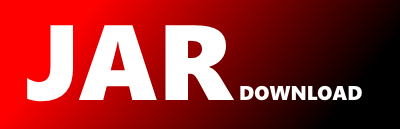
com.pulumi.azurenative.network.outputs.MonitorConfigResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.network.outputs;
import com.pulumi.azurenative.network.outputs.MonitorConfigResponseCustomHeaders;
import com.pulumi.azurenative.network.outputs.MonitorConfigResponseExpectedStatusCodeRanges;
import com.pulumi.core.annotations.CustomType;
import java.lang.Double;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class MonitorConfigResponse {
/**
* @return List of custom headers.
*
*/
private @Nullable List customHeaders;
/**
* @return List of expected status code ranges.
*
*/
private @Nullable List expectedStatusCodeRanges;
/**
* @return The monitor interval for endpoints in this profile. This is the interval at which Traffic Manager will check the health of each endpoint in this profile.
*
*/
private @Nullable Double intervalInSeconds;
/**
* @return The path relative to the endpoint domain name used to probe for endpoint health.
*
*/
private @Nullable String path;
/**
* @return The TCP port used to probe for endpoint health.
*
*/
private @Nullable Double port;
/**
* @return The profile-level monitoring status of the Traffic Manager profile.
*
*/
private @Nullable String profileMonitorStatus;
/**
* @return The protocol (HTTP, HTTPS or TCP) used to probe for endpoint health.
*
*/
private @Nullable String protocol;
/**
* @return The monitor timeout for endpoints in this profile. This is the time that Traffic Manager allows endpoints in this profile to response to the health check.
*
*/
private @Nullable Double timeoutInSeconds;
/**
* @return The number of consecutive failed health check that Traffic Manager tolerates before declaring an endpoint in this profile Degraded after the next failed health check.
*
*/
private @Nullable Double toleratedNumberOfFailures;
private MonitorConfigResponse() {}
/**
* @return List of custom headers.
*
*/
public List customHeaders() {
return this.customHeaders == null ? List.of() : this.customHeaders;
}
/**
* @return List of expected status code ranges.
*
*/
public List expectedStatusCodeRanges() {
return this.expectedStatusCodeRanges == null ? List.of() : this.expectedStatusCodeRanges;
}
/**
* @return The monitor interval for endpoints in this profile. This is the interval at which Traffic Manager will check the health of each endpoint in this profile.
*
*/
public Optional intervalInSeconds() {
return Optional.ofNullable(this.intervalInSeconds);
}
/**
* @return The path relative to the endpoint domain name used to probe for endpoint health.
*
*/
public Optional path() {
return Optional.ofNullable(this.path);
}
/**
* @return The TCP port used to probe for endpoint health.
*
*/
public Optional port() {
return Optional.ofNullable(this.port);
}
/**
* @return The profile-level monitoring status of the Traffic Manager profile.
*
*/
public Optional profileMonitorStatus() {
return Optional.ofNullable(this.profileMonitorStatus);
}
/**
* @return The protocol (HTTP, HTTPS or TCP) used to probe for endpoint health.
*
*/
public Optional protocol() {
return Optional.ofNullable(this.protocol);
}
/**
* @return The monitor timeout for endpoints in this profile. This is the time that Traffic Manager allows endpoints in this profile to response to the health check.
*
*/
public Optional timeoutInSeconds() {
return Optional.ofNullable(this.timeoutInSeconds);
}
/**
* @return The number of consecutive failed health check that Traffic Manager tolerates before declaring an endpoint in this profile Degraded after the next failed health check.
*
*/
public Optional toleratedNumberOfFailures() {
return Optional.ofNullable(this.toleratedNumberOfFailures);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(MonitorConfigResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable List customHeaders;
private @Nullable List expectedStatusCodeRanges;
private @Nullable Double intervalInSeconds;
private @Nullable String path;
private @Nullable Double port;
private @Nullable String profileMonitorStatus;
private @Nullable String protocol;
private @Nullable Double timeoutInSeconds;
private @Nullable Double toleratedNumberOfFailures;
public Builder() {}
public Builder(MonitorConfigResponse defaults) {
Objects.requireNonNull(defaults);
this.customHeaders = defaults.customHeaders;
this.expectedStatusCodeRanges = defaults.expectedStatusCodeRanges;
this.intervalInSeconds = defaults.intervalInSeconds;
this.path = defaults.path;
this.port = defaults.port;
this.profileMonitorStatus = defaults.profileMonitorStatus;
this.protocol = defaults.protocol;
this.timeoutInSeconds = defaults.timeoutInSeconds;
this.toleratedNumberOfFailures = defaults.toleratedNumberOfFailures;
}
@CustomType.Setter
public Builder customHeaders(@Nullable List customHeaders) {
this.customHeaders = customHeaders;
return this;
}
public Builder customHeaders(MonitorConfigResponseCustomHeaders... customHeaders) {
return customHeaders(List.of(customHeaders));
}
@CustomType.Setter
public Builder expectedStatusCodeRanges(@Nullable List expectedStatusCodeRanges) {
this.expectedStatusCodeRanges = expectedStatusCodeRanges;
return this;
}
public Builder expectedStatusCodeRanges(MonitorConfigResponseExpectedStatusCodeRanges... expectedStatusCodeRanges) {
return expectedStatusCodeRanges(List.of(expectedStatusCodeRanges));
}
@CustomType.Setter
public Builder intervalInSeconds(@Nullable Double intervalInSeconds) {
this.intervalInSeconds = intervalInSeconds;
return this;
}
@CustomType.Setter
public Builder path(@Nullable String path) {
this.path = path;
return this;
}
@CustomType.Setter
public Builder port(@Nullable Double port) {
this.port = port;
return this;
}
@CustomType.Setter
public Builder profileMonitorStatus(@Nullable String profileMonitorStatus) {
this.profileMonitorStatus = profileMonitorStatus;
return this;
}
@CustomType.Setter
public Builder protocol(@Nullable String protocol) {
this.protocol = protocol;
return this;
}
@CustomType.Setter
public Builder timeoutInSeconds(@Nullable Double timeoutInSeconds) {
this.timeoutInSeconds = timeoutInSeconds;
return this;
}
@CustomType.Setter
public Builder toleratedNumberOfFailures(@Nullable Double toleratedNumberOfFailures) {
this.toleratedNumberOfFailures = toleratedNumberOfFailures;
return this;
}
public MonitorConfigResponse build() {
final var _resultValue = new MonitorConfigResponse();
_resultValue.customHeaders = customHeaders;
_resultValue.expectedStatusCodeRanges = expectedStatusCodeRanges;
_resultValue.intervalInSeconds = intervalInSeconds;
_resultValue.path = path;
_resultValue.port = port;
_resultValue.profileMonitorStatus = profileMonitorStatus;
_resultValue.protocol = protocol;
_resultValue.timeoutInSeconds = timeoutInSeconds;
_resultValue.toleratedNumberOfFailures = toleratedNumberOfFailures;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy