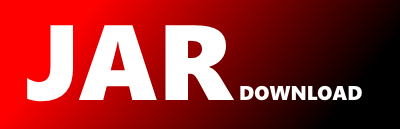
com.pulumi.azurenative.policyinsights.RemediationAtManagementGroupArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.policyinsights;
import com.pulumi.azurenative.policyinsights.enums.ResourceDiscoveryMode;
import com.pulumi.azurenative.policyinsights.inputs.RemediationFiltersArgs;
import com.pulumi.azurenative.policyinsights.inputs.RemediationPropertiesFailureThresholdArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class RemediationAtManagementGroupArgs extends com.pulumi.resources.ResourceArgs {
public static final RemediationAtManagementGroupArgs Empty = new RemediationAtManagementGroupArgs();
/**
* The remediation failure threshold settings
*
*/
@Import(name="failureThreshold")
private @Nullable Output failureThreshold;
/**
* @return The remediation failure threshold settings
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy