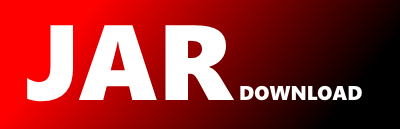
com.pulumi.azurenative.network.outputs.GetPrivateLinkServiceResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.network.outputs;
import com.pulumi.azurenative.network.outputs.ExtendedLocationResponse;
import com.pulumi.azurenative.network.outputs.FrontendIPConfigurationResponse;
import com.pulumi.azurenative.network.outputs.NetworkInterfaceResponse;
import com.pulumi.azurenative.network.outputs.PrivateEndpointConnectionResponse;
import com.pulumi.azurenative.network.outputs.PrivateLinkServiceIpConfigurationResponse;
import com.pulumi.azurenative.network.outputs.PrivateLinkServicePropertiesResponseAutoApproval;
import com.pulumi.azurenative.network.outputs.PrivateLinkServicePropertiesResponseVisibility;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetPrivateLinkServiceResult {
/**
* @return The alias of the private link service.
*
*/
private String alias;
/**
* @return The auto-approval list of the private link service.
*
*/
private @Nullable PrivateLinkServicePropertiesResponseAutoApproval autoApproval;
/**
* @return Whether the private link service is enabled for proxy protocol or not.
*
*/
private @Nullable Boolean enableProxyProtocol;
/**
* @return A unique read-only string that changes whenever the resource is updated.
*
*/
private String etag;
/**
* @return The extended location of the load balancer.
*
*/
private @Nullable ExtendedLocationResponse extendedLocation;
/**
* @return The list of Fqdn.
*
*/
private @Nullable List fqdns;
/**
* @return Resource ID.
*
*/
private @Nullable String id;
/**
* @return An array of private link service IP configurations.
*
*/
private @Nullable List ipConfigurations;
/**
* @return An array of references to the load balancer IP configurations.
*
*/
private @Nullable List loadBalancerFrontendIpConfigurations;
/**
* @return Resource location.
*
*/
private @Nullable String location;
/**
* @return Resource name.
*
*/
private String name;
/**
* @return An array of references to the network interfaces created for this private link service.
*
*/
private List networkInterfaces;
/**
* @return An array of list about connections to the private endpoint.
*
*/
private List privateEndpointConnections;
/**
* @return The provisioning state of the private link service resource.
*
*/
private String provisioningState;
/**
* @return Resource tags.
*
*/
private @Nullable Map tags;
/**
* @return Resource type.
*
*/
private String type;
/**
* @return The visibility list of the private link service.
*
*/
private @Nullable PrivateLinkServicePropertiesResponseVisibility visibility;
private GetPrivateLinkServiceResult() {}
/**
* @return The alias of the private link service.
*
*/
public String alias() {
return this.alias;
}
/**
* @return The auto-approval list of the private link service.
*
*/
public Optional autoApproval() {
return Optional.ofNullable(this.autoApproval);
}
/**
* @return Whether the private link service is enabled for proxy protocol or not.
*
*/
public Optional enableProxyProtocol() {
return Optional.ofNullable(this.enableProxyProtocol);
}
/**
* @return A unique read-only string that changes whenever the resource is updated.
*
*/
public String etag() {
return this.etag;
}
/**
* @return The extended location of the load balancer.
*
*/
public Optional extendedLocation() {
return Optional.ofNullable(this.extendedLocation);
}
/**
* @return The list of Fqdn.
*
*/
public List fqdns() {
return this.fqdns == null ? List.of() : this.fqdns;
}
/**
* @return Resource ID.
*
*/
public Optional id() {
return Optional.ofNullable(this.id);
}
/**
* @return An array of private link service IP configurations.
*
*/
public List ipConfigurations() {
return this.ipConfigurations == null ? List.of() : this.ipConfigurations;
}
/**
* @return An array of references to the load balancer IP configurations.
*
*/
public List loadBalancerFrontendIpConfigurations() {
return this.loadBalancerFrontendIpConfigurations == null ? List.of() : this.loadBalancerFrontendIpConfigurations;
}
/**
* @return Resource location.
*
*/
public Optional location() {
return Optional.ofNullable(this.location);
}
/**
* @return Resource name.
*
*/
public String name() {
return this.name;
}
/**
* @return An array of references to the network interfaces created for this private link service.
*
*/
public List networkInterfaces() {
return this.networkInterfaces;
}
/**
* @return An array of list about connections to the private endpoint.
*
*/
public List privateEndpointConnections() {
return this.privateEndpointConnections;
}
/**
* @return The provisioning state of the private link service resource.
*
*/
public String provisioningState() {
return this.provisioningState;
}
/**
* @return Resource tags.
*
*/
public Map tags() {
return this.tags == null ? Map.of() : this.tags;
}
/**
* @return Resource type.
*
*/
public String type() {
return this.type;
}
/**
* @return The visibility list of the private link service.
*
*/
public Optional visibility() {
return Optional.ofNullable(this.visibility);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetPrivateLinkServiceResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String alias;
private @Nullable PrivateLinkServicePropertiesResponseAutoApproval autoApproval;
private @Nullable Boolean enableProxyProtocol;
private String etag;
private @Nullable ExtendedLocationResponse extendedLocation;
private @Nullable List fqdns;
private @Nullable String id;
private @Nullable List ipConfigurations;
private @Nullable List loadBalancerFrontendIpConfigurations;
private @Nullable String location;
private String name;
private List networkInterfaces;
private List privateEndpointConnections;
private String provisioningState;
private @Nullable Map tags;
private String type;
private @Nullable PrivateLinkServicePropertiesResponseVisibility visibility;
public Builder() {}
public Builder(GetPrivateLinkServiceResult defaults) {
Objects.requireNonNull(defaults);
this.alias = defaults.alias;
this.autoApproval = defaults.autoApproval;
this.enableProxyProtocol = defaults.enableProxyProtocol;
this.etag = defaults.etag;
this.extendedLocation = defaults.extendedLocation;
this.fqdns = defaults.fqdns;
this.id = defaults.id;
this.ipConfigurations = defaults.ipConfigurations;
this.loadBalancerFrontendIpConfigurations = defaults.loadBalancerFrontendIpConfigurations;
this.location = defaults.location;
this.name = defaults.name;
this.networkInterfaces = defaults.networkInterfaces;
this.privateEndpointConnections = defaults.privateEndpointConnections;
this.provisioningState = defaults.provisioningState;
this.tags = defaults.tags;
this.type = defaults.type;
this.visibility = defaults.visibility;
}
@CustomType.Setter
public Builder alias(String alias) {
if (alias == null) {
throw new MissingRequiredPropertyException("GetPrivateLinkServiceResult", "alias");
}
this.alias = alias;
return this;
}
@CustomType.Setter
public Builder autoApproval(@Nullable PrivateLinkServicePropertiesResponseAutoApproval autoApproval) {
this.autoApproval = autoApproval;
return this;
}
@CustomType.Setter
public Builder enableProxyProtocol(@Nullable Boolean enableProxyProtocol) {
this.enableProxyProtocol = enableProxyProtocol;
return this;
}
@CustomType.Setter
public Builder etag(String etag) {
if (etag == null) {
throw new MissingRequiredPropertyException("GetPrivateLinkServiceResult", "etag");
}
this.etag = etag;
return this;
}
@CustomType.Setter
public Builder extendedLocation(@Nullable ExtendedLocationResponse extendedLocation) {
this.extendedLocation = extendedLocation;
return this;
}
@CustomType.Setter
public Builder fqdns(@Nullable List fqdns) {
this.fqdns = fqdns;
return this;
}
public Builder fqdns(String... fqdns) {
return fqdns(List.of(fqdns));
}
@CustomType.Setter
public Builder id(@Nullable String id) {
this.id = id;
return this;
}
@CustomType.Setter
public Builder ipConfigurations(@Nullable List ipConfigurations) {
this.ipConfigurations = ipConfigurations;
return this;
}
public Builder ipConfigurations(PrivateLinkServiceIpConfigurationResponse... ipConfigurations) {
return ipConfigurations(List.of(ipConfigurations));
}
@CustomType.Setter
public Builder loadBalancerFrontendIpConfigurations(@Nullable List loadBalancerFrontendIpConfigurations) {
this.loadBalancerFrontendIpConfigurations = loadBalancerFrontendIpConfigurations;
return this;
}
public Builder loadBalancerFrontendIpConfigurations(FrontendIPConfigurationResponse... loadBalancerFrontendIpConfigurations) {
return loadBalancerFrontendIpConfigurations(List.of(loadBalancerFrontendIpConfigurations));
}
@CustomType.Setter
public Builder location(@Nullable String location) {
this.location = location;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetPrivateLinkServiceResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder networkInterfaces(List networkInterfaces) {
if (networkInterfaces == null) {
throw new MissingRequiredPropertyException("GetPrivateLinkServiceResult", "networkInterfaces");
}
this.networkInterfaces = networkInterfaces;
return this;
}
public Builder networkInterfaces(NetworkInterfaceResponse... networkInterfaces) {
return networkInterfaces(List.of(networkInterfaces));
}
@CustomType.Setter
public Builder privateEndpointConnections(List privateEndpointConnections) {
if (privateEndpointConnections == null) {
throw new MissingRequiredPropertyException("GetPrivateLinkServiceResult", "privateEndpointConnections");
}
this.privateEndpointConnections = privateEndpointConnections;
return this;
}
public Builder privateEndpointConnections(PrivateEndpointConnectionResponse... privateEndpointConnections) {
return privateEndpointConnections(List.of(privateEndpointConnections));
}
@CustomType.Setter
public Builder provisioningState(String provisioningState) {
if (provisioningState == null) {
throw new MissingRequiredPropertyException("GetPrivateLinkServiceResult", "provisioningState");
}
this.provisioningState = provisioningState;
return this;
}
@CustomType.Setter
public Builder tags(@Nullable Map tags) {
this.tags = tags;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetPrivateLinkServiceResult", "type");
}
this.type = type;
return this;
}
@CustomType.Setter
public Builder visibility(@Nullable PrivateLinkServicePropertiesResponseVisibility visibility) {
this.visibility = visibility;
return this;
}
public GetPrivateLinkServiceResult build() {
final var _resultValue = new GetPrivateLinkServiceResult();
_resultValue.alias = alias;
_resultValue.autoApproval = autoApproval;
_resultValue.enableProxyProtocol = enableProxyProtocol;
_resultValue.etag = etag;
_resultValue.extendedLocation = extendedLocation;
_resultValue.fqdns = fqdns;
_resultValue.id = id;
_resultValue.ipConfigurations = ipConfigurations;
_resultValue.loadBalancerFrontendIpConfigurations = loadBalancerFrontendIpConfigurations;
_resultValue.location = location;
_resultValue.name = name;
_resultValue.networkInterfaces = networkInterfaces;
_resultValue.privateEndpointConnections = privateEndpointConnections;
_resultValue.provisioningState = provisioningState;
_resultValue.tags = tags;
_resultValue.type = type;
_resultValue.visibility = visibility;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy