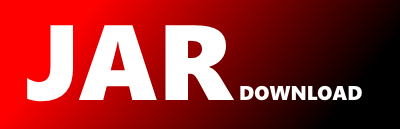
com.pulumi.azurenative.network.inputs.NatRuleArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.network.inputs;
import com.pulumi.azurenative.network.enums.FirewallPolicyRuleNetworkProtocol;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Rule of type nat.
*
*/
public final class NatRuleArgs extends com.pulumi.resources.ResourceArgs {
public static final NatRuleArgs Empty = new NatRuleArgs();
/**
* Description of the rule.
*
*/
@Import(name="description")
private @Nullable Output description;
/**
* @return Description of the rule.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy