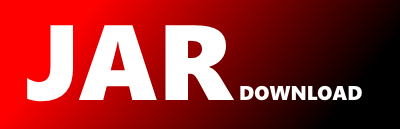
com.pulumi.azurenative.network.outputs.GetEndpointResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.network.outputs;
import com.pulumi.azurenative.network.outputs.EndpointPropertiesResponseCustomHeaders;
import com.pulumi.azurenative.network.outputs.EndpointPropertiesResponseSubnets;
import com.pulumi.core.annotations.CustomType;
import java.lang.Double;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetEndpointResult {
/**
* @return If Always Serve is enabled, probing for endpoint health will be disabled and endpoints will be included in the traffic routing method.
*
*/
private @Nullable String alwaysServe;
/**
* @return List of custom headers.
*
*/
private @Nullable List customHeaders;
/**
* @return Specifies the location of the external or nested endpoints when using the 'Performance' traffic routing method.
*
*/
private @Nullable String endpointLocation;
/**
* @return The monitoring status of the endpoint.
*
*/
private @Nullable String endpointMonitorStatus;
/**
* @return The status of the endpoint. If the endpoint is Enabled, it is probed for endpoint health and is included in the traffic routing method.
*
*/
private @Nullable String endpointStatus;
/**
* @return The list of countries/regions mapped to this endpoint when using the 'Geographic' traffic routing method. Please consult Traffic Manager Geographic documentation for a full list of accepted values.
*
*/
private @Nullable List geoMapping;
/**
* @return Fully qualified resource Id for the resource. Ex - /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Network/trafficManagerProfiles/{resourceName}
*
*/
private @Nullable String id;
/**
* @return The minimum number of endpoints that must be available in the child profile in order for the parent profile to be considered available. Only applicable to endpoint of type 'NestedEndpoints'.
*
*/
private @Nullable Double minChildEndpoints;
/**
* @return The minimum number of IPv4 (DNS record type A) endpoints that must be available in the child profile in order for the parent profile to be considered available. Only applicable to endpoint of type 'NestedEndpoints'.
*
*/
private @Nullable Double minChildEndpointsIPv4;
/**
* @return The minimum number of IPv6 (DNS record type AAAA) endpoints that must be available in the child profile in order for the parent profile to be considered available. Only applicable to endpoint of type 'NestedEndpoints'.
*
*/
private @Nullable Double minChildEndpointsIPv6;
/**
* @return The name of the resource
*
*/
private @Nullable String name;
/**
* @return The priority of this endpoint when using the 'Priority' traffic routing method. Possible values are from 1 to 1000, lower values represent higher priority. This is an optional parameter. If specified, it must be specified on all endpoints, and no two endpoints can share the same priority value.
*
*/
private @Nullable Double priority;
/**
* @return The list of subnets, IP addresses, and/or address ranges mapped to this endpoint when using the 'Subnet' traffic routing method. An empty list will match all ranges not covered by other endpoints.
*
*/
private @Nullable List subnets;
/**
* @return The fully-qualified DNS name or IP address of the endpoint. Traffic Manager returns this value in DNS responses to direct traffic to this endpoint.
*
*/
private @Nullable String target;
/**
* @return The Azure Resource URI of the of the endpoint. Not applicable to endpoints of type 'ExternalEndpoints'.
*
*/
private @Nullable String targetResourceId;
/**
* @return The type of the resource. Ex- Microsoft.Network/trafficManagerProfiles.
*
*/
private @Nullable String type;
/**
* @return The weight of this endpoint when using the 'Weighted' traffic routing method. Possible values are from 1 to 1000.
*
*/
private @Nullable Double weight;
private GetEndpointResult() {}
/**
* @return If Always Serve is enabled, probing for endpoint health will be disabled and endpoints will be included in the traffic routing method.
*
*/
public Optional alwaysServe() {
return Optional.ofNullable(this.alwaysServe);
}
/**
* @return List of custom headers.
*
*/
public List customHeaders() {
return this.customHeaders == null ? List.of() : this.customHeaders;
}
/**
* @return Specifies the location of the external or nested endpoints when using the 'Performance' traffic routing method.
*
*/
public Optional endpointLocation() {
return Optional.ofNullable(this.endpointLocation);
}
/**
* @return The monitoring status of the endpoint.
*
*/
public Optional endpointMonitorStatus() {
return Optional.ofNullable(this.endpointMonitorStatus);
}
/**
* @return The status of the endpoint. If the endpoint is Enabled, it is probed for endpoint health and is included in the traffic routing method.
*
*/
public Optional endpointStatus() {
return Optional.ofNullable(this.endpointStatus);
}
/**
* @return The list of countries/regions mapped to this endpoint when using the 'Geographic' traffic routing method. Please consult Traffic Manager Geographic documentation for a full list of accepted values.
*
*/
public List geoMapping() {
return this.geoMapping == null ? List.of() : this.geoMapping;
}
/**
* @return Fully qualified resource Id for the resource. Ex - /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Network/trafficManagerProfiles/{resourceName}
*
*/
public Optional id() {
return Optional.ofNullable(this.id);
}
/**
* @return The minimum number of endpoints that must be available in the child profile in order for the parent profile to be considered available. Only applicable to endpoint of type 'NestedEndpoints'.
*
*/
public Optional minChildEndpoints() {
return Optional.ofNullable(this.minChildEndpoints);
}
/**
* @return The minimum number of IPv4 (DNS record type A) endpoints that must be available in the child profile in order for the parent profile to be considered available. Only applicable to endpoint of type 'NestedEndpoints'.
*
*/
public Optional minChildEndpointsIPv4() {
return Optional.ofNullable(this.minChildEndpointsIPv4);
}
/**
* @return The minimum number of IPv6 (DNS record type AAAA) endpoints that must be available in the child profile in order for the parent profile to be considered available. Only applicable to endpoint of type 'NestedEndpoints'.
*
*/
public Optional minChildEndpointsIPv6() {
return Optional.ofNullable(this.minChildEndpointsIPv6);
}
/**
* @return The name of the resource
*
*/
public Optional name() {
return Optional.ofNullable(this.name);
}
/**
* @return The priority of this endpoint when using the 'Priority' traffic routing method. Possible values are from 1 to 1000, lower values represent higher priority. This is an optional parameter. If specified, it must be specified on all endpoints, and no two endpoints can share the same priority value.
*
*/
public Optional priority() {
return Optional.ofNullable(this.priority);
}
/**
* @return The list of subnets, IP addresses, and/or address ranges mapped to this endpoint when using the 'Subnet' traffic routing method. An empty list will match all ranges not covered by other endpoints.
*
*/
public List subnets() {
return this.subnets == null ? List.of() : this.subnets;
}
/**
* @return The fully-qualified DNS name or IP address of the endpoint. Traffic Manager returns this value in DNS responses to direct traffic to this endpoint.
*
*/
public Optional target() {
return Optional.ofNullable(this.target);
}
/**
* @return The Azure Resource URI of the of the endpoint. Not applicable to endpoints of type 'ExternalEndpoints'.
*
*/
public Optional targetResourceId() {
return Optional.ofNullable(this.targetResourceId);
}
/**
* @return The type of the resource. Ex- Microsoft.Network/trafficManagerProfiles.
*
*/
public Optional type() {
return Optional.ofNullable(this.type);
}
/**
* @return The weight of this endpoint when using the 'Weighted' traffic routing method. Possible values are from 1 to 1000.
*
*/
public Optional weight() {
return Optional.ofNullable(this.weight);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetEndpointResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String alwaysServe;
private @Nullable List customHeaders;
private @Nullable String endpointLocation;
private @Nullable String endpointMonitorStatus;
private @Nullable String endpointStatus;
private @Nullable List geoMapping;
private @Nullable String id;
private @Nullable Double minChildEndpoints;
private @Nullable Double minChildEndpointsIPv4;
private @Nullable Double minChildEndpointsIPv6;
private @Nullable String name;
private @Nullable Double priority;
private @Nullable List subnets;
private @Nullable String target;
private @Nullable String targetResourceId;
private @Nullable String type;
private @Nullable Double weight;
public Builder() {}
public Builder(GetEndpointResult defaults) {
Objects.requireNonNull(defaults);
this.alwaysServe = defaults.alwaysServe;
this.customHeaders = defaults.customHeaders;
this.endpointLocation = defaults.endpointLocation;
this.endpointMonitorStatus = defaults.endpointMonitorStatus;
this.endpointStatus = defaults.endpointStatus;
this.geoMapping = defaults.geoMapping;
this.id = defaults.id;
this.minChildEndpoints = defaults.minChildEndpoints;
this.minChildEndpointsIPv4 = defaults.minChildEndpointsIPv4;
this.minChildEndpointsIPv6 = defaults.minChildEndpointsIPv6;
this.name = defaults.name;
this.priority = defaults.priority;
this.subnets = defaults.subnets;
this.target = defaults.target;
this.targetResourceId = defaults.targetResourceId;
this.type = defaults.type;
this.weight = defaults.weight;
}
@CustomType.Setter
public Builder alwaysServe(@Nullable String alwaysServe) {
this.alwaysServe = alwaysServe;
return this;
}
@CustomType.Setter
public Builder customHeaders(@Nullable List customHeaders) {
this.customHeaders = customHeaders;
return this;
}
public Builder customHeaders(EndpointPropertiesResponseCustomHeaders... customHeaders) {
return customHeaders(List.of(customHeaders));
}
@CustomType.Setter
public Builder endpointLocation(@Nullable String endpointLocation) {
this.endpointLocation = endpointLocation;
return this;
}
@CustomType.Setter
public Builder endpointMonitorStatus(@Nullable String endpointMonitorStatus) {
this.endpointMonitorStatus = endpointMonitorStatus;
return this;
}
@CustomType.Setter
public Builder endpointStatus(@Nullable String endpointStatus) {
this.endpointStatus = endpointStatus;
return this;
}
@CustomType.Setter
public Builder geoMapping(@Nullable List geoMapping) {
this.geoMapping = geoMapping;
return this;
}
public Builder geoMapping(String... geoMapping) {
return geoMapping(List.of(geoMapping));
}
@CustomType.Setter
public Builder id(@Nullable String id) {
this.id = id;
return this;
}
@CustomType.Setter
public Builder minChildEndpoints(@Nullable Double minChildEndpoints) {
this.minChildEndpoints = minChildEndpoints;
return this;
}
@CustomType.Setter
public Builder minChildEndpointsIPv4(@Nullable Double minChildEndpointsIPv4) {
this.minChildEndpointsIPv4 = minChildEndpointsIPv4;
return this;
}
@CustomType.Setter
public Builder minChildEndpointsIPv6(@Nullable Double minChildEndpointsIPv6) {
this.minChildEndpointsIPv6 = minChildEndpointsIPv6;
return this;
}
@CustomType.Setter
public Builder name(@Nullable String name) {
this.name = name;
return this;
}
@CustomType.Setter
public Builder priority(@Nullable Double priority) {
this.priority = priority;
return this;
}
@CustomType.Setter
public Builder subnets(@Nullable List subnets) {
this.subnets = subnets;
return this;
}
public Builder subnets(EndpointPropertiesResponseSubnets... subnets) {
return subnets(List.of(subnets));
}
@CustomType.Setter
public Builder target(@Nullable String target) {
this.target = target;
return this;
}
@CustomType.Setter
public Builder targetResourceId(@Nullable String targetResourceId) {
this.targetResourceId = targetResourceId;
return this;
}
@CustomType.Setter
public Builder type(@Nullable String type) {
this.type = type;
return this;
}
@CustomType.Setter
public Builder weight(@Nullable Double weight) {
this.weight = weight;
return this;
}
public GetEndpointResult build() {
final var _resultValue = new GetEndpointResult();
_resultValue.alwaysServe = alwaysServe;
_resultValue.customHeaders = customHeaders;
_resultValue.endpointLocation = endpointLocation;
_resultValue.endpointMonitorStatus = endpointMonitorStatus;
_resultValue.endpointStatus = endpointStatus;
_resultValue.geoMapping = geoMapping;
_resultValue.id = id;
_resultValue.minChildEndpoints = minChildEndpoints;
_resultValue.minChildEndpointsIPv4 = minChildEndpointsIPv4;
_resultValue.minChildEndpointsIPv6 = minChildEndpointsIPv6;
_resultValue.name = name;
_resultValue.priority = priority;
_resultValue.subnets = subnets;
_resultValue.target = target;
_resultValue.targetResourceId = targetResourceId;
_resultValue.type = type;
_resultValue.weight = weight;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy