output connectionStatus;
/**
* @return The connection status.
*
*/
public Output connectionStatus() {
return this.connectionStatus;
}
/**
* DPD timeout in seconds for vpn connection.
*
*/
@Export(name="dpdTimeoutSeconds", refs={Integer.class}, tree="[0]")
private Output* @Nullable */ Integer> dpdTimeoutSeconds;
/**
* @return DPD timeout in seconds for vpn connection.
*
*/
public Output> dpdTimeoutSeconds() {
return Codegen.optional(this.dpdTimeoutSeconds);
}
/**
* Egress bytes transferred.
*
*/
@Export(name="egressBytesTransferred", refs={Double.class}, tree="[0]")
private Output egressBytesTransferred;
/**
* @return Egress bytes transferred.
*
*/
public Output egressBytesTransferred() {
return this.egressBytesTransferred;
}
/**
* EnableBgp flag.
*
*/
@Export(name="enableBgp", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> enableBgp;
/**
* @return EnableBgp flag.
*
*/
public Output> enableBgp() {
return Codegen.optional(this.enableBgp);
}
/**
* Enable internet security.
*
*/
@Export(name="enableInternetSecurity", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> enableInternetSecurity;
/**
* @return Enable internet security.
*
*/
public Output> enableInternetSecurity() {
return Codegen.optional(this.enableInternetSecurity);
}
/**
* EnableBgp flag.
*
*/
@Export(name="enableRateLimiting", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> enableRateLimiting;
/**
* @return EnableBgp flag.
*
*/
public Output> enableRateLimiting() {
return Codegen.optional(this.enableRateLimiting);
}
/**
* A unique read-only string that changes whenever the resource is updated.
*
*/
@Export(name="etag", refs={String.class}, tree="[0]")
private Output etag;
/**
* @return A unique read-only string that changes whenever the resource is updated.
*
*/
public Output etag() {
return this.etag;
}
/**
* Ingress bytes transferred.
*
*/
@Export(name="ingressBytesTransferred", refs={Double.class}, tree="[0]")
private Output ingressBytesTransferred;
/**
* @return Ingress bytes transferred.
*
*/
public Output ingressBytesTransferred() {
return this.ingressBytesTransferred;
}
/**
* The IPSec Policies to be considered by this connection.
*
*/
@Export(name="ipsecPolicies", refs={List.class,IpsecPolicyResponse.class}, tree="[0,1]")
private Output* @Nullable */ List> ipsecPolicies;
/**
* @return The IPSec Policies to be considered by this connection.
*
*/
public Output>> ipsecPolicies() {
return Codegen.optional(this.ipsecPolicies);
}
/**
* The name of the resource that is unique within a resource group. This name can be used to access the resource.
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> name;
/**
* @return The name of the resource that is unique within a resource group. This name can be used to access the resource.
*
*/
public Output> name() {
return Codegen.optional(this.name);
}
/**
* The provisioning state of the VPN connection resource.
*
*/
@Export(name="provisioningState", refs={String.class}, tree="[0]")
private Output provisioningState;
/**
* @return The provisioning state of the VPN connection resource.
*
*/
public Output provisioningState() {
return this.provisioningState;
}
/**
* Id of the connected vpn site.
*
*/
@Export(name="remoteVpnSite", refs={SubResourceResponse.class}, tree="[0]")
private Output* @Nullable */ SubResourceResponse> remoteVpnSite;
/**
* @return Id of the connected vpn site.
*
*/
public Output> remoteVpnSite() {
return Codegen.optional(this.remoteVpnSite);
}
/**
* The Routing Configuration indicating the associated and propagated route tables on this connection.
*
*/
@Export(name="routingConfiguration", refs={RoutingConfigurationResponse.class}, tree="[0]")
private Output* @Nullable */ RoutingConfigurationResponse> routingConfiguration;
/**
* @return The Routing Configuration indicating the associated and propagated route tables on this connection.
*
*/
public Output> routingConfiguration() {
return Codegen.optional(this.routingConfiguration);
}
/**
* Routing weight for vpn connection.
*
*/
@Export(name="routingWeight", refs={Integer.class}, tree="[0]")
private Output* @Nullable */ Integer> routingWeight;
/**
* @return Routing weight for vpn connection.
*
*/
public Output> routingWeight() {
return Codegen.optional(this.routingWeight);
}
/**
* SharedKey for the vpn connection.
*
*/
@Export(name="sharedKey", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> sharedKey;
/**
* @return SharedKey for the vpn connection.
*
*/
public Output> sharedKey() {
return Codegen.optional(this.sharedKey);
}
/**
* The Traffic Selector Policies to be considered by this connection.
*
*/
@Export(name="trafficSelectorPolicies", refs={List.class,TrafficSelectorPolicyResponse.class}, tree="[0,1]")
private Output* @Nullable */ List> trafficSelectorPolicies;
/**
* @return The Traffic Selector Policies to be considered by this connection.
*
*/
public Output>> trafficSelectorPolicies() {
return Codegen.optional(this.trafficSelectorPolicies);
}
/**
* Use local azure ip to initiate connection.
*
*/
@Export(name="useLocalAzureIpAddress", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> useLocalAzureIpAddress;
/**
* @return Use local azure ip to initiate connection.
*
*/
public Output> useLocalAzureIpAddress() {
return Codegen.optional(this.useLocalAzureIpAddress);
}
/**
* Enable policy-based traffic selectors.
*
*/
@Export(name="usePolicyBasedTrafficSelectors", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> usePolicyBasedTrafficSelectors;
/**
* @return Enable policy-based traffic selectors.
*
*/
public Output> usePolicyBasedTrafficSelectors() {
return Codegen.optional(this.usePolicyBasedTrafficSelectors);
}
/**
* Connection protocol used for this connection.
*
*/
@Export(name="vpnConnectionProtocolType", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> vpnConnectionProtocolType;
/**
* @return Connection protocol used for this connection.
*
*/
public Output> vpnConnectionProtocolType() {
return Codegen.optional(this.vpnConnectionProtocolType);
}
/**
* List of all vpn site link connections to the gateway.
*
*/
@Export(name="vpnLinkConnections", refs={List.class,VpnSiteLinkConnectionResponse.class}, tree="[0,1]")
private Output* @Nullable */ List> vpnLinkConnections;
/**
* @return List of all vpn site link connections to the gateway.
*
*/
public Output>> vpnLinkConnections() {
return Codegen.optional(this.vpnLinkConnections);
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public VpnConnection(java.lang.String name) {
this(name, VpnConnectionArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public VpnConnection(java.lang.String name, VpnConnectionArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public VpnConnection(java.lang.String name, VpnConnectionArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:network:VpnConnection", name, makeArgs(args, options), makeResourceOptions(options, Codegen.empty()), false);
}
private VpnConnection(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:network:VpnConnection", name, null, makeResourceOptions(options, id), false);
}
private static VpnConnectionArgs makeArgs(VpnConnectionArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
if (options != null && options.getUrn().isPresent()) {
return null;
}
return args == null ? VpnConnectionArgs.Empty : args;
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.aliases(List.of(
Output.of(Alias.builder().type("azure-native:network/v20180401:VpnConnection").build()),
Output.of(Alias.builder().type("azure-native:network/v20180601:VpnConnection").build()),
Output.of(Alias.builder().type("azure-native:network/v20180701:VpnConnection").build()),
Output.of(Alias.builder().type("azure-native:network/v20180801:VpnConnection").build()),
Output.of(Alias.builder().type("azure-native:network/v20181001:VpnConnection").build()),
Output.of(Alias.builder().type("azure-native:network/v20181101:VpnConnection").build()),
Output.of(Alias.builder().type("azure-native:network/v20181201:VpnConnection").build()),
Output.of(Alias.builder().type("azure-native:network/v20190201:VpnConnection").build()),
Output.of(Alias.builder().type("azure-native:network/v20190401:VpnConnection").build()),
Output.of(Alias.builder().type("azure-native:network/v20190601:VpnConnection").build()),
Output.of(Alias.builder().type("azure-native:network/v20190701:VpnConnection").build()),
Output.of(Alias.builder().type("azure-native:network/v20190801:VpnConnection").build()),
Output.of(Alias.builder().type("azure-native:network/v20190901:VpnConnection").build()),
Output.of(Alias.builder().type("azure-native:network/v20191101:VpnConnection").build()),
Output.of(Alias.builder().type("azure-native:network/v20191201:VpnConnection").build()),
Output.of(Alias.builder().type("azure-native:network/v20200301:VpnConnection").build()),
Output.of(Alias.builder().type("azure-native:network/v20200401:VpnConnection").build()),
Output.of(Alias.builder().type("azure-native:network/v20200501:VpnConnection").build()),
Output.of(Alias.builder().type("azure-native:network/v20200601:VpnConnection").build()),
Output.of(Alias.builder().type("azure-native:network/v20200701:VpnConnection").build()),
Output.of(Alias.builder().type("azure-native:network/v20200801:VpnConnection").build()),
Output.of(Alias.builder().type("azure-native:network/v20201101:VpnConnection").build()),
Output.of(Alias.builder().type("azure-native:network/v20210201:VpnConnection").build()),
Output.of(Alias.builder().type("azure-native:network/v20210301:VpnConnection").build()),
Output.of(Alias.builder().type("azure-native:network/v20210501:VpnConnection").build()),
Output.of(Alias.builder().type("azure-native:network/v20210801:VpnConnection").build()),
Output.of(Alias.builder().type("azure-native:network/v20220101:VpnConnection").build()),
Output.of(Alias.builder().type("azure-native:network/v20220501:VpnConnection").build()),
Output.of(Alias.builder().type("azure-native:network/v20220701:VpnConnection").build()),
Output.of(Alias.builder().type("azure-native:network/v20220901:VpnConnection").build()),
Output.of(Alias.builder().type("azure-native:network/v20221101:VpnConnection").build()),
Output.of(Alias.builder().type("azure-native:network/v20230201:VpnConnection").build()),
Output.of(Alias.builder().type("azure-native:network/v20230401:VpnConnection").build()),
Output.of(Alias.builder().type("azure-native:network/v20230501:VpnConnection").build()),
Output.of(Alias.builder().type("azure-native:network/v20230601:VpnConnection").build()),
Output.of(Alias.builder().type("azure-native:network/v20230901:VpnConnection").build()),
Output.of(Alias.builder().type("azure-native:network/v20231101:VpnConnection").build()),
Output.of(Alias.builder().type("azure-native:network/v20240101:VpnConnection").build()),
Output.of(Alias.builder().type("azure-native:network/v20240301:VpnConnection").build())
))
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static VpnConnection get(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new VpnConnection(name, id, options);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy