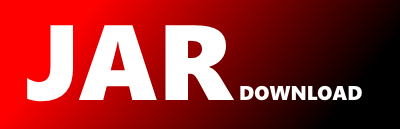
com.pulumi.azurenative.network.outputs.IPTrafficResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.network.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Objects;
@CustomType
public final class IPTrafficResponse {
/**
* @return List of destination IP addresses of the traffic..
*
*/
private List destinationIps;
/**
* @return The destination ports of the traffic.
*
*/
private List destinationPorts;
private List protocols;
/**
* @return List of source IP addresses of the traffic..
*
*/
private List sourceIps;
/**
* @return The source ports of the traffic.
*
*/
private List sourcePorts;
private IPTrafficResponse() {}
/**
* @return List of destination IP addresses of the traffic..
*
*/
public List destinationIps() {
return this.destinationIps;
}
/**
* @return The destination ports of the traffic.
*
*/
public List destinationPorts() {
return this.destinationPorts;
}
public List protocols() {
return this.protocols;
}
/**
* @return List of source IP addresses of the traffic..
*
*/
public List sourceIps() {
return this.sourceIps;
}
/**
* @return The source ports of the traffic.
*
*/
public List sourcePorts() {
return this.sourcePorts;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(IPTrafficResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private List destinationIps;
private List destinationPorts;
private List protocols;
private List sourceIps;
private List sourcePorts;
public Builder() {}
public Builder(IPTrafficResponse defaults) {
Objects.requireNonNull(defaults);
this.destinationIps = defaults.destinationIps;
this.destinationPorts = defaults.destinationPorts;
this.protocols = defaults.protocols;
this.sourceIps = defaults.sourceIps;
this.sourcePorts = defaults.sourcePorts;
}
@CustomType.Setter
public Builder destinationIps(List destinationIps) {
if (destinationIps == null) {
throw new MissingRequiredPropertyException("IPTrafficResponse", "destinationIps");
}
this.destinationIps = destinationIps;
return this;
}
public Builder destinationIps(String... destinationIps) {
return destinationIps(List.of(destinationIps));
}
@CustomType.Setter
public Builder destinationPorts(List destinationPorts) {
if (destinationPorts == null) {
throw new MissingRequiredPropertyException("IPTrafficResponse", "destinationPorts");
}
this.destinationPorts = destinationPorts;
return this;
}
public Builder destinationPorts(String... destinationPorts) {
return destinationPorts(List.of(destinationPorts));
}
@CustomType.Setter
public Builder protocols(List protocols) {
if (protocols == null) {
throw new MissingRequiredPropertyException("IPTrafficResponse", "protocols");
}
this.protocols = protocols;
return this;
}
public Builder protocols(String... protocols) {
return protocols(List.of(protocols));
}
@CustomType.Setter
public Builder sourceIps(List sourceIps) {
if (sourceIps == null) {
throw new MissingRequiredPropertyException("IPTrafficResponse", "sourceIps");
}
this.sourceIps = sourceIps;
return this;
}
public Builder sourceIps(String... sourceIps) {
return sourceIps(List.of(sourceIps));
}
@CustomType.Setter
public Builder sourcePorts(List sourcePorts) {
if (sourcePorts == null) {
throw new MissingRequiredPropertyException("IPTrafficResponse", "sourcePorts");
}
this.sourcePorts = sourcePorts;
return this;
}
public Builder sourcePorts(String... sourcePorts) {
return sourcePorts(List.of(sourcePorts));
}
public IPTrafficResponse build() {
final var _resultValue = new IPTrafficResponse();
_resultValue.destinationIps = destinationIps;
_resultValue.destinationPorts = destinationPorts;
_resultValue.protocols = protocols;
_resultValue.sourceIps = sourceIps;
_resultValue.sourcePorts = sourcePorts;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy